Joystick enabled version of USBHID -library. Has full Playstation 3 functionality including button pressures and a working home-button implementation, while maintaining full PC/MAC/linux -compatibility. basic operation of the lib: #include "mbed.h" #include "usbhid.h" USBJoystick joystick; int main() { while(1) { char dpad = 0xf; /*only the rightmost 4 bits matter*/ short button = 0xff; /*only the rightmost 13 bits matter*/ /*buttons are square, cross, circle, triangle, l1, r1, l2, r2, l3, r3, home.*/ char stick1x = 0; char stick1y = 0; char stick2x = 0; char stick2y = 0; joystick.joystick(dpad, buttons, stick1x, stick1y, stick2x, stick2y); wait_ms(5); } }
usbhid.cpp
00001 /* usbhid.cpp */ 00002 /* USB HID class device */ 00003 /* Copyright (c) Phil Wright 2008 */ 00004 00005 /* modified by Shinichiro Oba <http://mbed.org/users/bricklife/> */ 00006 00007 /* additional modification (PS3 home button functionality) by Panu Kauppinen*/ 00008 00009 #include "mbed.h" 00010 #include "usbhid.h" 00011 #include "asciihid.h" 00012 00013 /* Endpoint packet sizes */ 00014 #define MAX_PACKET_SIZE_EP1 (64) 00015 00016 /* HID Class */ 00017 #define HID_CLASS (3) 00018 #define HID_SUBCLASS_NONE (0) 00019 #define HID_PROTOCOL_NONE (0) 00020 #define HID_DESCRIPTOR (33) 00021 #define REPORT_DESCRIPTOR (34) 00022 00023 /* Class requests */ 00024 #define GET_REPORT (0x1) 00025 #define GET_IDLE (0x2) 00026 #define SET_REPORT (0x9) 00027 #define SET_IDLE (0xa) 00028 00029 00030 /* HID Class Report Descriptor */ 00031 /* Short items: size is 0, 1, 2 or 3 specifying 0, 1, 2 or 4 (four) bytes of data as per HID Class standard */ 00032 00033 /* Main items */ 00034 #define INPUT(size) (0x80 | size) 00035 #define OUTPUT(size) (0x90 | size) 00036 #define FEATURE(size) (0xb0 | size) 00037 #define COLLECTION(size) (0xa0 | size) 00038 #define END_COLLECTION(size) (0xc0 | size) 00039 00040 /* Global items */ 00041 #define USAGE_PAGE(size) (0x04 | size) 00042 #define LOGICAL_MIN(size) (0x14 | size) 00043 #define LOGICAL_MAX(size) (0x24 | size) 00044 #define PHYSICAL_MIN(size) (0x34 | size) 00045 #define PHYSICAL_MAX(size) (0x44 | size) 00046 #define UNIT_EXPONENT(size) (0x54 | size) 00047 #define UNIT(size) (0x64 | size) 00048 #define REPORT_SIZE(size) (0x74 | size) 00049 #define REPORT_ID(size) (0x84 | size) 00050 #define REPORT_COUNT(size) (0x94 | size) 00051 #define PUSH(size) (0xa4 | size) 00052 #define POP(size) (0xb4 | size) 00053 00054 /* Local items */ 00055 #define USAGE(size) (0x08 | size) 00056 #define USAGE_MIN(size) (0x18 | size) 00057 #define USAGE_MAX(size) (0x28 | size) 00058 #define DESIGNATOR_INDEX(size) (0x38 | size) 00059 #define DESIGNATOR_MIN(size) (0x48 | size) 00060 #define DESIGNATOR_MAX(size) (0x58 | size) 00061 #define STRING_INDEX(size) (0x78 | size) 00062 #define STRING_MIN(size) (0x88 | size) 00063 #define STRING_MAX(size) (0x98 | size) 00064 #define DELIMITER(size) (0xa8 | size) 00065 00066 #define REPORT_ID_KEYBOARD (1) 00067 #define REPORT_ID_MOUSE (2) 00068 00069 #define MAX_REPORT_SIZE (64) 00070 00071 unsigned char reportDescriptor[] = { 00072 /* Keyboard */ 00073 USAGE_PAGE(1), 0x01, 00074 USAGE(1), 0x06, 00075 COLLECTION(1), 0x01, 00076 REPORT_ID(1), REPORT_ID_KEYBOARD, 00077 USAGE_PAGE(1), 0x07, 00078 USAGE_MIN(1), 0xE0, 00079 USAGE_MAX(1), 0xE7, 00080 LOGICAL_MIN(1), 0x00, 00081 LOGICAL_MAX(1), 0x01, 00082 REPORT_SIZE(1), 0x01, 00083 REPORT_COUNT(1), 0x08, 00084 INPUT(1), 0x02, 00085 REPORT_COUNT(1), 0x01, 00086 REPORT_SIZE(1), 0x08, 00087 INPUT(1), 0x01, 00088 REPORT_COUNT(1), 0x05, 00089 REPORT_SIZE(1), 0x01, 00090 USAGE_PAGE(1), 0x08, 00091 USAGE_MIN(1), 0x01, 00092 USAGE_MAX(1), 0x05, 00093 OUTPUT(1), 0x02, 00094 REPORT_COUNT(1), 0x01, 00095 REPORT_SIZE(1), 0x03, 00096 OUTPUT(1), 0x01, 00097 REPORT_COUNT(1), 0x06, 00098 REPORT_SIZE(1), 0x08, 00099 LOGICAL_MIN(1), 0x00, 00100 LOGICAL_MAX(2), 0xff, 0x00, 00101 USAGE_PAGE(1), 0x07, 00102 USAGE_MIN(1), 0x00, 00103 USAGE_MAX(2), 0xff, 0x00, 00104 INPUT(1), 0x00, 00105 END_COLLECTION(0), 00106 00107 /* Mouse */ 00108 USAGE_PAGE(1), 0x01, 00109 USAGE(1), 0x02, 00110 COLLECTION(1), 0x01, 00111 USAGE(1), 0x01, 00112 COLLECTION(1), 0x00, 00113 REPORT_ID(1), REPORT_ID_MOUSE, 00114 REPORT_COUNT(1), 0x03, 00115 REPORT_SIZE(1), 0x01, 00116 USAGE_PAGE(1), 0x09, 00117 USAGE_MIN(1), 0x1, 00118 USAGE_MAX(1), 0x3, 00119 LOGICAL_MIN(1), 0x00, 00120 LOGICAL_MAX(1), 0x01, 00121 INPUT(1), 0x02, 00122 REPORT_COUNT(1), 0x01, 00123 REPORT_SIZE(1), 0x05, 00124 INPUT(1), 0x01, 00125 REPORT_COUNT(1), 0x03, 00126 REPORT_SIZE(1), 0x08, 00127 USAGE_PAGE(1), 0x01, 00128 USAGE(1), 0x30, 00129 USAGE(1), 0x31, 00130 USAGE(1), 0x38, 00131 LOGICAL_MIN(1), 0x81, 00132 LOGICAL_MAX(1), 0x7f, 00133 INPUT(1), 0x06, 00134 END_COLLECTION(0), 00135 END_COLLECTION(0), 00136 }; 00137 00138 00139 00140 00141 /* Descriptors */ 00142 unsigned char deviceDescriptor[] = { 00143 0x12, /* bLength */ 00144 DEVICE_DESCRIPTOR, /* bDescriptorType */ 00145 0x10, /* bcdUSB (LSB) */ 00146 0x01, /* bcdUSB (MSB) */ 00147 0x00, /* bDeviceClass */ 00148 0x00, /* bDeviceSubClass */ 00149 0x00, /* bDeviceprotocol */ 00150 MAX_PACKET_SIZE_EP0, /* bMaxPacketSize0 */ 00151 0xC4, /* idVendor (LSB) */ 00152 0x10, /* idVendor (MSB) */ 00153 0xC0, /* idProduct (LSB) */ 00154 0x82, /* idProduct (MSB) */ 00155 0x01, /* bcdDevice (LSB) */ 00156 0x00, /* bcdDevice (MSB) */ 00157 0x01, /* iManufacturer */ 00158 0x02, /* iProduct */ 00159 0x00, /* iSerialNumber */ 00160 0x01 /* bNumConfigurations */ 00161 }; 00162 00163 unsigned char configurationDescriptor[] = { 00164 0x09, /* bLength */ 00165 CONFIGURATION_DESCRIPTOR, /* bDescriptorType */ 00166 0x09+0x09+0x09+0x07, /* wTotalLength (LSB) */ 00167 0x00, /* wTotalLength (MSB) */ 00168 0x01, /* bNumInterfaces */ 00169 0x01, /* bConfigurationValue */ 00170 0x00, /* iConfiguration */ 00171 0x80, /* bmAttributes */ 00172 0x32, /* bMaxPower */ 00173 00174 0x09, /* bLength */ 00175 INTERFACE_DESCRIPTOR, /* bDescriptorType */ 00176 0x00, /* bInterfaceNumber */ 00177 0x00, /* bAlternateSetting */ 00178 0x01, /* bNumEndpoints */ 00179 HID_CLASS, /* bInterfaceClass */ 00180 HID_SUBCLASS_NONE, /* bInterfaceSubClass */ 00181 HID_PROTOCOL_NONE, /* bInterfaceProtocol */ 00182 0x00, /* iInterface */ 00183 00184 0x09, /* bLength */ 00185 HID_DESCRIPTOR, /* bDescriptorType */ 00186 0x11, /* bcdHID (LSB) */ 00187 0x01, /* bcdHID (MSB) */ 00188 0x00, /* bCountryCode */ 00189 0x01, /* bNumDescriptors */ 00190 REPORT_DESCRIPTOR, /* bDescriptorType */ 00191 0x79, /* wDescriptorLength (LSB) */ 00192 0x00, /* wDescriptorLength (MSB) */ 00193 00194 0x07, /* bLength */ 00195 ENDPOINT_DESCRIPTOR, /* bDescriptorType */ 00196 0x81, /* bEndpointAddress */ 00197 0x03, /* bmAttributes */ 00198 MAX_PACKET_SIZE_EP1, /* wMaxPacketSize (LSB) */ 00199 0x00, /* wMaxPacketSize (MSB) */ 00200 0x05, /* bInterval */ 00201 }; 00202 00203 00204 00205 volatile bool complete; 00206 volatile bool configured; 00207 unsigned char outputReport[MAX_REPORT_SIZE]; 00208 00209 usbhid::usbhid() 00210 { 00211 configured = false; 00212 connect(); 00213 } 00214 00215 void usbhid::deviceEventReset() 00216 { 00217 configured = false; 00218 00219 /* Must call base class */ 00220 usbdevice::deviceEventReset(); 00221 } 00222 00223 bool usbhid::requestSetConfiguration(void) 00224 { 00225 bool result; 00226 00227 /* Configure IN interrupt endpoint */ 00228 realiseEndpoint(EP1IN, MAX_PACKET_SIZE_EP1); 00229 enableEndpointEvent(EP1IN); 00230 00231 /* Must call base class */ 00232 result = usbdevice::requestSetConfiguration(); 00233 00234 if (result) 00235 { 00236 /* Now configured */ 00237 configured = true; 00238 } 00239 00240 return result; 00241 } 00242 00243 bool usbhid::requestGetDescriptor(void) 00244 { 00245 bool success = false; 00246 00247 00248 switch (DESCRIPTOR_TYPE(transfer.setup.wValue)) 00249 { 00250 case DEVICE_DESCRIPTOR: 00251 transfer.remaining = sizeof(deviceDescriptor); 00252 transfer.ptr = deviceDescriptor; 00253 transfer.direction = DEVICE_TO_HOST; 00254 success = true; 00255 break; 00256 case CONFIGURATION_DESCRIPTOR: 00257 transfer.remaining = sizeof(configurationDescriptor); 00258 transfer.ptr = configurationDescriptor; 00259 transfer.direction = DEVICE_TO_HOST; 00260 success = true; 00261 break; 00262 case STRING_DESCRIPTOR: 00263 case INTERFACE_DESCRIPTOR: 00264 case ENDPOINT_DESCRIPTOR: 00265 /* TODO: Support is optional, not implemented here */ 00266 break; 00267 case HID_DESCRIPTOR: 00268 transfer.remaining = 0x09; /* TODO: Fix hard coded size/offset */ 00269 transfer.ptr = &configurationDescriptor[18]; 00270 transfer.direction = DEVICE_TO_HOST; 00271 success = true; 00272 break; 00273 case REPORT_DESCRIPTOR: 00274 transfer.remaining = sizeof(reportDescriptor); 00275 transfer.ptr = reportDescriptor; 00276 transfer.direction = DEVICE_TO_HOST; 00277 success = true; 00278 break; 00279 default: 00280 break; 00281 } 00282 00283 return success; 00284 } 00285 00286 bool usbhid::requestSetup(void) 00287 { 00288 /* Process class requests */ 00289 bool success = false; 00290 00291 if (transfer.setup.bmRequestType.Type == CLASS_TYPE) 00292 { 00293 switch (transfer.setup.bRequest) 00294 { 00295 case SET_REPORT: 00296 switch (transfer.setup.wValue & 0xff) 00297 { 00298 case REPORT_ID_KEYBOARD: 00299 /* TODO: LED state */ 00300 transfer.remaining = sizeof(outputReport); 00301 