GUI parts for DISCO-F469I a fork of DISCO-F746NG. GuiBase, Button, ButtonGroup, ResetButton, Label, BlinkLabel, NumericLabel, SeekBar, SeekbarGroup
Dependents: DISCO-F469NI_LCDTS_GUI_demo DISCO-F469NI_LCDTS_GUI_demo projekt_PSW_v1 DISCO-F469NI_LCDTS_GUI_demoaaaaaaaaaxxxx ... more
Fork of F746_GUI by
GuiBase.hpp
00001 //----------------------------------------------------------- 00002 // GuiBase class (abstract base class) ---- Header 00003 // 00004 // 2016/04/10, Copyright (c) 2016 MIKAMI, Naoki 00005 //----------------------------------------------------------- 00006 00007 #ifndef F746_GUI_BASE_HPP 00008 #define F746_GUI_BASE_HPP 00009 00010 #include "mbed.h" 00011 #include <string> 00012 #include "TS_DISCO_F469NI.h" 00013 #include "LCD_DISCO_F469NI.h" 00014 00015 namespace Mikami 00016 { 00017 class GuiBase 00018 { 00019 public: 00020 static LCD_DISCO_F469NI* GetLcdPtr() { return &lcd_; } 00021 static TS_DISCO_F469NI* GetTsPtr() { return &ts_; } 00022 00023 // If panel touched, return true 00024 static bool PanelTouched(); 00025 // Get touch panel state 00026 static TS_StateTypeDef GetTsState() { return state_; } 00027 00028 enum { ENUM_TEXT = 0xFFFFFFFF, ENUM_BACK = 0xFF003538, 00029 ENUM_CREATED = 0xFF0068B7, ENUM_TOUCHED = 0xFF7F7FFF, 00030 ENUM_INACTIVE = 0xD0003538, ENUM_INACTIVE_TEXT = 0xFF808080}; 00031 00032 protected: 00033 static LCD_DISCO_F469NI lcd_; // for LCD display 00034 static TS_DISCO_F469NI ts_; // for touch pannel 00035 00036 static TS_StateTypeDef state_; 00037 static bool multiTouch_; 00038 00039 const uint16_t X_, Y_; 00040 sFONT *const FONTS_; 00041 00042 const uint32_t TEXT_COLOR_; 00043 const uint32_t BACK_COLOR_; 00044 const uint32_t CREATED_COLOR_; 00045 const uint32_t TOUCHED_COLOR_; 00046 const uint32_t INACTIVE_COLOR_; 00047 const uint32_t INACTIVE_TEXT_COLOR_; 00048 00049 // Constructor 00050 GuiBase(uint16_t x =0, uint16_t y =0, 00051 sFONT &fonts = Font12, 00052 uint32_t textColor = GuiBase::ENUM_TEXT, 00053 uint32_t backColor = GuiBase::ENUM_BACK, 00054 uint32_t createdColor = GuiBase::ENUM_CREATED, 00055 uint32_t touchedColor = GuiBase::ENUM_TOUCHED, 00056 uint32_t inactiveColor = GuiBase::ENUM_INACTIVE, 00057 uint32_t inactiveTextColor = GuiBase::ENUM_INACTIVE_TEXT); 00058 00059 void DrawString(uint16_t x, uint16_t y, const string str) 00060 { lcd_.DisplayStringAt(x, y, (uint8_t *)str.c_str(), LEFT_MODE); } 00061 00062 private: 00063 static bool first_; 00064 00065 // disallow copy constructor and assignment operator 00066 GuiBase(const GuiBase&); 00067 GuiBase& operator=(const GuiBase&); 00068 }; 00069 } 00070 #endif // F746_GUI_BASE_HPP
Generated on Thu Jul 14 2022 15:35:42 by
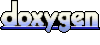