Fork for fixes
Embed:
(wiki syntax)
Show/hide line numbers
TcpServer.cpp
00001 /* 00002 TcpServer.cpp - Arduino implementation of a UIP wrapper class. 00003 Copyright (c) 2013 Norbert Truchsess <norbert.truchsess@t-online.de> 00004 All rights reserved. 00005 00006 This program is free software: you can redistribute it and/or modify 00007 it under the terms of the GNU General Public License as published by 00008 the Free Software Foundation, either version 3 of the License, or 00009 (at your option) any later version. 00010 00011 This program is distributed in the hope that it will be useful, 00012 but WITHOUT ANY WARRANTY; without even the implied warranty of 00013 MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00014 GNU General Public License for more details. 00015 00016 You should have received a copy of the GNU General Public License 00017 along with this program. If not, see <http://www.gnu.org/licenses/>. 00018 */ 00019 #include "UipEthernet.h" 00020 #include "TcpServer.h" 00021 extern "C" 00022 { 00023 #include "utility/uip-conf.h" 00024 } 00025 /** 00026 * @brief 00027 * @note 00028 * @param 00029 * @retval 00030 */ 00031 TcpServer::TcpServer() : 00032 _conns(1) 00033 {} 00034 00035 /** 00036 * @brief 00037 * @note 00038 * @param 00039 * @retval 00040 */ 00041 TcpClient* TcpServer::accept() 00042 { 00043 TcpClient* result = NULL; 00044 00045 UipEthernet::ethernet->tick(); 00046 for (uip_userdata_t * data = &TcpClient::all_data[0]; data < &TcpClient::all_data[_conns]; data++) { 00047 if 00048 ( 00049 data->packets_in[0] != NOBLOCK && 00050 ( 00051 ((data->state & UIP_CLIENT_CONNECTED) && uip_conns[data->state & UIP_CLIENT_SOCKETS].lport == _port) || 00052 ((data->state & UIP_CLIENT_REMOTECLOSED) && ((uip_userdata_closed_t*)data)->lport == _port) 00053 ) 00054 ) { 00055 data->ripaddr[0] = uip_conns[data->state & UIP_CLIENT_SOCKETS].ripaddr[0]; 00056 data->ripaddr[1] = uip_conns[data->state & UIP_CLIENT_SOCKETS].ripaddr[1]; 00057 result = new TcpClient(data); 00058 result->setInstance(result); 00059 return result; 00060 } 00061 } 00062 00063 return result; 00064 } 00065 00066 /** 00067 * @brief 00068 * @note 00069 * @param 00070 * @retval 00071 */ 00072 void TcpServer::open(UipEthernet* ethernet) 00073 { 00074 if (UipEthernet::ethernet != ethernet) 00075 UipEthernet::ethernet = ethernet; 00076 } 00077 00078 /** 00079 * @brief 00080 * @note 00081 * @param 00082 * @retval 00083 */ 00084 void TcpServer::bind(uint8_t port) 00085 { 00086 _port = htons(port); 00087 } 00088 00089 /** 00090 * @brief 00091 * @note 00092 * @param 00093 * @retval 00094 */ 00095 void TcpServer::bind(const char* ip, uint8_t port) 00096 { 00097 _port = htons(port); 00098 } 00099 00100 /** 00101 * @brief 00102 * @note 00103 * @param 00104 * @retval 00105 */ 00106 void TcpServer::listen(uint8_t conns) 00107 { 00108 _conns = _conns < UIP_CONNS ? conns : UIP_CONNS; 00109 uip_listen(_port); 00110 UipEthernet::ethernet->tick(); 00111 } 00112 00113 /** 00114 * @brief 00115 * @note 00116 * @param 00117 * @retval 00118 */ 00119 size_t TcpServer::send(uint8_t c) 00120 { 00121 return send(&c, 1); 00122 } 00123 00124 /** 00125 * @brief 00126 * @note 00127 * @param 00128 * @retval 00129 */ 00130 size_t TcpServer::send(const uint8_t* buf, size_t size) 00131 { 00132 size_t ret = 0; 00133 for (uip_userdata_t * data = &TcpClient::all_data[0]; data < &TcpClient::all_data[_conns]; data++) { 00134 if ((data->state & UIP_CLIENT_CONNECTED) && uip_conns[data->state & UIP_CLIENT_SOCKETS].lport == _port) 00135 ret += TcpClient::_write(data, buf, size); 00136 } 00137 00138 return ret; 00139 }
Generated on Fri Jul 15 2022 22:55:10 by
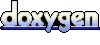