
Example program with HTTPServer and sensor data streaming over TCPSockets, using Donatien Garnier's Net APIs and services code on top of LWIP. Files StreamServer.h and .cpp encapsulate streaming over TCPSockets. Broadcast is done by sendToAll(), and all incoming data is echoed back to the client. Echo code can be replaced with some remote control of the streaming interface. See main() that shows how to periodically send some data to all subscribed clients. To subscribe, a client should open a socket at <mbed_ip> port 123. I used few lines in TCL code to set up a quick sink for the data. HTTP files are served on port 80 concurrently to the streaming.
lwipopts.h
00001 /* 00002 * Copyright (c) 2001-2003 Swedish Institute of Computer Science. 00003 * All rights reserved. 00004 * 00005 * Redistribution and use in source and binary forms, with or without modification, 00006 * are permitted provided that the following conditions are met: 00007 * 00008 * 1. Redistributions of source code must retain the above copyright notice, 00009 * this list of conditions and the following disclaimer. 00010 * 2. Redistributions in binary form must reproduce the above copyright notice, 00011 * this list of conditions and the following disclaimer in the documentation 00012 * and/or other materials provided with the distribution. 00013 * 3. The name of the author may not be used to endorse or promote products 00014 * derived from this software without specific prior written permission. 00015 * 00016 * THIS SOFTWARE IS PROVIDED BY THE AUTHOR ``AS IS'' AND ANY EXPRESS OR IMPLIED 00017 * WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF 00018 * MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT 00019 * SHALL THE AUTHOR BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, 00020 * EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT 00021 * OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS 00022 * INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN 00023 * CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING 00024 * IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY 00025 * OF SUCH DAMAGE. 00026 * 00027 * This file is part of the lwIP TCP/IP stack. 00028 * 00029 * Author: Adam Dunkels <adam@sics.se> 00030 * 00031 */ 00032 #ifndef __LWIPOPTS_H__ 00033 #define __LWIPOPTS_H__ 00034 00035 #include "netCfg.h" 00036 #if NET_LWIP_STACK 00037 00038 //#include "arch/sys_arch.h" 00039 00040 /* <sys/time.h> is included in cc.h! */ 00041 #define LWIP_TIMEVAL_PRIVATE 0 00042 00043 //#define __LWIP_DEBUG 00044 #include "dbg/dbg.h" 00045 00046 #ifdef __LWIP_DEBUG 00047 00048 #define LWIP_DEBUG 1 00049 00050 #define LWIP_DBG_MIN_LEVEL 0 00051 //#define LWIP_COMPAT_SOCKETS 1 00052 #define TAPIF_DEBUG LWIP_DBG_OFF 00053 #define TUNIF_DEBUG LWIP_DBG_OFF 00054 #define UNIXIF_DEBUG LWIP_DBG_OFF 00055 #define DELIF_DEBUG LWIP_DBG_OFF 00056 #define SIO_FIFO_DEBUG LWIP_DBG_OFF 00057 #define TCPDUMP_DEBUG LWIP_DBG_OFF 00058 00059 #define PPP_DEBUG LWIP_DBG_OFF 00060 #define MEM_DEBUG LWIP_DBG_OFF 00061 #define MEMP_DEBUG LWIP_DBG_OFF 00062 #define PBUF_DEBUG LWIP_DBG_OFF 00063 #define API_LIB_DEBUG LWIP_DBG_OFF 00064 #define API_MSG_DEBUG LWIP_DBG_OFF 00065 #define TCPIP_DEBUG LWIP_DBG_ON 00066 #define NETIF_DEBUG LWIP_DBG_OFF 00067 #define SOCKETS_DEBUG LWIP_DBG_OFF 00068 #define DEMO_DEBUG LWIP_DBG_OFF 00069 #define IP_DEBUG LWIP_DBG_OFF 00070 #define IP_REASS_DEBUG LWIP_DBG_OFF 00071 #define RAW_DEBUG