Circular Buffer C implementation, must create a buffer structure instance in your main and then dinamically allocate memory for the buffer array.
circularBuff.cpp
00001 #include "circularBuff.h" 00002 00003 int circ_buf_rst(circ_buf_t * cbuf) 00004 { 00005 int r = -1; 00006 00007 if(cbuf) 00008 { 00009 cbuf->head = 0; 00010 cbuf->tail = 0; 00011 r = 0; 00012 } 00013 00014 return r; 00015 } 00016 00017 bool circ_buf_empty(circ_buf_t cbuf) 00018 { 00019 // We define empty as head == tail 00020 return (cbuf.head == cbuf.tail); 00021 } 00022 00023 bool circ_buf_full(circ_buf_t cbuf) 00024 { 00025 // We determine "full" case by head being one position behind the tail 00026 // Note that this means we are wasting one space in the buffer! 00027 // Instead, you could have an "empty" flag and determine buffer full that way 00028 return ((cbuf.head + 1) % cbuf.size) == cbuf.tail; 00029 } 00030 00031 int circ_buf_put(circ_buf_t * cbuf, uint8_t data) 00032 { 00033 int r = -1; 00034 00035 if(cbuf) 00036 { 00037 cbuf->buffer[cbuf->head] = data; 00038 cbuf->head = (cbuf->head + 1) % cbuf->size; 00039 00040 if(cbuf->head == cbuf->tail) 00041 { 00042 cbuf->tail = (cbuf->tail + 1) % cbuf->size; 00043 } 00044 00045 r = 0; 00046 } 00047 00048 return r; 00049 } 00050 00051 int circ_buf_get(circ_buf_t * cbuf, uint8_t * data) 00052 { 00053 int r = -1; 00054 00055 if(cbuf && data && !circ_buf_empty(*cbuf)) 00056 { 00057 *data = cbuf->buffer[cbuf->tail]; 00058 cbuf->tail = (cbuf->tail + 1) % cbuf->size; 00059 00060 r = 0; 00061 } 00062 00063 return r; 00064 }
Generated on Tue Jul 19 2022 12:46:54 by
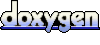