
Source code for the Curilights Controller. See http://www.saccade.com/writing/projects/CuriController/ for details.
Dependencies: FatFileSystem mbed
UserInterface.h
00001 // 00002 // User interface implementation 00003 // 00004 00005 #ifndef _USERINTERFACE_ 00006 #define _USERINTERFACE_ 00007 00008 #ifndef _UIMENU_ 00009 #include "UIMenu.h" 00010 #endif 00011 00012 #ifndef _SETTINGSMENU_ 00013 #include "SettingsMenu.h" 00014 #endif 00015 00016 class HomeMenu; 00017 class LightString; 00018 00019 // The ControllerUI is a pure virtual class describing the 00020 // functions one of the light selectors needs to provide 00021 class ControllerUI 00022 { 00023 public: 00024 ControllerUI( CheapLCD * lcd, LightString * lights ) 00025 : fLCD( lcd ), fLights( lights ), fLightsOn( true ) {}; 00026 00027 virtual ~ControllerUI() {} 00028 00029 virtual void Display( bool on ) = 0; 00030 virtual int32_t KnobMoved( int32_t step ) = 0; 00031 00032 virtual void LightSwitch( bool on ); 00033 virtual void TurnOn() = 0; 00034 virtual bool AreLightsOn() const { return fLightsOn; } 00035 00036 protected: 00037 CheapLCD * fLCD; 00038 LightString * fLights; 00039 bool fLightsOn; 00040 }; 00041 00042 // PatternSelector lets you choose patterns stored on the SD card 00043 typedef vector<uint32_t> Pattern; 00044 00045 class PatternSelector : public ControllerUI 00046 { 00047 public: 00048 PatternSelector( CheapLCD * lcd, LightString * lights ); 00049 00050 virtual ~PatternSelector() {}; 00051 00052 virtual void Display( bool on ); 00053 virtual int32_t KnobMoved( int32_t step ); 00054 00055 virtual void TurnOn(); 00056 00057 private: 00058 void DrawPattern(); 00059 void SetLights(); 00060 00061 vector<string> fPatternNames; 00062 vector<Pattern> fPatterns; 00063 }; 00064 00065 // This selector lets you choose among saturated colors 00066 class ColorSelector : public ControllerUI 00067 { 00068 public: 00069 ColorSelector( CheapLCD * lcd, LightString * lights ) 00070 : ControllerUI( lcd, lights ) 00071 {} 00072 00073 virtual ~ColorSelector() {}; 00074 00075 virtual void Display( bool on ); 00076 virtual int32_t KnobMoved( int32_t step ); 00077 00078 virtual void TurnOn(); 00079 00080 private: 00081 void DrawCursor( bool drawSprite ); 00082 }; 00083 00084 // This selector lets you choose white lights 00085 // with a variety of brightness levels 00086 class WhiteSelector : public ControllerUI 00087 { 00088 public: 00089 WhiteSelector( CheapLCD * lcd, LightString * lights ) 00090 : ControllerUI( lcd, lights ) 00091 {} 00092 00093 virtual ~WhiteSelector() {}; 00094 00095 virtual void Display( bool on ); 00096 virtual int32_t KnobMoved( int32_t step ); 00097 00098 virtual void TurnOn(); 00099 00100 private: 00101 void SetLights(); 00102 void DrawLevel(); 00103 }; 00104 00105 // This is the generic interface the UI uses to choose a 00106 // selector and talk to it. 00107 typedef enum { kColorSelector = 0, kWhiteSelector = 1, kPatternSelector = 2 } ESelector; 00108 00109 class LightController : public PushKnobUI 00110 { 00111 public: 00112 LightController( HomeMenu * parent, CheapLCD * lcd, LightString * lights ); 00113 00114 virtual void Display( bool on ) { fSubController->Display( on ); } 00115 void LightSwitch( bool on ) { fSubController->LightSwitch( on ); } 00116 bool AreLightsOn() const { return fSubController->AreLightsOn(); } 00117 00118 void SetSelector( ESelector sel ); 00119 00120 protected: 00121 00122 virtual int32_t KnobMoved( int32_t step ); 00123 virtual void KnobPushed(); 00124 00125 SETUP_KNOB_CALLBACKS( LightController ) 00126 00127 private: 00128 HomeMenu * fParent; 00129 LightString * fLights; 00130 00131 ControllerUI * fSubController; 00132 ColorSelector fColorSelector; 00133 WhiteSelector fWhiteSelector; 00134 PatternSelector fPatternSelector; 00135 }; 00136 00137 // This implements the home-page menu 00138 // 00139 class HomeMenu : public UIMenu 00140 { 00141 public: 00142 HomeMenu( CheapLCD * lcd, LightString * lights ); 00143 virtual ~HomeMenu() {}; 00144 00145 void SetLightsOn( bool isOn ); 00146 00147 protected: 00148 void DoLightController( ESelector sel ); 00149 void DoToggleLightsOn(); 00150 00151 virtual void KnobPushed(); 00152 virtual void AttachButton( PushButton * button ) { button->attach( this, &HomeMenu::KnobPushed ); } 00153 00154 LightController fLightController; 00155 SettingsMenu fSettingsMenu; 00156 00157 private: 00158 bool fForcedOff; 00159 }; 00160 00161 #endif 00162
Generated on Wed Jul 13 2022 22:22:40 by
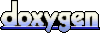