Nanopb is a plain-C implementation of Google's Protocol Buffers data format. It is targeted at 32 bit microcontrollers, but is also fit for other embedded systems with tight (2-10 kB ROM, <1 kB RAM) memory constraints.
Dependents: FBRLogger Dumb_box_rev2
pb_encode.h
00001 #ifndef _PB_ENCODE_H_ 00002 #define _PB_ENCODE_H_ 00003 00004 /* pb_encode.h: Functions to encode protocol buffers. Depends on pb_encode.c. 00005 * The main function is pb_encode. You also need an output stream, structures 00006 * and their field descriptions (just like with pb_decode). 00007 */ 00008 00009 #include <stdbool.h> 00010 #include "pb.h" 00011 00012 #ifdef __cplusplus 00013 extern "C" { 00014 #endif 00015 00016 /* Lightweight output stream. 00017 * You can provide callback for writing or use pb_ostream_from_buffer. 00018 * 00019 * Alternatively, callback can be NULL in which case the stream will just 00020 * count the number of bytes that would have been written. In this case 00021 * max_size is not checked. 00022 * 00023 * Rules for callback: 00024 * 1) Return false on IO errors. This will cause encoding to abort. 00025 * 00026 * 2) You can use state to store your own data (e.g. buffer pointer). 00027 * 00028 * 3) pb_write will update bytes_written after your callback runs. 00029 * 00030 * 4) Substreams will modify max_size and bytes_written. Don't use them to 00031 * calculate any pointers. 00032 */ 00033 struct _pb_ostream_t 00034 { 00035 #ifdef PB_BUFFER_ONLY 00036 /* Callback pointer is not used in buffer-only configuration. 00037 * Having an int pointer here allows binary compatibility but 00038 * gives an error if someone tries to assign callback function. 00039 * Also, NULL pointer marks a 'sizing stream' that does not 00040 * write anything. 00041 */ 00042 int *callback; 00043 #else 00044 bool (*callback)(pb_ostream_t *stream, const uint8_t *buf, size_t count); 00045 #endif 00046 void *state; /* Free field for use by callback implementation */ 00047 size_t max_size; /* Limit number of output bytes written (or use SIZE_MAX). */ 00048 size_t bytes_written; 00049 }; 00050 00051 pb_ostream_t pb_ostream_from_buffer(uint8_t *buf, size_t bufsize); 00052 bool pb_write(pb_ostream_t *stream, const uint8_t *buf, size_t count); 00053 00054 /* Encode struct to given output stream. 00055 * Returns true on success, false on any failure. 00056 * The actual struct pointed to by src_struct must match the description in fields. 00057 * All required fields in the struct are assumed to have been filled in. 00058 */ 00059 bool pb_encode(pb_ostream_t *stream, const pb_field_t fields[], const void *src_struct); 00060 00061 /* --- Helper functions --- 00062 * You may want to use these from your caller or callbacks. 00063 */ 00064 00065 /* Encode field header based on LTYPE and field number defined in the field structure. 00066 * Call this from the callback before writing out field contents. */ 00067 bool pb_encode_tag_for_field(pb_ostream_t *stream, const pb_field_t *field); 00068 00069 /* Encode field header by manually specifing wire type. You need to use this if 00070 * you want to write out packed arrays from a callback field. */ 00071 bool pb_encode_tag(pb_ostream_t *stream, pb_wire_type_t wiretype, uint32_t field_number); 00072 00073 /* Encode an integer in the varint format. 00074 * This works for bool, enum, int32, int64, uint32 and uint64 field types. */ 00075 bool pb_encode_varint(pb_ostream_t *stream, uint64_t value); 00076 00077 /* Encode an integer in the zig-zagged svarint format. 00078 * This works for sint32 and sint64. */ 00079 bool pb_encode_svarint(pb_ostream_t *stream, int64_t value); 00080 00081 /* Encode a string or bytes type field. For strings, pass strlen(s) as size. */ 00082 bool pb_encode_string(pb_ostream_t *stream, const uint8_t *buffer, size_t size); 00083 00084 /* Encode a fixed32, sfixed32 or float value. 00085 * You need to pass a pointer to a 4-byte wide C variable. */ 00086 bool pb_encode_fixed32(pb_ostream_t *stream, const void *value); 00087 00088 /* Encode a fixed64, sfixed64 or double value. 00089 * You need to pass a pointer to a 8-byte wide C variable. */ 00090 bool pb_encode_fixed64(pb_ostream_t *stream, const void *value); 00091 00092 /* Encode a submessage field. 00093 * You need to pass the pb_field_t array and pointer to struct, just like with pb_encode(). 00094 * This internally encodes the submessage twice, first to calculate message size and then to actually write it out. 00095 */ 00096 bool pb_encode_submessage(pb_ostream_t *stream, const pb_field_t fields[], const void *src_struct); 00097 00098 /* --- Internal functions --- 00099 * These functions are not terribly useful for the average library user, but 00100 * are exported to make the unit testing and extending nanopb easier. 00101 */ 00102 00103 #ifdef NANOPB_INTERNALS 00104 bool pb_enc_varint(pb_ostream_t *stream, const pb_field_t *field, const void *src); 00105 bool pb_enc_svarint(pb_ostream_t *stream, const pb_field_t *field, const void *src); 00106 bool pb_enc_fixed32(pb_ostream_t *stream, const pb_field_t *field, const void *src); 00107 bool pb_enc_fixed64(pb_ostream_t *stream, const pb_field_t *field, const void *src); 00108 bool pb_enc_bytes(pb_ostream_t *stream, const pb_field_t *field, const void *src); 00109 bool pb_enc_string(pb_ostream_t *stream, const pb_field_t *field, const void *src); 00110 #endif 00111 00112 /* This function is not recommended for new programs. Use pb_encode_submessage() 00113 * instead, it has the same functionality with a less confusing interface. */ 00114 bool pb_enc_submessage(pb_ostream_t *stream, const pb_field_t *field, const void *src); 00115 00116 #ifdef __cplusplus 00117 } /* extern "C" */ 00118 #endif 00119 00120 #endif
Generated on Tue Jul 12 2022 21:22:20 by
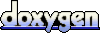