
Example program with HTTPServer and sensor data streaming over TCPSockets, using Donatien Garnier's Net APIs and services code on top of LWIP. Files StreamServer.h and .cpp encapsulate streaming over TCPSockets. Broadcast is done by sendToAll(), and all incoming data is echoed back to the client. Echo code can be replaced with some remote control of the streaming interface. See main() that shows how to periodically send some data to all subscribed clients. To subscribe, a client should open a socket at <mbed_ip> port 123. I used few lines in TCL code to set up a quick sink for the data. HTTP files are served on port 80 concurrently to the streaming.
ppp.h
00001 /***************************************************************************** 00002 * ppp.h - Network Point to Point Protocol header file. 00003 * 00004 * Copyright (c) 2003 by Marc Boucher, Services Informatiques (MBSI) inc. 00005 * portions Copyright (c) 1997 Global Election Systems Inc. 00006 * 00007 * The authors hereby grant permission to use, copy, modify, distribute, 00008 * and license this software and its documentation for any purpose, provided 00009 * that existing copyright notices are retained in all copies and that this 00010 * notice and the following disclaimer are included verbatim in any 00011 * distributions. No written agreement, license, or royalty fee is required 00012 * for any of the authorized uses. 00013 * 00014 * THIS SOFTWARE IS PROVIDED BY THE CONTRIBUTORS *AS IS* AND ANY EXPRESS OR 00015 * IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES 00016 * OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 00017 * IN NO EVENT SHALL THE CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, 00018 * INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 00019 * NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, 00020 * DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY 00021 * THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00022 * (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF 00023 * THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00024 * 00025 ****************************************************************************** 00026 * REVISION HISTORY 00027 * 00028 * 03-01-01 Marc Boucher <marc@mbsi.ca> 00029 * Ported to lwIP. 00030 * 97-11-05 Guy Lancaster <glanca@gesn.com>, Global Election Systems Inc. 00031 * Original derived from BSD codes. 00032 *****************************************************************************/ 00033 00034 #ifndef PPP_H 00035 #define PPP_H 00036 00037 #include "lwip/opt.h" 00038 00039 #if PPP_SUPPORT /* don't build if not configured for use in lwipopts.h */ 00040 00041 #include "lwip/def.h" 00042 #include "lwip/sio.h" 00043 #include "lwip/stats.h" 00044 #include "lwip/mem.h" 00045 #include "lwip/netif.h" 00046 #include "lwip/sys.h" 00047 #include "lwip/timers.h" 00048 00049 /** Some defines for code we skip compared to the original pppd. 00050 * These are just here to minimise the use of the ugly "#if 0". */ 00051 #define PPP_ADDITIONAL_CALLBACKS 0 00052 00053 /** Some error checks to test for unsupported code */ 00054 #if CBCP_SUPPORT 00055 #error "CBCP is not supported in lwIP PPP" 00056 #endif 00057 #if CCP_SUPPORT 00058 #error "CCP is not supported in lwIP PPP" 00059 #endif 00060 00061 /* 00062 * pppd.h - PPP daemon global declarations. 00063 * 00064 * Copyright (c) 1989 Carnegie Mellon University. 00065 * All rights reserved. 00066 * 00067 * Redistribution and use in source and binary forms are permitted 00068 * provided that the above copyright notice and this paragraph are 00069 * duplicated in all such forms and that any documentation, 00070 * advertising materials, and other materials related to such 00071 * distribution and use acknowledge that the software was developed 00072 * by Carnegie Mellon University. The name of the 00073 * University may not be used to endorse or promote products derived 00074 * from this software without specific prior written permission. 