
Example program with HTTPServer and sensor data streaming over TCPSockets, using Donatien Garnier's Net APIs and services code on top of LWIP. Files StreamServer.h and .cpp encapsulate streaming over TCPSockets. Broadcast is done by sendToAll(), and all incoming data is echoed back to the client. Echo code can be replaced with some remote control of the streaming interface. See main() that shows how to periodically send some data to all subscribed clients. To subscribe, a client should open a socket at <mbed_ip> port 123. I used few lines in TCL code to set up a quick sink for the data. HTTP files are served on port 80 concurrently to the streaming.
def.c
00001 /** 00002 * @file 00003 * Common functions used throughout the stack. 00004 * 00005 */ 00006 00007 /* 00008 * Copyright (c) 2001-2004 Swedish Institute of Computer Science. 00009 * All rights reserved. 00010 * 00011 * Redistribution and use in source and binary forms, with or without modification, 00012 * are permitted provided that the following conditions are met: 00013 * 00014 * 1. Redistributions of source code must retain the above copyright notice, 00015 * this list of conditions and the following disclaimer. 00016 * 2. Redistributions in binary form must reproduce the above copyright notice, 00017 * this list of conditions and the following disclaimer in the documentation 00018 * and/or other materials provided with the distribution. 00019 * 3. The name of the author may not be used to endorse or promote products 00020 * derived from this software without specific prior written permission. 00021 * 00022 * THIS SOFTWARE IS PROVIDED BY THE AUTHOR ``AS IS'' AND ANY EXPRESS OR IMPLIED 00023 * WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF 00024 * MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT 00025 * SHALL THE AUTHOR BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, 00026 * EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT 00027 * OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS 00028 * INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN 00029 * CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING 00030 * IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY 00031 * OF SUCH DAMAGE. 00032 * 00033 * This file is part of the lwIP TCP/IP stack. 00034 * 00035 * Author: Simon Goldschmidt 00036 * 00037 */ 00038 00039 #include "lwip/opt.h" 00040 #include "lwip/def.h" 00041 00042 /** 00043 * These are reference implementations of the byte swapping functions. 00044 * Again with the aim of being simple, correct and fully portable. 00045 * Byte swapping is the second thing you would want to optimize. You will 00046 * need to port it to your architecture and in your cc.h: 00047 * 00048 * #define LWIP_PLATFORM_BYTESWAP 1 00049 * #define LWIP_PLATFORM_HTONS(x) <your_htons> 00050 * #define LWIP_PLATFORM_HTONL(x) <your_htonl> 00051 * 00052 * Note ntohs() and ntohl() are merely references to the htonx counterparts. 00053 */ 00054 00055 #if (LWIP_PLATFORM_BYTESWAP == 0) && (BYTE_ORDER == LITTLE_ENDIAN) 00056 00057 /** 00058 * Convert an u16_t from host- to network byte order. 00059 * 00060 * @param n u16_t in host byte order 00061 * @return n in network byte order 00062 */ 00063 u16_t 00064 htons(u16_t n) 00065 { 00066 return ((n & 0xff) << 8) | ((n & 0xff00) >> 8); 00067 } 00068 00069 /** 00070 * Convert an u16_t from network- to host byte order. 00071 * 00072 * @param n u16_t in network byte order 00073 * @return n in host byte order 00074 */ 00075 u16_t 00076 ntohs(u16_t n) 00077 { 00078 return htons(n); 00079 } 00080 00081 /** 00082 * Convert an u32_t from host- to network byte order. 00083 * 00084 * @param n u32_t in host byte order 00085 * @return n in network byte order 00086 */ 00087 u32_t 00088 htonl(u32_t n) 00089 { 00090 return ((n & 0xff) << 24) | 00091 ((n & 0xff00) << 8) | 00092 ((n & 0xff0000UL) >> 8) | 00093 ((n & 0xff000000UL) >> 24); 00094 } 00095 00096 /** 00097 * Convert an u32_t from network- to host byte order. 00098 * 00099 * @param n u32_t in network byte order 00100 * @return n in host byte order 00101 */ 00102 u32_t 00103 ntohl(u32_t n) 00104 { 00105 return htonl(n); 00106 } 00107 00108 #endif /* (LWIP_PLATFORM_BYTESWAP == 0) && (BYTE_ORDER == LITTLE_ENDIAN) */
Generated on Tue Jul 12 2022 21:10:25 by
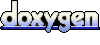