Fork for fixes
Embed:
(wiki syntax)
Show/hide line numbers
DhcpClient.h
00001 // DHCP Library v0.3 - April 25, 2009 00002 // Author: Jordan Terrell - blog.jordanterrell.com 00003 #ifndef DHCPCLIENT_h 00004 #define DHCPCLIENT_h 00005 00006 #include "UdpSocket.h" 00007 #include "IpAddress.h" 00008 00009 /* DHCP state machine. */ 00010 00011 #define STATE_DHCP_START 0 00012 #define STATE_DHCP_DISCOVER 1 00013 #define STATE_DHCP_REQUEST 2 00014 #define STATE_DHCP_LEASED 3 00015 #define STATE_DHCP_REREQUEST 4 00016 #define STATE_DHCP_RELEASE 5 00017 00018 #define DHCP_FLAGSBROADCAST 0x8000 00019 00020 /* UDP port numbers for DHCP */ 00021 00022 #define DHCP_SERVER_PORT 67 /* from server to client */ 00023 00024 #define DHCP_CLIENT_PORT 68 /* from client to server */ 00025 00026 /* DHCP message OP code */ 00027 00028 #define DHCP_BOOTREQUEST 1 00029 #define DHCP_BOOTREPLY 2 00030 00031 /* DHCP message type */ 00032 00033 #define DHCP_DISCOVER 1 00034 #define DHCP_OFFER 2 00035 #define DHCP_REQUEST 3 00036 #define DHCP_DECLINE 4 00037 #define DHCP_ACK 5 00038 #define DHCP_NAK 6 00039 #define DHCP_RELEASE 7 00040 #define DHCP_INFORM 8 00041 00042 #define DHCP_HTYPE10MB 1 00043 #define DHCP_HTYPE100MB 2 00044 00045 #define DHCP_HLENETHERNET 6 00046 #define DHCP_HOPS 0 00047 #define DHCP_SECS 0 00048 00049 #define MAGIC_COOKIE 0x63825363 00050 #define MAX_DHCP_OPT 16 00051 00052 #define HOST_NAME "H00001" 00053 #define DEFAULT_LEASE (900) //default lease time in seconds 00054 00055 #define DHCP_CHECK_NONE (0) 00056 #define DHCP_CHECK_RENEW_FAIL (1) 00057 #define DHCP_CHECK_RENEW_OK (2) 00058 #define DHCP_CHECK_REBIND_FAIL (3) 00059 #define DHCP_CHECK_REBIND_OK (4) 00060 00061 enum 00062 { 00063 padOption = 0, 00064 subnetMask = 1, 00065 timerOffset = 2, 00066 routersOnSubnet = 3, 00067 timeServer = 4, 00068 nameServer = 5, 00069 dns = 6, 00070 logServer = 7, 00071 cookieServer = 8, 00072 lprServer = 9, 00073 impressServer = 10, 00074 resourceLocationServer = 11, 00075 hostName = 12, 00076 bootFileSize = 13, 00077 meritDumpFile = 14, 00078 domainName = 15, 00079 swapServer = 16, 00080 rootPath = 17, 00081 extentionsPath = 18, 00082 IPforwarding = 19, 00083 nonLocalSourceRouting = 20, 00084 policyFilter = 21, 00085 maxDgramReasmSize = 22, 00086 defaultIPTTL = 23, 00087 pathMTUagingTimeout = 24, 00088 pathMTUplateauTable = 25, 00089 ifMTU = 26, 00090 allSubnetsLocal = 27, 00091 broadcastAddr = 28, 00092 performMaskDiscovery = 29, 00093 maskSupplier = 30, 00094 performRouterDiscovery = 31, 00095 routerSolicitationAddr = 32, 00096 staticRoute = 33, 00097 trailerEncapsulation = 34, 00098 arpCacheTimeout = 35, 00099 ethernetEncapsulation = 36, 00100 tcpDefaultTTL = 37, 00101 tcpKeepaliveInterval = 38, 00102 tcpKeepaliveGarbage = 39, 00103 nisDomainName = 40, 00104 nisServers = 41, 00105 ntpServers = 42, 00106 vendorSpecificInfo = 43, 00107 netBIOSnameServer = 44, 00108 netBIOSdgramDistServer = 45, 00109 netBIOSnodeType = 46, 00110 netBIOSscope = 47, 00111 xFontServer = 48, 00112 xDisplayManager = 49, 00113 dhcpRequestedIPaddr = 50, 00114 dhcpIPaddrLeaseTime = 51, 00115 dhcpOptionOverload = 52, 00116 dhcpMessageType = 53, 00117 dhcpServerIdentifier = 54, 00118 dhcpParamRequest = 55, 00119 dhcpMsg = 56, 00120 dhcpMaxMsgSize = 57, 00121 dhcpT1value = 58, 00122 dhcpT2value = 59, 00123 dhcpClassIdentifier = 60, 00124 dhcpClientIdentifier = 61, 00125 userMqtt = 224, 00126 endOption = 255 00127 }; 00128 00129 typedef struct _RIP_MSG_FIXED 00130 { 00131 uint8_t op; 00132 uint8_t htype; 00133 uint8_t hlen; 00134 uint8_t hops; 00135 uint32_t xid; 00136 uint16_t secs; 00137 uint16_t flags; 00138 uint8_t ciaddr[4]; 00139 uint8_t yiaddr[4]; 00140 uint8_t siaddr[4]; 00141 uint8_t giaddr[4]; 00142 uint8_t chaddr[6]; 00143 } RIP_MSG_FIXED; 00144 00145 class DhcpClient 00146 { 00147 private: 00148 uint32_t _dhcpInitialTransactionId; 00149 uint32_t _dhcpTransactionId; 00150 uint8_t _dhcpMacAddr[6]; 00151 uint8_t _dhcpLocalIp[4]; 00152 uint8_t _dhcpSubnetMask[4]; 00153 uint8_t _dhcpGatewayIp[4]; 00154 uint8_t _dhcpDhcpServerIp[4]; 00155 uint8_t _dhcpDnsServerIp[4]; 00156 uint32_t _dhcpLeaseTime; 00157 uint32_t _dhcpT1, _dhcpT2; 00158 uint8_t _dhcpUserMqttIP[4]; 00159 time_t _renewInSec; 00160 time_t _rebindInSec; 00161 time_t _lastCheck; 00162 time_t _timeout; 00163 time_t _responseTimeout; 00164 time_t _secTimeout; 00165 uint8_t _dhcp_state; 00166 UdpSocket _dhcpUdpSocket; 00167 00168 int requestDhcpLease(); 00169 void resetDhcpLease(); 00170 void presendDhcp(); 00171 void sendDhcpMessage(uint8_t, uint16_t); 00172 void printByte(char* , uint8_t); 00173 00174 uint8_t parseDhcpResponse(unsigned long responseTimeout, uint32_t& transactionId); 00175 00176 public: 00177 IpAddress getLocalIp(); 00178 IpAddress getSubnetMask(); 00179 IpAddress getGatewayIp(); 00180 IpAddress getDhcpServerIp(); 00181 IpAddress getDnsServerIp(); 00182 IpAddress getMqttServerIp(); 00183 00184 int begin(uint8_t* mac, unsigned long timeout = 60, unsigned long responseTimeout = 4); 00185 int checkLease(); 00186 }; 00187 #endif
Generated on Fri Jul 15 2022 22:55:10 by
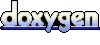