
Example program with HTTPServer and sensor data streaming over TCPSockets, using Donatien Garnier's Net APIs and services code on top of LWIP. Files StreamServer.h and .cpp encapsulate streaming over TCPSockets. Broadcast is done by sendToAll(), and all incoming data is echoed back to the client. Echo code can be replaced with some remote control of the streaming interface. See main() that shows how to periodically send some data to all subscribed clients. To subscribe, a client should open a socket at <mbed_ip> port 123. I used few lines in TCL code to set up a quick sink for the data. HTTP files are served on port 80 concurrently to the streaming.
udp.h
00001 /* 00002 * Copyright (c) 2001-2004 Swedish Institute of Computer Science. 00003 * All rights reserved. 00004 * 00005 * Redistribution and use in source and binary forms, with or without modification, 00006 * are permitted provided that the following conditions are met: 00007 * 00008 * 1. Redistributions of source code must retain the above copyright notice, 00009 * this list of conditions and the following disclaimer. 00010 * 2. Redistributions in binary form must reproduce the above copyright notice, 00011 * this list of conditions and the following disclaimer in the documentation 00012 * and/or other materials provided with the distribution. 00013 * 3. The name of the author may not be used to endorse or promote products 00014 * derived from this software without specific prior written permission. 00015 * 00016 * THIS SOFTWARE IS PROVIDED BY THE AUTHOR ``AS IS'' AND ANY EXPRESS OR IMPLIED 00017 * WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF 00018 * MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT 00019 * SHALL THE AUTHOR BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, 00020 * EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT 00021 * OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS 00022 * INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN 00023 * CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING 00024 * IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY 00025 * OF SUCH DAMAGE. 00026 * 00027 * This file is part of the lwIP TCP/IP stack. 00028 * 00029 * Author: Adam Dunkels <adam@sics.se> 00030 * 00031 */ 00032 #ifndef __LWIP_UDP_H__ 00033 #define __LWIP_UDP_H__ 00034 00035 #include "lwip/opt.h" 00036 00037 #if LWIP_UDP /* don't build if not configured for use in lwipopts.h */ 00038 00039 #include "lwip/pbuf.h" 00040 #include "lwip/netif.h" 00041 #include "lwip/ip_addr.h" 00042 #include "lwip/ip.h" 00043 00044 #ifdef __cplusplus 00045 extern "C" { 00046 #endif 00047 00048 #define UDP_HLEN 8 00049 00050 /* Fields are (of course) in network byte order. */ 00051 #ifdef PACK_STRUCT_USE_INCLUDES 00052 # include "arch/bpstruct.h" 00053 #endif 00054 PACK_STRUCT_BEGIN 00055 struct udp_hdr { 00056 PACK_STRUCT_FIELD(u16_t src); 00057 PACK_STRUCT_FIELD(u16_t dest); /* src/dest UDP ports */ 00058 PACK_STRUCT_FIELD(u16_t len); 00059 PACK_STRUCT_FIELD(u16_t chksum); 00060 } PACK_STRUCT_STRUCT; 00061 PACK_STRUCT_END 00062 #ifdef PACK_STRUCT_USE_INCLUDES 00063 # include "arch/epstruct.h" 00064 #endif 00065 00066 #define UDP_FLAGS_NOCHKSUM 0x01U 00067 #define UDP_FLAGS_UDPLITE 0x02U 00068 #define UDP_FLAGS_CONNECTED 0x04U 00069 00070 struct udp_pcb; 00071 00072 /** Function prototype for udp pcb receive callback functions 00073 * addr and port are in same byte order as in the pcb 00074 * The callback is responsible for freeing the pbuf 00075 * if it's not used any more. 00076 * 00077 * ATTENTION: Be aware that 'addr' points into the pbuf 'p' so freeing this pbuf 00078 * makes 'addr' invalid, too. 00079 * 00080 * @param arg user supplied argument (udp_pcb.recv_arg) 00081 * @param pcb the udp_pcb which received data 00082 * @param p the packet buffer that was received 00083 * @param addr the remote IP address from which the packet was received 00084 * @param port the remote port from which the packet was received 00085 */ 00086 typedef void (*udp_recv_fn)(void *arg, struct udp_pcb *pcb, struct pbuf *p, 00087 ip_addr_t *addr, u16_t port); 00088 00089 00090 struct udp_pcb { 00091 /* Common members of all PCB types */ 00092 IP_PCB; 00093 00094 /* Protocol specific PCB members */ 00095 00096 struct udp_pcb *next; 00097 00098 u8_t flags; 00099 /** ports are in host byte order */ 00100 u16_t local_port, remote_port; 00101 00102 #if LWIP_IGMP 00103 /** outgoing network interface for multicast packets */ 00104 ip_addr_t multicast_ip; 00105 #endif /* LWIP_IGMP */ 00106 00107 #if LWIP_UDPLITE 00108 /** used for UDP_LITE only */ 00109 u16_t chksum_len_rx, chksum_len_tx; 00110 #endif /* LWIP_UDPLITE */ 00111 00112 /** receive callback function */ 00113 udp_recv_fn recv; 00114 /** user-supplied argument for the recv callback */ 00115 void *recv_arg; 00116 }; 00117 /* udp_pcbs export for exernal reference (e.g. SNMP agent) */ 00118 extern struct udp_pcb *udp_pcbs; 00119 00120 /* The following functions is the application layer interface to the 00121 UDP code. */ 00122 struct udp_pcb * udp_new (void); 00123 void udp_remove (struct udp_pcb *pcb); 00124 err_t udp_bind (struct udp_pcb *pcb, ip_addr_t *ipaddr, 00125 u16_t port); 00126 err_t udp_connect (struct udp_pcb *pcb, ip_addr_t *ipaddr, 00127 u16_t port); 00128 void udp_disconnect (struct udp_pcb *pcb); 00129 void udp_recv (struct udp_pcb *pcb, udp_recv_fn recv, 00130 void *recv_arg); 00131 err_t udp_sendto_if (struct udp_pcb *pcb, struct pbuf *p, 00132 ip_addr_t *dst_ip, u16_t dst_port, 00133 struct netif *netif); 00134 err_t udp_sendto (struct udp_pcb *pcb, struct pbuf *p, 00135 ip_addr_t *dst_ip, u16_t dst_port); 00136 err_t udp_send (struct udp_pcb *pcb, struct pbuf *p); 00137 00138 #define udp_flags(pcb) ((pcb)->flags) 00139 #define udp_setflags(pcb, f) ((pcb)->flags = (f)) 00140 00141 /* The following functions are the lower layer interface to UDP. */ 00142 void udp_input (struct pbuf *p, struct netif *inp); 00143 00144 #define udp_init() /* Compatibility define, not init needed. */ 00145 00146 #if UDP_DEBUG 00147 void udp_debug_print(struct udp_hdr *udphdr); 00148 #else 00149 #define udp_debug_print(udphdr) 00150 #endif 00151 00152 #ifdef __cplusplus 00153 } 00154 #endif 00155 00156 #endif /* LWIP_UDP */ 00157 00158 #endif /* __LWIP_UDP_H__ */
Generated on Tue Jul 12 2022 21:10:26 by
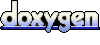