transfer.ptr = outputReport; 00302 transfer.direction = HOST_TO_DEVICE; 00303 success = true; 00304 break; 00305 default: 00306 break; 00307 } 00308 break; 00309 default: 00310 break; 00311 } 00312 } 00313 00314 if (success) 00315 { 00316 /* We've handled this request */ 00317 return true; 00318 } 00319 00320 return usbdevice::requestSetup(); 00321 } 00322 00323 bool usbhid::sendInputReport(unsigned char id, unsigned char *data, unsigned char size) 00324 { 00325 /* Send an Input Report */ 00326 /* If data is NULL an all zero report is sent */ 00327 00328 static unsigned char report[MAX_REPORT_SIZE+1]; /* +1 for report ID */ 00329 unsigned char i; 00330 00331 if (size > MAX_REPORT_SIZE) 00332 { 00333 return false; 00334 } 00335 00336 /* Add report ID */ 00337 report[0]=id; 00338 00339 /* Add report data */ 00340 if (data != NULL) 00341 { 00342 for (i=0; i<size; i++) 00343 { 00344 report[i+1] = *data++; 00345 } 00346 } 00347 else 00348 { 00349 for (i=0; i<size; i++) 00350 { 00351 report[i+1] = 0; 00352 } 00353 } 00354 00355 /* Block if not configured */ 00356 while (!configured); 00357 00358 /* Send report */ 00359 complete = false; 00360 disableEvents(); 00361 endpointWrite(EP1IN, report, size+1); /* +1 for report ID */ 00362 enableEvents(); 00363 00364 /* Wait for completion */ 00365 while(!complete && configured); 00366 return true; 00367 } 00368 00369 void usbhid::endpointEventEP1In(void) 00370 { 00371 complete = true; 00372 } 00373 00374 bool usbhid::keyboard(char c) 00375 { 00376 /* Send a simulated keyboard keypress. Returns true if successful. */ 00377 unsigned char report[8]={0,0,0,0,0,0,0,0}; 00378 00379 report[0] = keymap[c].modifier; 00380 report[2] = keymap[c].usage; 00381 00382 /* Key down */ 00383 if (!sendInputReport(REPORT_ID_KEYBOARD, report, 8)) 00384 { 00385 return false; 00386 } 00387 00388 /* Key up */ 00389 if (!sendInputReport(REPORT_ID_KEYBOARD, NULL, 8)) 00390 { 00391 return false; 00392 } 00393 00394 return true; 00395 } 00396 00397 bool usbhid::keyboard(char *string) 00398 { 00399 /* Send a string of characters. Returns true if successful. */ 00400 do { 00401 if (!keyboard(*string++)) 00402 { 00403 return false; 00404 } 00405 } while (*string != '\0'); 00406 00407 return true; 00408 } 00409 00410 bool usbhid::mouse(signed char x, signed char y, unsigned char buttons, signed char wheel) 00411 { 00412 /* Send a simulated mouse event. Returns true if successful. */ 00413 unsigned char report[4]={0,0,0,0}; 00414 00415 report[0] = buttons; 00416 report[1] = x; 00417 report[2] = y; 00418 report[3] = wheel; 00419 00420 if (!sendInputReport(REPORT_ID_MOUSE, report, 4)) 00421 { 00422 return false; 00423 } 00424 00425 return true; 00426 } 00427 00428 00429 // 00430 // USBJoystick 00431 // 00432 00433 unsigned char magic_init_bytes[] = {0x21, 0x26, 0x01, 0x07, 0x00, 0x00, 0x00, 0x00}; // PS3 will request these bytes as a class type report 0x0300 00434 00435 /* Joystick */ 00436 unsigned char reportDescriptorJoystick[] = { 00437 0x05, 0x01, // USAGE_PAGE (Generic Desktop) 00438 0x09, 0x05, // USAGE (Gamepad) 00439 0xa1, 0x01, // COLLECTION (Application) 00440 00441 0x15, 0x00, // LOGICAL_MINIMUM (0) 00442 0x25, 0x01, // LOGICAL_MAXIMUM (1) 00443 0x35, 0x00, // PHYSICAL_MINIMUM (0) 00444 0x45, 0x01, // PHYSICAL_MAXIMUM (1) 00445 0x75, 0x01, // REPORT_SIZE (1) 00446 0x95, 0x0d, // REPORT_COUNT (13) 00447 0x05, 0x09, // USAGE_PAGE (Button) 00448 0x19, 0x01, // USAGE_MINIMUM (Button 1) 00449 0x29, 0x0d, // USAGE_MAXIMUM (Button 13) 00450 0x81, 0x02, // INPUT (Data,Var,Abs) 00451 0x95, 0x03, // REPORT_COUNT (3) 00452 0x81, 0x01, // INPUT (Cnst,Ary,Abs) 00453 0x05, 0x01, // USAGE_PAGE (Generic Desktop) 00454 0x25, 0x07, // LOGICAL_MAXIMUM (7) 00455 0x46, 0x3b, 0x01, // PHYSICAL_MAXIMUM (315) 00456 0x75, 0x04, // REPORT_SIZE (4) 00457 0x95, 0x01, // REPORT_COUNT (1) 00458 0x65, 0x14, // UNIT (Eng Rot:Angular Pos) 00459 0x09, 0x39, // USAGE (Hat switch) 00460 0x81, 0x42, // INPUT (Data,Var,Abs,Null) 00461 0x65, 0x00, // UNIT (None) 00462 0x95, 0x01, // REPORT_COUNT (1) 00463 0x81, 0x01, // INPUT (Cnst,Ary,Abs) 00464 00465 0x26, 0xff, 0x00, // LOGICAL_MAXIMUM (255) 00466 0x46, 0xff, 0x00, // PHYSICAL_MAXIMUM (255) 00467 0x09, 0x30, // USAGE (X) 00468 0x09, 0x31, // USAGE (Y) 00469 0x09, 0x32, // USAGE (Z) 00470 0x09, 0x35, // USAGE (Rz) 00471 0x75, 0x08, // REPORT_SIZE (8) 00472 0x95, 0x04, // REPORT_COUNT (4) 00473 0x81, 0x02, // INPUT (Data,Var,Abs) 00474 00475 0x06, 0x00, 0xff, // USAGE_PAGE (Vendor Specific) 00476 0x09, 0x20, // Unknown 00477 0x09, 0x21, // Unknown 00478 0x09, 0x22, // Unknown 00479 0x09, 0x23, // Unknown 00480 0x09, 0x24, // Unknown 00481 0x09, 0x25, // Unknown 00482 0x09, 0x26, // Unknown 00483 0x09, 0x27, // Unknown 00484 0x09, 0x28, // Unknown 00485 0x09, 0x29, // Unknown 00486 0x09, 0x2a, // Unknown 00487 0x09, 0x2b, // Unknown 00488 0x95, 0x0c, // REPORT_COUNT (12) 00489 0x81, 0x02, // INPUT (Data,Var,Abs) 00490 0x0a, 0x21, 0x26, // Unknown 00491 0x95, 0x08, // REPORT_COUNT (8) 00492 0xb1, 0x02, // FEATURE (Data,Var,Abs) 00493 00494 0xc0, // END_COLLECTION 00495 }; 00496 00497 USBJoystick::USBJoystick() 00498 { 00499 int reportDescriptorSize = sizeof(reportDescriptorJoystick); 00500 configurationDescriptor[25] = reportDescriptorSize & 0xff; 00501 configurationDescriptor[26] = (reportDescriptorSize >> 8) & 0xff; 00502 } 00503 00504 bool USBJoystick::requestGetDescriptor(void) 00505 { 00506 bool success = false; 00507 00508 switch (DESCRIPTOR_TYPE(transfer.setup.wValue)) 00509 { 00510 case REPORT_DESCRIPTOR: 00511 transfer.remaining = sizeof(reportDescriptorJoystick); 00512 transfer.ptr = reportDescriptorJoystick; 00513 transfer.direction = DEVICE_TO_HOST; 00514 success = true; 00515 break; 00516 default: 00517 success = usbhid::requestGetDescriptor(); 00518 break; 00519 } 00520 00521 return success; 00522 } 00523 00524 bool USBJoystick::sendInputReport(unsigned char *data, unsigned char size) 00525 { 00526 /* Send an Input Report */ 00527 /* If data is NULL an all zero report is sent */ 00528 00529 static unsigned char report[MAX_REPORT_SIZE]; 00530 unsigned char i; 00531 00532 if (size > MAX_REPORT_SIZE) 00533 { 00534 return false; 00535 } 00536 00537 /* Add report data */ 