00075 * THIS SOFTWARE IS PROVIDED ``AS IS'' AND WITHOUT ANY EXPRESS OR 00076 * IMPLIED WARRANTIES, INCLUDING, WITHOUT LIMITATION, THE IMPLIED 00077 * WARRANTIES OF MERCHANTIBILITY AND FITNESS FOR A PARTICULAR PURPOSE. 00078 * 00079 */ 00080 /* 00081 * ppp_defs.h - PPP definitions. 00082 * 00083 * Copyright (c) 1994 The Australian National University. 00084 * All rights reserved. 00085 * 00086 * Permission to use, copy, modify, and distribute this software and its 00087 * documentation is hereby granted, provided that the above copyright 00088 * notice appears in all copies. This software is provided without any 00089 * warranty, express or implied. The Australian National University 00090 * makes no representations about the suitability of this software for 00091 * any purpose. 00092 * 00093 * IN NO EVENT SHALL THE AUSTRALIAN NATIONAL UNIVERSITY BE LIABLE TO ANY 00094 * PARTY FOR DIRECT, INDIRECT, SPECIAL, INCIDENTAL, OR CONSEQUENTIAL DAMAGES 00095 * ARISING OUT OF THE USE OF THIS SOFTWARE AND ITS DOCUMENTATION, EVEN IF 00096 * THE AUSTRALIAN NATIONAL UNIVERSITY HAVE BEEN ADVISED OF THE POSSIBILITY 00097 * OF SUCH DAMAGE. 00098 * 00099 * THE AUSTRALIAN NATIONAL UNIVERSITY SPECIFICALLY DISCLAIMS ANY WARRANTIES, 00100 * INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY 00101 * AND FITNESS FOR A PARTICULAR PURPOSE. THE SOFTWARE PROVIDED HEREUNDER IS 00102 * ON AN "AS IS" BASIS, AND THE AUSTRALIAN NATIONAL UNIVERSITY HAS NO 00103 * OBLIGATION TO PROVIDE MAINTENANCE, SUPPORT, UPDATES, ENHANCEMENTS, 00104 * OR MODIFICATIONS. 00105 */ 00106 00107 #define TIMEOUT(f, a, t) do { sys_untimeout((f), (a)); sys_timeout((t)*1000, (f), (a)); } while(0) 00108 #define UNTIMEOUT(f, a) sys_untimeout((f), (a)) 00109 00110 00111 #ifndef __u_char_defined 00112 00113 /* Type definitions for BSD code. */ 00114 typedef unsigned long u_long; 00115 typedef unsigned int u_int; 00116 typedef unsigned short u_short; 00117 typedef unsigned char u_char; 00118 00119 #endif 00120 00121 /* 00122 * Constants and structures defined by the internet system, 00123 * Per RFC 790, September 1981, and numerous additions. 00124 */ 00125 00126 /* 00127 * The basic PPP frame. 00128 */ 00129 #define PPP_HDRLEN 4 /* octets for standard ppp header */ 00130 #define PPP_FCSLEN 2 /* octets for FCS */ 00131 00132 00133 /* 00134 * Significant octet values. 00135 */ 00136 #define PPP_ALLSTATIONS 0xff /* All-Stations broadcast address */ 00137 #define PPP_UI 0x03 /* Unnumbered Information */ 00138 #define PPP_FLAG 0x7e /* Flag Sequence */ 00139 #define PPP_ESCAPE 0x7d /* Asynchronous Control Escape */ 00140 #define PPP_TRANS 0x20 /* Asynchronous transparency modifier */ 00141 00142 /* 00143 * Protocol field values. 00144 */ 00145 #define PPP_IP 0x21 /* Internet Protocol */ 00146 #define PPP_AT 0x29 /* AppleTalk Protocol */ 00147 #define PPP_VJC_COMP 0x2d /* VJ compressed TCP */ 00148 #define PPP_VJC_UNCOMP 0x2f /* VJ uncompressed TCP */ 00149 #define PPP_COMP 0xfd /* compressed packet */ 00150 #define PPP_IPCP 0x8021 /* IP Control Protocol */ 00151 #define PPP_ATCP 0x8029 /* AppleTalk Control Protocol */ 00152 #define PPP_CCP 0x80fd /* Compression Control Protocol */ 00153 #define PPP_LCP 0xc021 /* Link Control Protocol */ 00154 #define PPP_PAP 0xc023 /* Password Authentication Protocol */ 00155 #define PPP_LQR 0xc025 /* Link Quality Report protocol */ 00156 #define PPP_CHAP 0xc223 /* Cryptographic Handshake Auth. Protocol */ 00157 #define PPP_CBCP 0xc029 /* Callback Control Protocol */ 00158 00159 /* 00160 * Values for FCS calculations. 00161 */ 00162 #define PPP_INITFCS 0xffff /* Initial FCS value */ 00163 #define PPP_GOODFCS 0xf0b8 /* Good final FCS value */ 00164 #define PPP_FCS(fcs, c) (((fcs) >> 8) ^ fcstab[((fcs) ^ (c)) & 0xff]) 00165 00166 /* 00167 * Extended asyncmap - allows any character to be escaped. 