Nanopb is a plain-C implementation of Google's Protocol Buffers data format. It is targeted at 32 bit microcontrollers, but is also fit for other embedded systems with tight (2-10 kB ROM, <1 kB RAM) memory constraints.
Dependents: FBRLogger Dumb_box_rev2
pb_decode.h
00001 #ifndef _PB_DECODE_H_ 00002 #define _PB_DECODE_H_ 00003 00004 /* pb_decode.h: Functions to decode protocol buffers. Depends on pb_decode.c. 00005 * The main function is pb_decode. You will also need to create an input 00006 * stream, which is easiest to do with pb_istream_from_buffer(). 00007 * 00008 * You also need structures and their corresponding pb_field_t descriptions. 00009 * These are usually generated from .proto-files with a script. 00010 */ 00011 00012 #include <stdbool.h> 00013 #include "pb.h" 00014 00015 #ifdef __cplusplus 00016 extern "C" { 00017 #endif 00018 00019 /* Lightweight input stream. 00020 * You can provide a callback function for reading or use 00021 * pb_istream_from_buffer. 00022 * 00023 * Rules for callback: 00024 * 1) Return false on IO errors. This will cause decoding to abort. 00025 * 00026 * 2) You can use state to store your own data (e.g. buffer pointer), 00027 * and rely on pb_read to verify that no-body reads past bytes_left. 00028 * 00029 * 3) Your callback may be used with substreams, in which case bytes_left 00030 * is different than from the main stream. Don't use bytes_left to compute 00031 * any pointers. 00032 */ 00033 struct _pb_istream_t 00034 { 00035 #ifdef PB_BUFFER_ONLY 00036 /* Callback pointer is not used in buffer-only configuration. 00037 * Having an int pointer here allows binary compatibility but 00038 * gives an error if someone tries to assign callback function. 00039 */ 00040 int *callback; 00041 #else 00042 bool (*callback)(pb_istream_t *stream, uint8_t *buf, size_t count); 00043 #endif 00044 00045 void *state; /* Free field for use by callback implementation */ 00046 size_t bytes_left; 00047 00048 #ifndef PB_NO_ERRMSG 00049 const char *errmsg; 00050 #endif 00051 }; 00052 00053 pb_istream_t pb_istream_from_buffer(uint8_t *buf, size_t bufsize); 00054 bool pb_read(pb_istream_t *stream, uint8_t *buf, size_t count); 00055 00056 /* Decode from stream to destination struct. 00057 * Returns true on success, false on any failure. 00058 * The actual struct pointed to by dest must match the description in fields. 00059 */ 00060 bool pb_decode(pb_istream_t *stream, const pb_field_t fields[], void *dest_struct); 00061 00062 /* Same as pb_decode, except does not initialize the destination structure 00063 * to default values. This is slightly faster if you need no default values 00064 * and just do memset(struct, 0, sizeof(struct)) yourself. 00065 */ 00066 bool pb_decode_noinit(pb_istream_t *stream, const pb_field_t fields[], void *dest_struct); 00067 00068 /* --- Helper functions --- 00069 * You may want to use these from your caller or callbacks. 00070 */ 00071 00072 /* Decode the tag for the next field in the stream. Gives the wire type and 00073 * field tag. At end of the message, returns false and sets eof to true. */ 00074 bool pb_decode_tag(pb_istream_t *stream, pb_wire_type_t *wire_type, uint32_t *tag, bool *eof); 00075 00076 /* Skip the field payload data, given the wire type. */ 00077 bool pb_skip_field(pb_istream_t *stream, pb_wire_type_t wire_type); 00078 00079 /* Decode an integer in the varint format. This works for bool, enum, int32, 00080 * int64, uint32 and uint64 field types. */ 00081 bool pb_decode_varint(pb_istream_t *stream, uint64_t *dest); 00082 00083 /* Decode an integer in the zig-zagged svarint format. This works for sint32 00084 * and sint64. */ 00085 bool pb_decode_svarint(pb_istream_t *stream, int64_t *dest); 00086 00087 /* Decode a fixed32, sfixed32 or float value. You need to pass a pointer to 00088 * a 4-byte wide C variable. */ 00089 bool pb_decode_fixed32(pb_istream_t *stream, void *dest); 00090 00091 /* Decode a fixed64, sfixed64 or double value. You need to pass a pointer to 00092 * a 8-byte wide C variable. */ 00093 bool pb_decode_fixed64(pb_istream_t *stream, void *dest); 00094 00095 /* Make a limited-length substream for reading a PB_WT_STRING field. */ 00096 bool pb_make_string_substream(pb_istream_t *stream, pb_istream_t *substream); 00097 void pb_close_string_substream(pb_istream_t *stream, pb_istream_t *substream); 00098 00099 /* --- Internal functions --- 00100 * These functions are not terribly useful for the average library user, but 00101 * are exported to make the unit testing and extending nanopb easier. 00102 */ 00103 00104 #ifdef NANOPB_INTERNALS 00105 bool pb_dec_varint(pb_istream_t *stream, const pb_field_t *field, void *dest); 00106 bool pb_dec_svarint(pb_istream_t *stream, const pb_field_t *field, void *dest); 00107 bool pb_dec_fixed32(pb_istream_t *stream, const pb_field_t *field, void *dest); 00108 bool pb_dec_fixed64(pb_istream_t *stream, const pb_field_t *field, void *dest); 00109 00110 bool pb_dec_bytes(pb_istream_t *stream, const pb_field_t *field, void *dest); 00111 bool pb_dec_string(pb_istream_t *stream, const pb_field_t *field, void *dest); 00112 bool pb_dec_submessage(pb_istream_t *stream, const pb_field_t *field, void *dest); 00113 00114 bool pb_skip_varint(pb_istream_t *stream); 00115 bool pb_skip_string(pb_istream_t *stream); 00116 #endif 00117 00118 #ifdef __cplusplus 00119 } /* extern "C" */ 00120 #endif 00121 00122 #endif
Generated on Tue Jul 12 2022 21:22:20 by
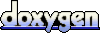