LWIP_DBG_OFF 00072 #define ICMP_DEBUG LWIP_DBG_OFF 00073 #define UDP_DEBUG LWIP_DBG_OFF 00074 #define TCP_DEBUG LWIP_DBG_OFF 00075 #define TCP_INPUT_DEBUG LWIP_DBG_OFF 00076 #define TCP_OUTPUT_DEBUG LWIP_DBG_OFF 00077 #define TCP_RTO_DEBUG LWIP_DBG_OFF 00078 #define TCP_CWND_DEBUG LWIP_DBG_OFF 00079 #define TCP_WND_DEBUG LWIP_DBG_OFF 00080 #define TCP_FR_DEBUG LWIP_DBG_OFF 00081 #define TCP_QLEN_DEBUG LWIP_DBG_OFF 00082 #define TCP_RST_DEBUG LWIP_DBG_OFF 00083 #define ETHARP_DEBUG LWIP_DBG_OFF 00084 00085 #endif 00086 00087 /* 00088 extern unsigned char debug_flags; 00089 #define LWIP_DBG_TYPES_ON debug_flags 00090 */ 00091 #define NO_SYS 1 00092 #define LWIP_SOCKET (NO_SYS==0) 00093 #define LWIP_NETCONN (NO_SYS==0) 00094 00095 00096 #define IP_FRAG_USES_STATIC_BUF 0 00097 00098 00099 00100 /* ---------- Memory options ---------- */ 00101 /* MEM_ALIGNMENT: should be set to the alignment of the CPU for which 00102 lwIP is compiled. 4 byte alignment -> define MEM_ALIGNMENT to 4, 2 00103 byte alignment -> define MEM_ALIGNMENT to 2. */ 00104 /* MSVC port: intel processors don't need 4-byte alignment, 00105 but are faster that way! */ 00106 #define MEM_ALIGNMENT 4 00107 00108 /* MEM_SIZE: the size of the heap memory. If the application will send 00109 a lot of data that needs to be copied, this should be set high. */ 00110 //#define MEM_SIZE 10240 00111 #define MEM_SIZE 2000//5000 00112 00113 /// 00114 #if TARGET_LPC1768 00115 00116 #define MEM_POSITION __attribute((section("AHBSRAM0"))) 00117 00118 /* MEMP_NUM_PBUF: the number of memp struct pbufs. If the application 00119 sends a lot of data out of ROM (or other static memory), this 00120 should be set high. */ 00121 #define MEMP_NUM_PBUF 16 00122 /* MEMP_NUM_RAW_PCB: the number of UDP protocol control blocks. One 00123 per active RAW "connection". */ 00124 //#define MEMP_NUM_RAW_PCB 3 00125 /* MEMP_NUM_UDP_PCB: the number of UDP protocol control blocks. One 00126 per active UDP "connection". */ 00127 #define MEMP_NUM_UDP_PCB 4 00128 /* MEMP_NUM_TCP_PCB: the number of simulatenously active TCP 00129 connections. */ 00130 #define MEMP_NUM_TCP_PCB 8 00131 /* MEMP_NUM_TCP_PCB_LISTEN: the number of listening TCP 00132 connections. */ 00133 #define MEMP_NUM_TCP_PCB_LISTEN 2//4 00134 /* MEMP_NUM_TCP_SEG: the number of simultaneously queued TCP 00135 segments. */ 00136 #define MEMP_NUM_TCP_SEG 16 00137 /* MEMP_NUM_SYS_TIMEOUT: the number of simulateously active 00138 timeouts. */ 00139 #define MEMP_NUM_SYS_TIMEOUT 12 00140 00141 /* The following four are used only with the sequential API and can be 00142 set to 0 if the application only will use the raw API. */ 00143 /* MEMP_NUM_NETBUF: the number of struct netbufs. */ 00144 #define MEMP_NUM_NETBUF 0 00145 /* MEMP_NUM_NETCONN: the number of struct netconns. */ 00146 #define MEMP_NUM_NETCONN 0 00147 /* MEMP_NUM_TCPIP_MSG_*: the number of struct tcpip_msg, which is used 00148 for sequential API communication and incoming packets. Used in 00149 src/api/tcpip.c. */ 00150 #define MEMP_NUM_TCPIP_MSG_API 0 00151 #define MEMP_NUM_TCPIP_MSG_INPKT 0 00152 00153 /* ---------- Pbuf options ---------- */ 00154 /* PBUF_POOL_SIZE: the number of buffers in the pbuf pool. */ 00155 #define PBUF_POOL_SIZE 8//16//100 00156 00157 /* PBUF_POOL_BUFSIZE: the size of each pbuf in the pbuf pool. */ 00158 #define PBUF_POOL_BUFSIZE 128 00159 00160 /* PBUF_LINK_HLEN: the number of bytes that should be allocated for a 00161 link level header. */ 00162 //#define PBUF_LINK_HLEN 16 00163 00164 /** SYS_LIGHTWEIGHT_PROT 00165 * define SYS_LIGHTWEIGHT_PROT in lwipopts.h if you want inter-task protection 00166 * for certain critical regions during buffer allocation, deallocation and memory 00167 * allocation and deallocation. 