MPL3115A2_for_weather_shield
Dependents: SPARKFUN_WEATHER_SHIELD
Fork of MPL3115A2 by
Altitude.h
00001 /* 00002 MPL3115A2 Barometric Pressure and Tempurature Sensor Library 00003 By: Michael Lange 00004 Date: March 31, 2014 00005 License: This code is public domain. 00006 00007 This class encapsulates an altitude reading from the sensor. 00008 00009 */ 00010 00011 00012 #ifndef ALTITUDE_H 00013 #define ALTITUDE_H 00014 00015 #include "mbed.h" 00016 00017 //! Casting a float to a char truncates, therefore negative numbers become positive. 00018 //! This will properly cast a float in the range -128 to 127 to a char. 00019 #define float_to_char(x) (((x)<0)?(-(char)(x)):((char)(x))) 00020 00021 //! Altitude provides a wrapper around altitude data coming from the sensor. The class handles 00022 //! working with compressed data from the sensor and provides convenient functions for retreiving 00023 //! the data in various units (with room to add more if needed). 00024 class Altitude 00025 { 00026 public: 00027 00028 //! The size of the compressed data buffer from the sensor. Used in an I2C read. 00029 static const int size = 3; 00030 00031 //! The units we support converting the sensor data to. 00032 enum unitsType { METERS, FEET }; 00033 00034 Altitude(); 00035 Altitude(float a, unitsType units = FEET); 00036 Altitude(const char* compressed); 00037 Altitude(const char msb, const char csb, const char lsb); 00038 00039 //! Allows using the object directly in an I2C read operation. 00040 operator char*(void) { return _compressed; } 00041 //! Same as calling altitude with METERS as the parameter. 00042 operator float(void) { return _altitude; } 00043 00044 //! Returns the altitude in the units you specifiy, defaulting to FEET if none specified. 00045 float altitude(unitsType units = FEET); 00046 //! Call to decompress the sensor data after an I2C read. 00047 void setAltitude(); 00048 void setAltitude(const char* compressed); 00049 void setAltitude(const char msb, const char csb, const char lsb); 00050 void setAltitude(float a, unitsType units = FEET); 00051 00052 //! Returns the altitude as a string in the units specified, defaulting to FEET if none specified. 00053 const char* print(unitsType units = FEET); 00054 00055 //! Converts meters to feet. 00056 static float MetersToFeet(float meters) { return meters * 3.28084; } 00057 //! Converts feet to meters. 00058 static float FeetToMeters(float feet) { return feet / 3.28084; } 00059 00060 private: 00061 float _altitude; 00062 char _compressed[3]; 00063 char _printBuffer[9]; 00064 }; 00065 00066 #endif // ALTITUDE_H
Generated on Wed Jul 20 2022 03:44:08 by
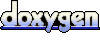