Embed:
(wiki syntax)
Show/hide line numbers
SteeringTire.h
00001 #ifndef INCLUDED_STEERING_TIRE_H 00002 #define INCLUDED_STEERING_TIRE_H 00003 00004 #include "Steering.h" 00005 #include <stdint.h> 00006 #include "mbed.h" 00007 class Command; 00008 class I2CMotor; 00009 00010 class SteeringTire{ 00011 public: 00012 SteeringTire( I2CMotor** tire ); 00013 ~SteeringTire(); 00014 void update( Steering::ActionType action, Command command ); 00015 00016 private: 00017 void updateMove( Command command ); 00018 void updateRoll( Command command ); 00019 void updateStop( Command command ); 00020 void updateWaitServo( Command command ); 00021 00022 static const uint32_t mReleaseStopTime_ms[]; 00023 static const float mBrakeReleaseThreshold; 00024 00025 I2CMotor** mTire; 00026 00027 bool mIsReleaseStop; 00028 Timer* mTimer; 00029 Steering::ActionType mPrevActionType; 00030 }; 00031 00032 #endif
Generated on Sat Jul 23 2022 01:56:48 by
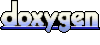