00168 */ 00169 #define SYS_LIGHTWEIGHT_PROT 0 //No sys here 00170 00171 /* ---------- TCP options ---------- */ 00172 #define LWIP_TCP 1 00173 #define TCP_TTL 255 00174 00175 /* Controls if TCP should queue segments that arrive out of 00176 order. Define to 0 if your device is low on memory. */ 00177 #define TCP_QUEUE_OOSEQ 0 00178 00179 /* TCP Maximum segment size. */ 00180 //#define TCP_MSS 1024 00181 #define TCP_MSS 0x276//536//0x276 00182 00183 /* TCP sender buffer space (bytes). */ 00184 #define TCP_SND_BUF 2048 00185 00186 /* TCP sender buffer space (pbufs). This must be at least = 2 * 00187 TCP_SND_BUF/TCP_MSS for things to work. */ 00188 #define TCP_SND_QUEUELEN (3 * TCP_SND_BUF/TCP_MSS)//(4 * TCP_SND_BUF/TCP_MSS) 00189 00190 /* TCP writable space (bytes). This must be less than or equal 00191 to TCP_SND_BUF. It is the amount of space which must be 00192 available in the tcp snd_buf for select to return writable */ 00193 #define TCP_SNDLOWAT (TCP_SND_BUF/2) 00194 00195 /* TCP receive window. */ 00196 #define TCP_WND 1024 //8096 00197 00198 /* Maximum number of retransmissions of data segments. */ 00199 //#define TCP_MAXRTX 12 00200 00201 /* Maximum number of retransmissions of SYN segments. */ 00202 //#define TCP_SYNMAXRTX 4 00203 00204 #elif TARGET_LPC2368 00205 00206 #define MEM_POSITION 00207 00208 /* MEMP_NUM_PBUF: the number of memp struct pbufs. If the application 00209 sends a lot of data out of ROM (or other static memory), this 00210 should be set high. */ 00211 #define MEMP_NUM_PBUF 8 00212 /* MEMP_NUM_RAW_PCB: the number of UDP protocol control blocks. One 00213 per active RAW "connection". */ 00214 //#define MEMP_NUM_RAW_PCB 3 00215 /* MEMP_NUM_UDP_PCB: the number of UDP protocol control blocks. One 00216 per active UDP "connection". */ 00217 #define MEMP_NUM_UDP_PCB 2 00218 /* MEMP_NUM_TCP_PCB: the number of simulatenously active TCP 00219 connections. */ 00220 #define MEMP_NUM_TCP_PCB 2 00221 /* MEMP_NUM_TCP_PCB_LISTEN: the number of listening TCP 00222 connections. */ 00223 #define MEMP_NUM_TCP_PCB_LISTEN 1//4 00224 /* MEMP_NUM_TCP_SEG: the number of simultaneously queued TCP 00225 segments. */ 00226 #define MEMP_NUM_TCP_SEG 8 00227 /* MEMP_NUM_SYS_TIMEOUT: the number of simulateously active 00228 timeouts. */ 00229 #define MEMP_NUM_SYS_TIMEOUT 12 00230 00231 /* The following four are used only with the sequential API and can be 00232 set to 0 if the application only will use the raw API. */ 00233 /* MEMP_NUM_NETBUF: the number of struct netbufs. */ 00234 #define MEMP_NUM_NETBUF 0 00235 /* MEMP_NUM_NETCONN: the number of struct netconns. */ 00236 #define MEMP_NUM_NETCONN 0 00237 /* MEMP_NUM_TCPIP_MSG_*: the number of struct tcpip_msg, which is used 00238 for sequential API communication and incoming packets. Used in 00239 src/api/tcpip.c. */ 00240 #define MEMP_NUM_TCPIP_MSG_API 0 00241 #define MEMP_NUM_TCPIP_MSG_INPKT 0 00242 00243 /* ---------- Pbuf options ---------- */ 00244 /* PBUF_POOL_SIZE: the number of buffers in the pbuf pool. */ 00245 #define PBUF_POOL_SIZE 4//16//100 00246 00247 /* PBUF_POOL_BUFSIZE: the size of each pbuf in the pbuf pool. */ 00248 #define PBUF_POOL_BUFSIZE 128 00249 00250 /* PBUF_LINK_HLEN: the number of bytes that should be allocated for a 00251 link level header. */ 00252 //#define PBUF_LINK_HLEN 16 00253 00254 /** SYS_LIGHTWEIGHT_PROT 00255 * define SYS_LIGHTWEIGHT_PROT in lwipopts.h if you want inter-task protection 00256 * for certain critical regions during buffer allocation, deallocation and memory 00257 * allocation and deallocation. 