Arduino Core API Library besed on mbed platform.
Dependents: WeeESP8266 ESP8266_moj
WString.h
00001 /* 00002 WString.h - String library for Wiring & Arduino 00003 ...mostly rewritten by Paul Stoffregen... 00004 Copyright (c) 2009-10 Hernando Barragan. All right reserved. 00005 Copyright 2011, Paul Stoffregen, paul@pjrc.com 00006 00007 This library is free software; you can redistribute it and/or 00008 modify it under the terms of the GNU Lesser General Public 00009 License as published by the Free Software Foundation; either 00010 version 2.1 of the License, or (at your option) any later version. 00011 00012 This library is distributed in the hope that it will be useful, 00013 but WITHOUT ANY WARRANTY; without even the implied warranty of 00014 MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU 00015 Lesser General Public License for more details. 00016 00017 You should have received a copy of the GNU Lesser General Public 00018 License along with this library; if not, write to the Free Software 00019 Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301 USA 00020 */ 00021 00022 #ifndef __ARDUINOAPI_WSTRING_H__ 00023 #define __ARDUINOAPI_WSTRING_H__ 00024 #ifdef __cplusplus 00025 00026 #include "mbed.h" 00027 00028 #include "itoas.h" 00029 00030 #include <ctype.h> 00031 #include <cstddef> 00032 #include <cstdlib> 00033 #include <cstdio> 00034 #include <cstring> 00035 00036 00037 // An inherited class for holding the result of a concatenation. These 00038 // result objects are assumed to be writable by subsequent concatenations. 00039 class StringSumHelper; 00040 00041 // The string class 00042 class String 00043 { 00044 // use a function pointer to allow for "if (s)" without the 00045 // complications of an operator bool(). for more information, see: 00046 // http://www.artima.com/cppsource/safebool.html 00047 typedef void (String::*StringIfHelperType)() const; 00048 void StringIfHelper() const {} 00049 00050 public: 00051 // constructors 00052 // creates a copy of the initial value. 00053 // if the initial value is null or invalid, or if memory allocation 00054 // fails, the string will be marked as invalid (i.e. "if (s)" will 00055 // be false). 00056 String(const char *cstr = ""); 00057 String(const String &str); 00058 #ifdef __GXX_EXPERIMENTAL_CXX0X__ 00059 String(String &&rval); 00060 String(StringSumHelper &&rval); 00061 #endif 00062 explicit String(char c); 00063 explicit String(unsigned char, unsigned char base=10); 00064 explicit String(int, unsigned char base=10); 00065 explicit String(unsigned int, unsigned char base=10); 00066 explicit String(long, unsigned char base=10); 00067 explicit String(unsigned long, unsigned char base=10); 00068 ~String(void); 00069 00070 // memory management 00071 // return true on success, false on failure (in which case, the string 00072 // is left unchanged). reserve(0), if successful, will validate an 00073 // invalid string (i.e., "if (s)" will be true afterwards) 00074 unsigned char reserve(unsigned int size); 00075 inline unsigned int length(void) const {return len;} 00076 00077 // creates a copy of the assigned value. if the value is null or 00078 // invalid, or if the memory allocation fails, the string will be 00079 // marked as invalid ("if (s)" will be false). 00080 String & operator = (const String &rhs); 00081 String & operator = (const char *cstr); 00082 #ifdef __GXX_EXPERIMENTAL_CXX0X__ 00083 String & operator = (String &&rval); 00084 String & operator = (StringSumHelper &&rval); 00085 #endif 00086 00087 // concatenate (works w/ built-in types) 00088 00089 // returns true on success, false on failure (in which case, the string 00090 // is left unchanged). if the argument is null or invalid, the 00091 // concatenation is considered unsucessful. 00092 unsigned char concat(const String &str); 00093 unsigned char concat(const char *cstr); 00094 unsigned char concat(char c); 00095 unsigned char concat(unsigned char c); 00096 unsigned char concat(int num); 00097 unsigned char concat(unsigned int num); 00098 unsigned char concat(long num); 00099 unsigned char concat(unsigned long num); 00100 00101 // if there's not enough memory for the concatenated value, the string 00102 // will be left unchanged (but this isn't signalled in any way) 00103 String & operator += (const String &rhs) {concat(rhs); return (*this);} 00104 String & operator += (const char *cstr) {concat(cstr); return (*this);} 00105 String & operator += (char c) {concat(c); return (*this);} 00106 String & operator += (unsigned char num) {concat(num); return (*this);} 00107 String & operator += (int num) {concat(num); return (*this);} 00108 String & operator += (unsigned int