00168 */ 00169 typedef u_char ext_accm[32]; 00170 00171 /* 00172 * What to do with network protocol (NP) packets. 00173 */ 00174 enum NPmode { 00175 NPMODE_PASS, /* pass the packet through */ 00176 NPMODE_DROP, /* silently drop the packet */ 00177 NPMODE_ERROR, /* return an error */ 00178 NPMODE_QUEUE /* save it up for later. */ 00179 }; 00180 00181 /* 00182 * Inline versions of get/put char/short/long. 00183 * Pointer is advanced; we assume that both arguments 00184 * are lvalues and will already be in registers. 00185 * cp MUST be u_char *. 00186 */ 00187 #define GETCHAR(c, cp) { \ 00188 (c) = *(cp)++; \ 00189 } 00190 #define PUTCHAR(c, cp) { \ 00191 *(cp)++ = (u_char) (c); \ 00192 } 00193 00194 00195 #define GETSHORT(s, cp) { \ 00196 (s) = *(cp); (cp)++; (s) <<= 8; \ 00197 (s) |= *(cp); (cp)++; \ 00198 } 00199 #define PUTSHORT(s, cp) { \ 00200 *(cp)++ = (u_char) ((s) >> 8); \ 00201 *(cp)++ = (u_char) (s & 0xff); \ 00202 } 00203 00204 #define GETLONG(l, cp) { \ 00205 (l) = *(cp); (cp)++; (l) <<= 8; \ 00206 (l) |= *(cp); (cp)++; (l) <<= 8; \ 00207 (l) |= *(cp); (cp)++; (l) <<= 8; \ 00208 (l) |= *(cp); (cp)++; \ 00209 } 00210 #define PUTLONG(l, cp) { \ 00211 *(cp)++ = (u_char) ((l) >> 24); \ 00212 *(cp)++ = (u_char) ((l) >> 16); \ 00213 *(cp)++ = (u_char) ((l) >> 8); \ 00214 *(cp)++ = (u_char) (l); \ 00215 } 00216 00217 00218 #define INCPTR(n, cp) ((cp) += (n)) 00219 #define DECPTR(n, cp) ((cp) -= (n)) 00220 00221 #define BCMP(s0, s1, l) memcmp((u_char *)(s0), (u_char *)(s1), (l)) 00222 #define BCOPY(s, d, l) MEMCPY((d), (s), (l)) 00223 #define BZERO(s, n) memset(s, 0, n) 00224 00225 #if PPP_DEBUG 00226 #define PRINTMSG(m, l) { m[l] = '\0'; LWIP_DEBUGF(LOG_INFO, ("Remote message: %s\n", m)); } 00227 #else /* PPP_DEBUG */ 00228 #define PRINTMSG(m, l) 00229 #endif /* PPP_DEBUG */ 00230 00231 /* 00232 * MAKEHEADER - Add PPP Header fields to a packet. 00233 */ 00234 #define MAKEHEADER(p, t) { \ 00235 PUTCHAR(PPP_ALLSTATIONS, p); \ 00236 PUTCHAR(PPP_UI, p); \ 00237 PUTSHORT(t, p); } 00238 00239 /************************* 00240 *** PUBLIC DEFINITIONS *** 00241 *************************/ 00242 00243 /* Error codes. */ 00244 #define PPPERR_NONE 0 /* No error. */ 00245 #define PPPERR_PARAM -1 /* Invalid parameter. */ 00246 #define PPPERR_OPEN -2 /* Unable to open PPP session. */ 00247 #define PPPERR_DEVICE -3 /* Invalid I/O device for PPP. */ 00248 #define PPPERR_ALLOC -4 /* Unable to allocate resources. */ 00249 #define PPPERR_USER -5 /* User interrupt. */ 00250 #define PPPERR_CONNECT -6 /* Connection lost. */ 00251 #define PPPERR_AUTHFAIL -7 /* Failed authentication challenge. */ 00252 #define PPPERR_PROTOCOL -8 /* Failed to meet protocol. */ 00253 00254 /* 00255 * PPP IOCTL commands. 00256 */ 00257 /* 00258 * Get the up status - 0 for down, non-zero for up. The argument must 00259 * point to an int. 00260 */ 00261 #define PPPCTLG_UPSTATUS 100 /* Get the up status - 0 down else up */ 00262 #define PPPCTLS_ERRCODE 101 /* Set the error code */ 00263 #define PPPCTLG_ERRCODE 102 /* Get the error code */ 00264 #define PPPCTLG_FD 103 /* Get the fd associated with the ppp */ 00265 00266 /************************ 00267 *** PUBLIC DATA TYPES *** 00268 ************************/ 00269 00270 /* 00271 * The following struct gives the addresses of procedures to call 00272 * for a particular protocol. 