Dependencies: AmmoPusher AmmoSupplier Shooter
ShootingSystem.h
00001 #ifndef INCLUDED_SHOOTING_SYSTEM_H 00002 #define INCLUDED_SHOOTING_SYSTEM_H 00003 00004 class I2CServo; 00005 class I2CMotor; 00006 class Command; 00007 class AmmoPusher; 00008 class AmmoSupplier; 00009 class AmmoSupplierManager; 00010 class Shooter; 00011 #include "mbed.h" 00012 00013 class ShootingSystem{ 00014 public: 00015 enum AimState{ 00016 AIM_OWN_POLE = 0x00, 00017 AIM_CENTER_MIDDLE_POLE = 0x40, 00018 AIM_CENTER_SIDE_POLE = 0x80, 00019 AIM_ENEMYS_POLE = 0xC0 00020 }; 00021 00022 enum State{ 00023 WAITING, 00024 SHOOTING, 00025 SHOOT_PREPARATION, 00026 SHOOT_WAITING, 00027 AMMO_SUPPLYING 00028 }; 00029 00030 static bool isShootable(){ 00031 return mIsShootable; 00032 } 00033 00034 ShootingSystem( I2CServo* angleManagerServo, AmmoPusher* ammoPusher, 00035 AmmoSupplier* ammoSupplier, Shooter* shooter, 00036 I2CServo* positoinManager ); 00037 ~ShootingSystem(); 00038 00039 void update( Command command ); 00040 00041 private: 00042 void waiting( Command command ); 00043 void shooting(); 00044 void shootWaiting(); 00045 void shootPreparation(); 00046 void ammoSupply(); 00047 00048 static bool mIsShootable; 00049 00050 I2CServo* mShooterAngleServo; 00051 AmmoPusher* mAmmoPusher; 00052 AmmoSupplier* mAmmoSupplier; 00053 Shooter* mShooter; 00054 I2CServo* mPositionManager; 00055 State mState; 00056 Timer mTimer; 00057 }; 00058 00059 #endif
Generated on Mon Aug 1 2022 21:30:00 by
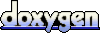