Circular Buffer C implementation, must create a buffer structure instance in your main and then dinamically allocate memory for the buffer array.
circularBuff.h
00001 #include "mbed.h" 00002 00003 #ifndef CIRCULARBUFF_H 00004 #define CIRCULARBUF_H 00005 00006 typedef struct { 00007 uint8_t *buffer; 00008 size_t head; 00009 size_t tail; 00010 size_t size; //of the buffer 00011 } circ_buf_t; 00012 00013 int circ_buf_rst(circ_buf_t * cbuf); 00014 int circ_buf_put(circ_buf_t * cbuf, uint8_t data); 00015 int circ_buf_get(circ_buf_t * cbuf, uint8_t * data); 00016 bool circ_buf_empty(circ_buf_t cbuf); 00017 bool circ_buf_full(circ_buf_t cbuf); 00018 00019 #endif
Generated on Tue Jul 19 2022 12:46:54 by
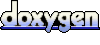