00273 */ 00274 struct protent { 00275 u_short protocol; /* PPP protocol number */ 00276 /* Initialization procedure */ 00277 void (*init) (int unit); 00278 /* Process a received packet */ 00279 void (*input) (int unit, u_char *pkt, int len); 00280 /* Process a received protocol-reject */ 00281 void (*protrej) (int unit); 00282 /* Lower layer has come up */ 00283 void (*lowerup) (int unit); 00284 /* Lower layer has gone down */ 00285 void (*lowerdown) (int unit); 00286 /* Open the protocol */ 00287 void (*open) (int unit); 00288 /* Close the protocol */ 00289 void (*close) (int unit, char *reason); 00290 #if PPP_ADDITIONAL_CALLBACKS 00291 /* Print a packet in readable form */ 00292 int (*printpkt) (u_char *pkt, int len, 00293 void (*printer) (void *, char *, ...), 00294 void *arg); 00295 /* Process a received data packet */ 00296 void (*datainput) (int unit, u_char *pkt, int len); 00297 #endif /* PPP_ADDITIONAL_CALLBACKS */ 00298 int enabled_flag; /* 0 if protocol is disabled */ 00299 char *name; /* Text name of protocol */ 00300 #if PPP_ADDITIONAL_CALLBACKS 00301 /* Check requested options, assign defaults */ 00302 void (*check_options) (u_long); 00303 /* Configure interface for demand-dial */ 00304 int (*demand_conf) (int unit); 00305 /* Say whether to bring up link for this pkt */ 00306 int (*active_pkt) (u_char *pkt, int len); 00307 #endif /* PPP_ADDITIONAL_CALLBACKS */ 00308 }; 00309 00310 /* 00311 * The following structure records the time in seconds since 00312 * the last NP packet was sent or received. 00313 */ 00314 struct ppp_idle { 00315 u_short xmit_idle; /* seconds since last NP packet sent */ 00316 u_short recv_idle; /* seconds since last NP packet received */ 00317 }; 00318 00319 struct ppp_settings { 00320 00321 u_int disable_defaultip : 1; /* Don't use hostname for default IP addrs */ 00322 u_int auth_required : 1; /* Peer is required to authenticate */ 00323 u_int explicit_remote : 1; /* remote_name specified with remotename opt */ 00324 u_int refuse_pap : 1; /* Don't wanna auth. ourselves with PAP */ 00325 u_int refuse_chap : 1; /* Don't wanna auth. ourselves with CHAP */ 00326 u_int usehostname : 1; /* Use hostname for our_name */ 00327 u_int usepeerdns : 1; /* Ask peer for DNS adds */ 00328 00329 u_short idle_time_limit; /* Shut down link if idle for this long */ 00330 int maxconnect; /* Maximum connect time (seconds) */ 00331 00332 char user [MAXNAMELEN + 1]; /* Username for PAP */ 00333 char passwd [MAXSECRETLEN + 1]; /* Password for PAP, secret for CHAP */ 00334 char our_name [MAXNAMELEN + 1]; /* Our name for authentication purposes */ 00335 char remote_name[MAXNAMELEN + 1]; /* Peer's name for authentication */ 00336 }; 00337 00338 struct ppp_addrs { 00339 ip_addr_t our_ipaddr, his_ipaddr, netmask, dns1, dns2; 00340 }; 00341 00342 /***************************** 00343 *** PUBLIC DATA STRUCTURES *** 00344 *****************************/ 00345 00346 /* Buffers for outgoing packets. */ 00347 extern u_char outpacket_buf[NUM_PPP][PPP_MRU+PPP_HDRLEN]; 00348 00349 extern struct ppp_settings ppp_settings; 00350 00351 extern struct protent *ppp_protocols[]; /* Table of pointers to supported protocols */ 00352 00353 00354 /*********************** 00355 *** PUBLIC FUNCTIONS *** 00356 ***********************/ 00357 00358 /* Initialize the PPP subsystem. */ 00359 void pppInit(void); 00360 00361 /* Warning: Using PPPAUTHTYPE_ANY might have security consequences. 00362 * RFC 1994 says: 00363 * 00364 * In practice, within or associated with each PPP server, there is a 00365 * database which associates "user" names with authentication 00366 * information ("secrets"). It is not anticipated that a particular 00367 * named user would be authenticated by multiple methods. This would 00368 * make the user vulnerable to attacks which negotiate the least secure 00369 * method from among a set (such as PAP rather than CHAP). If the same 00370 * secret was used, PAP would reveal the secret to be used later with 00371 * CHAP. 00372 * 00373 * Instead, for each user name there should be an indication of exactly 00374 * one method used to authenticate that user name. If a user needs to 00375 * make use of different authentication methods under different 00376 * circumstances, then distinct user names SHOULD be employed, each of 00377 * which identifies exactly one authentication method. 