num) {concat(num); return (*this);} 00109 String & operator += (long num) {concat(num); return (*this);} 00110 String & operator += (unsigned long num) {concat(num); return (*this);} 00111 00112 friend StringSumHelper & operator + (const StringSumHelper &lhs, const String &rhs); 00113 friend StringSumHelper & operator + (const StringSumHelper &lhs, const char *cstr); 00114 friend StringSumHelper & operator + (const StringSumHelper &lhs, char c); 00115 friend StringSumHelper & operator + (const StringSumHelper &lhs, unsigned char num); 00116 friend StringSumHelper & operator + (const StringSumHelper &lhs, int num); 00117 friend StringSumHelper & operator + (const StringSumHelper &lhs, unsigned int num); 00118 friend StringSumHelper & operator + (const StringSumHelper &lhs, long num); 00119 friend StringSumHelper & operator + (const StringSumHelper &lhs, unsigned long num); 00120 00121 // comparison (only works w/ Strings and "strings") 00122 operator StringIfHelperType() const { return buffer ? &String::StringIfHelper : 0; } 00123 int compareTo(const String &s) const; 00124 unsigned char equals(const String &s) const; 00125 unsigned char equals(const char *cstr) const; 00126 unsigned char operator == (const String &rhs) const {return equals(rhs);} 00127 unsigned char operator == (const char *cstr) const {return equals(cstr);} 00128 unsigned char operator != (const String &rhs) const {return !equals(rhs);} 00129 unsigned char operator != (const char *cstr) const {return !equals(cstr);} 00130 unsigned char operator < (const String &rhs) const; 00131 unsigned char operator > (const String &rhs) const; 00132 unsigned char operator <= (const String &rhs) const; 00133 unsigned char operator >= (const String &rhs) const; 00134 unsigned char equalsIgnoreCase(const String &s) const; 00135 unsigned char startsWith( const String &prefix) const; 00136 unsigned char startsWith(const String &prefix, unsigned int offset) const; 00137 unsigned char endsWith(const String &suffix) const; 00138 00139 // character acccess 00140 char charAt(unsigned int index) const; 00141 void setCharAt(unsigned int index, char c); 00142 char operator [] (unsigned int index) const; 00143 char& operator [] (unsigned int index); 00144 void getBytes(unsigned char *buf, unsigned int bufsize, unsigned int index=0) const; 00145 void toCharArray(char *buf, unsigned int bufsize, unsigned int index=0) const 00146 {getBytes((unsigned char *)buf, bufsize, index);} 00147 const char * c_str() const { return buffer; } 00148 00149 // search 00150 int indexOf( char ch ) const; 00151 int indexOf( char ch, unsigned int fromIndex ) const; 00152 int indexOf( const String &str ) const; 00153 int indexOf( const String &str, unsigned int fromIndex ) const; 00154 int lastIndexOf( char ch ) const; 00155 int lastIndexOf( char ch, unsigned int fromIndex ) const; 00156 int lastIndexOf( const String &str ) const; 00157 int lastIndexOf( const String &str, unsigned int fromIndex ) const; 00158 String substring( unsigned int beginIndex ) const; 00159 String substring( unsigned int beginIndex, unsigned int endIndex ) const; 00160 00161 // modification 00162 void replace(char find, char replace); 00163 void replace(const String& find, const String& replace); 00164 void toLowerCase(void); 00165 void toUpperCase(void); 00166 void trim(void); 00167 00168 // parsing/conversion 00169 long toInt(void) const; 00170 00171 protected: 00172 char *buffer; // the actual char array 00173 unsigned int capacity; // the array length minus one (for the '\0') 00174 unsigned int len; // the String length (not counting the '\0') 00175 unsigned char flags; // unused, for future features 00176 protected: 00177 void init(void); 00178 void invalidate(void); 00179 unsigned char changeBuffer(unsigned int maxStrLen); 00180 unsigned char concat(const char *cstr, unsigned int length); 00181 00182 // copy and move 00183 String & copy(const char *cstr, unsigned int length); 00184 #ifdef __GXX_EXPERIMENTAL_CXX0X__ 00185 void move(String &rhs); 00186 #endif 00187 }; 00188 00189 class StringSumHelper : public String 00190 { 00191 public: 00192 StringSumHelper(const String &s) : String(s) {} 00193 StringSumHelper(const char *p) : String(p) {} 00194 StringSumHelper(char c) : String(c) {} 00195 StringSumHelper(unsigned char num) : String(num) {} 00196 StringSumHelper(int num) : String(num) {} 00197 StringSumHelper(unsigned int num) : String(num) {} 00198 StringSumHelper(long num) : String(num) {} 00199 StringSumHelper(unsigned long num) : String(num) {} 00200 }; 00201 00202 #endif // __cplusplus 00203 #endif // __ARDUINOAPI_WSTRING_H__
Generated on Mon Jul 25 2022 12:21:32 by
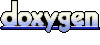