
MBED clock sync using NTP Server from from internet
Dependencies: EthernetInterface NTPClient mbed-rtos mbed
Fork of MbedClock by
main.cpp
00001 #include "mbed.h" 00002 #include "rtos.h" 00003 #include "EthernetInterface.h" 00004 #include "NTPClient.h" 00005 #include <string> 00006 00007 00008 EthernetInterface eth; 00009 TCPSocketConnection server; 00010 NTPClient ntp; 00011 DigitalOut led1(LED1); 00012 DigitalOut led2(LED2); 00013 time_t rtcTime; 00014 char tStr[32]; 00015 00016 00017 int main() 00018 { 00019 00020 printf("Setting up ethernet interface...\r\n"); 00021 if (eth.init() == 0 ) { //Use DHCP 00022 printf("Ethernet setup OK\r\n"); 00023 } else { 00024 printf("Error: cannot set ethernet interface\r\n"); 00025 return 1; 00026 } 00027 00028 printf("Trying to connect...\r\n"); 00029 wait(0.5); 00030 if ( eth.connect(30000) == 0 ) { 00031 printf("IP Address is %s\r\n", eth.getIPAddress()); 00032 } else { 00033 printf("Error: cannot set ethernet interface\r\n"); 00034 return 1; 00035 } 00036 00037 printf("Trying to update time...\r\n"); 00038 if (ntp.setTime("0.fr.pool.ntp.org") == 0) { //set RTC time 00039 printf("Set time successfully\r\n"); 00040 wait(1); 00041 rtcTime = time(NULL); //read the time 00042 printf("Current time is: %s\r\n", ctime(&rtcTime)); 00043 } else { 00044 printf("Error: Cannot set time\r\n"); 00045 } 00046 00047 printf("Disconnecting...\r\n"); 00048 eth.disconnect(); 00049 wait(1); 00050 printf("Ethernet disconnected\r\n"); 00051 00052 while(1) { 00053 rtcTime = time(NULL); //read the time 00054 strftime(tStr, 32, "%X\n", localtime(&rtcTime)); 00055 printf("Time as a custom formatted string = %s\r\n", tStr); 00056 wait(1); 00057 } 00058 }
Generated on Tue Jul 26 2022 00:46:09 by
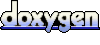