00378 * 00379 */ 00380 enum pppAuthType { 00381 PPPAUTHTYPE_NONE, 00382 PPPAUTHTYPE_ANY, 00383 PPPAUTHTYPE_PAP, 00384 PPPAUTHTYPE_CHAP 00385 }; 00386 00387 void pppSetAuth(enum pppAuthType authType, const char *user, const char *passwd); 00388 00389 /* 00390 * Open a new PPP connection using the given serial I/O device. 00391 * This initializes the PPP control block but does not 00392 * attempt to negotiate the LCP session. 00393 * Return a new PPP connection descriptor on success or 00394 * an error code (negative) on failure. 00395 */ 00396 int pppOverSerialOpen(sio_fd_t fd, void (*linkStatusCB)(void *ctx, int errCode, void *arg), void *linkStatusCtx); 00397 00398 /* 00399 * Open a new PPP Over Ethernet (PPPOE) connection. 00400 */ 00401 int pppOverEthernetOpen(struct netif *ethif, const char *service_name, const char *concentrator_name, void (*linkStatusCB)(void *ctx, int errCode, void *arg), void *linkStatusCtx); 00402 00403 /* for source code compatibility */ 00404 #define pppOpen(fd,cb,ls) pppOverSerialOpen(fd,cb,ls) 00405 00406 /* 00407 * Close a PPP connection and release the descriptor. 00408 * Any outstanding packets in the queues are dropped. 00409 * Return 0 on success, an error code on failure. 00410 */ 00411 int pppClose(int pd); 00412 00413 /* 00414 * Indicate to the PPP process that the line has disconnected. 00415 */ 00416 void pppSigHUP(int pd); 00417 00418 /* 00419 * Get and set parameters for the given connection. 00420 * Return 0 on success, an error code on failure. 00421 */ 00422 int pppIOCtl(int pd, int cmd, void *arg); 00423 00424 /* 00425 * Return the Maximum Transmission Unit for the given PPP connection. 00426 */ 00427 u_short pppMTU(int pd); 00428 00429 /* 00430 * Write n characters to a ppp link. 00431 * RETURN: >= 0 Number of characters written, -1 Failed to write to device. 00432 */ 00433 int pppWrite(int pd, const u_char *s, int n); 00434 00435 void pppInProcOverEthernet(int pd, struct pbuf *pb); 00436 00437 struct pbuf *pppSingleBuf(struct pbuf *p); 00438 00439 void pppLinkTerminated(int pd); 00440 00441 void pppLinkDown(int pd); 00442 00443 void pppos_input(int pd, u_char* data, int len); 00444 00445 /* Configure i/f transmit parameters */ 00446 void ppp_send_config (int, u16_t, u32_t, int, int); 00447 /* Set extended transmit ACCM */ 00448 void ppp_set_xaccm (int, ext_accm *); 00449 /* Configure i/f receive parameters */ 00450 void ppp_recv_config (int, int, u32_t, int, int); 00451 /* Find out how long link has been idle */ 00452 int get_idle_time (int, struct ppp_idle *); 00453 00454 /* Configure VJ TCP header compression */ 00455 int sifvjcomp (int, int, u8_t, u8_t); 00456 /* Configure i/f down (for IP) */ 00457 int sifup (int); 00458 /* Set mode for handling packets for proto */ 00459 int sifnpmode (int u, int proto, enum NPmode mode); 00460 /* Configure i/f down (for IP) */ 00461 int sifdown (int); 00462 /* Configure IP addresses for i/f */ 00463 int sifaddr (int, u32_t, u32_t, u32_t, u32_t, u32_t); 00464 /* Reset i/f IP addresses */ 00465 int cifaddr (int, u32_t, u32_t); 00466 /* Create default route through i/f */ 00467 int sifdefaultroute (int, u32_t, u32_t); 00468 /* Delete default route through i/f */ 00469 int cifdefaultroute (int, u32_t, u32_t); 00470 00471 /* Get appropriate netmask for address */ 00472 u32_t GetMask (u32_t); 00473 00474 #if LWIP_NETIF_STATUS_CALLBACK 00475 void ppp_set_netif_statuscallback(int pd, netif_status_callback_fn status_callback); 00476 #endif /* LWIP_NETIF_STATUS_CALLBACK */ 00477 #if LWIP_NETIF_LINK_CALLBACK 00478 void ppp_set_netif_linkcallback(int pd, netif_status_callback_fn link_callback); 00479 #endif /* LWIP_NETIF_LINK_CALLBACK */ 00480 00481 #endif /* PPP_SUPPORT */ 00482 00483 #endif /* PPP_H */
Generated on Tue Jul 12 2022 21:10:26 by
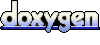