00258 */ 00259 #define SYS_LIGHTWEIGHT_PROT 0 //No sys here 00260 00261 /* ---------- TCP options ---------- */ 00262 #define LWIP_TCP 1 00263 #define TCP_TTL 255 00264 00265 /* Controls if TCP should queue segments that arrive out of 00266 order. Define to 0 if your device is low on memory. */ 00267 #define TCP_QUEUE_OOSEQ 0 00268 00269 /* TCP Maximum segment size. */ 00270 //#define TCP_MSS 1024 00271 #define TCP_MSS 0x276//536//0x276 00272 00273 /* TCP sender buffer space (bytes). */ 00274 #define TCP_SND_BUF 1024 00275 00276 /* TCP sender buffer space (pbufs). This must be at least = 2 * 00277 TCP_SND_BUF/TCP_MSS for things to work. */ 00278 #define TCP_SND_QUEUELEN (3 * TCP_SND_BUF/TCP_MSS)//(4 * TCP_SND_BUF/TCP_MSS) 00279 00280 /* TCP writable space (bytes). This must be less than or equal 00281 to TCP_SND_BUF. It is the amount of space which must be 00282 available in the tcp snd_buf for select to return writable */ 00283 #define TCP_SNDLOWAT (TCP_SND_BUF/2) 00284 00285 /* TCP receive window. */ 00286 #define TCP_WND 1024 //8096 00287 00288 /* Maximum number of retransmissions of data segments. */ 00289 //#define TCP_MAXRTX 12 00290 00291 /* Maximum number of retransmissions of SYN segments. */ 00292 //#define TCP_SYNMAXRTX 4 00293 00294 #endif 00295 00296 /* ---------- ARP options ---------- */ 00297 #define LWIP_ARP (NET_ETH | NET_ZG2100) 00298 #define ARP_TABLE_SIZE 2//4//10 00299 #define ARP_QUEUEING 0//1 00300 #define ETHARP_TRUST_IP_MAC 1 00301 00302 /* ---------- IP options ---------- */ 00303 /* Define IP_FORWARD to 1 if you wish to have the ability to forward 00304 IP packets across network interfaces. If you are going to run lwIP 00305 on a device with only one network interface, define this to 0. */ 00306 #define IP_FORWARD 0 00307 00308 00309 /* IP reassembly and segmentation.These are orthogonal even 00310 * if they both deal with IP fragments */ 00311 /* 00312 #define IP_REASSEMBLY 1 00313 #define IP_REASS_MAX_PBUFS 10 00314 #define MEMP_NUM_REASSDATA 10 00315 #define IP_FRAG 1 00316 */ 00317 /* ---------- ICMP options ---------- */ 00318 #define ICMP_TTL 255 00319 00320 /* ---------- DHCP options ---------- */ 00321 /* Define LWIP_DHCP to 1 if you want DHCP configuration of 00322 interfaces. */ 00323 #define LWIP_DHCP (NET_ETH | NET_ZG2100) 00324 00325 /* 1 if you want to do an ARP check on the offered address 00326 (recommended if using DHCP). */ 00327 #define DHCP_DOES_ARP_CHECK (LWIP_DHCP) 00328 00329 /* ---------- AUTOIP options ------- */ 00330 #define LWIP_AUTOIP 0 00331 00332 /* ---------- SNMP options ---------- */ 00333 /** @todo SNMP is experimental for now 00334 @note UDP must be available for SNMP transport */ 00335 #ifndef LWIP_SNMP 00336 #define LWIP_SNMP 0 00337 #endif 00338 00339 00340 #ifndef SNMP_PRIVATE_MIB 00341 #define SNMP_PRIVATE_MIB 0 00342 #endif 00343 00344 00345 /* ---------- UDP options ---------- */ 00346 #define LWIP_UDP 1 00347 #define UDP_TTL 255 00348 00349 /* ---------- DNS options ---------- */ 00350 #define LWIP_DNS 1 00351 00352 /* ---------- RAW options ---------- */ 00353 #define LWIP_RAW 0 00354 #define RAW_TTL 255 00355 00356 /* ---------- Statistics options ---------- */ 00357 /* individual STATS options can be turned off by defining them to 0 00358 * (e.g #define TCP_STATS 0). All of them are turned off if LWIP_STATS 00359 * is 0 00360 * */ 00361 00362 #define LWIP_STATS 0 00363 00364 /* ---------- PPP options ---------- */ 00365 00366 #define PPP_SUPPORT NET_PPP /* Set > 0 for PPP */ 00367 00368 #if PPP_SUPPORT > 0 00369 00370 #define NUM_PPP 1 /* Max PPP sessions. */ 00371 00372 00373 /* Select modules to enable. Ideally these would be set in the makefile but 00374 * we're limited by the command line length so you need to modify the settings 00375 * in this file. 00376 */ 00377 #define PAP_SUPPORT 1 /* Set > 0 for PAP. */ 00378 #define CHAP_SUPPORT 1 /* Set > 0 for CHAP. */ 00379 #define MSCHAP_SUPPORT 0 /* Set > 0 for MSCHAP (NOT FUNCTIONAL!) */ 00380 #define CBCP_SUPPORT 0 /* Set > 0 for CBCP (NOT FUNCTIONAL!) */ 00381 #define CCP_SUPPORT 0 /* Set > 0 for CCP (NOT FUNCTIONAL!) */ 00382 #define VJ_SUPPORT 1 /* Set > 0 for VJ header compression. */ 00383 #define MD5_SUPPORT 1 /* Set > 0 for MD5 (see also CHAP) */ 00384 00385 00386 /* 00387 * Timeouts. 00388 */ 00389 #define FSM_DEFTIMEOUT 6 /* Timeout time in seconds */ 00390 #define FSM_DEFMAXTERMREQS 2 /* Maximum Terminate-Request transmissions */ 00391 #define FSM_DEFMAXCONFREQS 10 /* Maximum Configure-Request transmissions */ 00392 #define FSM_DEFMAXNAKLOOPS 5 /* Maximum number of nak loops */ 00393 00394 #define UPAP_DEFTIMEOUT 6 /* Timeout (seconds) for retransmitting req */ 00395 #define UPAP_DEFREQTIME 30 /* Time to wait for auth-req from peer */ 00396 00397 #define CHAP_DEFTIMEOUT 6 /* Timeout time in seconds */ 00398 #define CHAP_DEFTRANSMITS 10 /* max # times to send challenge */ 00399 00400 00401 /* Interval in seconds between keepalive echo requests, 0 to disable. */ 00402 #if 1 00403 #define LCP_ECHOINTERVAL 0 00404 #else 00405 00406 #define LCP_ECHOINTERVAL 10 00407 #endif 00408 00409 00410 /* Number of unanswered echo requests before failure. */ 00411 #define LCP_MAXECHOFAILS 3 00412 00413 /* Max Xmit idle time (in jiffies) before resend flag char. */ 00414 #define PPP_MAXIDLEFLAG 0//Send it every time//100 00415 00416 /* 00417 * Packet sizes 00418 * 00419 * Note - lcp shouldn't be allowed to negotiate stuff outside these 00420 * limits. See lcp.h in the pppd directory. 00421 * (XXX - these constants should simply be shared by lcp.c instead 00422 * of living in lcp.h) 00423 */ 00424 #define PPP_MTU 1500 /* Default MTU (size of Info field) */ 00425 #if 0 00426 #define PPP_MAXMTU 65535 - (PPP_HDRLEN + PPP_FCSLEN) 00427 #else 00428 00429 #define PPP_MAXMTU 1500 /* Largest MTU we allow */ 00430 #endif 00431 00432 #define PPP_MINMTU 64 00433 #define PPP_MRU 1500 /* default MRU = max length of info field */ 00434 #define PPP_MAXMRU 1500 /* Largest MRU we allow */ 00435 #define PPP_DEFMRU 296 /* Try for this */ 00436 #define PPP_MINMRU 128 /* No MRUs below this */ 00437 00438 00439 #define MAXNAMELEN 64 /* max length of hostname or name for auth */ 00440 #define MAXSECRETLEN 64 /* max length of password or secret */ 00441 00442 #endif /* PPP_SUPPORT > 0 */ 00443 00444 //C++ Compat 00445 #define try vTry 00446 00447 #endif 00448 00449 00450 #endif /* __LWIPOPTS_H__ */
Generated on Tue Jul 12 2022 21:10:25 by
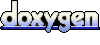