00538 if (data != NULL) 00539 { 00540 for (i=0; i<size; i++) 00541 { 00542 report[i] = *data++; 00543 } 00544 } 00545 else 00546 { 00547 for (i=0; i<size; i++) 00548 { 00549 report[i] = 0; 00550 } 00551 } 00552 00553 /* Block if not configured */ 00554 while (!configured); 00555 00556 00557 /* Send report */ 00558 complete = false; 00559 00560 disableEvents(); 00561 endpointWrite(EP1IN, report, size); 00562 enableEvents(); 00563 00564 00565 /* Wait for completion */ 00566 while(!complete && configured); 00567 00568 00569 return true; 00570 } 00571 00572 bool USBJoystick::joystick(unsigned char stick, unsigned short buttons, signed char x, signed char y, signed char z, signed char rz) 00573 { 00574 unsigned char report[8+8+3] = {0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0}; 00575 00576 unsigned char hatswitch; 00577 if (stick & JOYSTICK_UP) { 00578 hatswitch = 0; 00579 if (stick & JOYSTICK_RIGHT) hatswitch = 1; 00580 if (stick & JOYSTICK_LEFT) hatswitch = 7; 00581 } else if (stick & JOYSTICK_RIGHT) { 00582 hatswitch = 2; 00583 if (stick & JOYSTICK_DOWN) hatswitch = 3; 00584 } else if (stick & JOYSTICK_DOWN) { 00585 hatswitch = 4; 00586 if (stick & JOYSTICK_LEFT) hatswitch = 5; 00587 } else if (stick & JOYSTICK_LEFT) { 00588 hatswitch = 6; 00589 } else { 00590 hatswitch = 0xf; 00591 } 00592 00593 report[0] = buttons & 0xff; //buttons 1-8 00594 report[1] = ( buttons >> 8 ) & 0xff;//buttons 9-13 00595 report[2] = ((hatswitch ) & 0x0f); // d-pad 00596 report[3] = x + 0x80; //sticks 00597 report[4] = y + 0x80; 00598 report[5] = z + 0x80; 00599 report[6] = rz + 0x80; 00600 00601 report[7] = 0x00; //unknown 00602 report[8] = 0x00; //unknown 00603 report[9] = 0x00; //unknown 00604 report[10] = 0x00; //unknown 00605 00606 report[11] = 0x00; //button pressures 00607 report[12] = 0x00; //button pressures 00608 report[13] = 0x00; //button pressures 00609 report[14] = 0x00; //button pressures 00610 00611 report[15] = 0x00; //trigger pressures 00612 report[16] = 0x00; //trigger pressures 00613 report[17] = 0x00; //trigger pressures 00614 report[18] = 0x00; //trigger pressures 00615 00616 if (!sendInputReport(report, 19)) { 00617 return false; 00618 } 00619 00620 00621 return true; 00622 } 00623 00624 bool USBJoystick::requestSetup(void) 00625 { 00626 /* Process class requests */ 00627 bool success = false; 00628 00629 if (transfer.setup.bmRequestType.Type == CLASS_TYPE) 00630 { 00631 switch (transfer.setup.bRequest) 00632 { 00633 case GET_REPORT: 00634 switch (transfer.setup.wValue & 0xffff) 00635 { 00636 case 0x0300: //vendor specific request 00637 00638 transfer.ptr = (unsigned char*)magic_init_bytes; 00639 transfer.remaining = sizeof(magic_init_bytes); 00640 transfer.direction = DEVICE_TO_HOST; 00641 success = true; 00642 00643 break; 00644 default: 00645 break; 00646 } 00647 break; 00648 default: 00649 break; 00650 } 00651 } 00652 00653 if (success) 00654 { 00655 /* We've handled this request */ 00656 return true; 00657 } 00658 00659 return usbdevice::requestSetup(); 00660 }
Generated on Tue Jul 12 2022 21:25:10 by
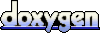