
test
Embed:
(wiki syntax)
Show/hide line numbers
DRV8825.h
00001 ///////////설정값////////////////////// 00002 #define Pulley_radius 7 //풀리의 반지름 00003 #define MOTOR_STEP_ANGLE 1.8 //스텝각 00004 #define MICRO_STEP_DIV 2 // 마이크로스텝 분할수 00005 #define STEP_CYCLE 1.25//스텝주기, 단위 : ms (스텝모터의 속도 설정) 00006 ///////////설정값////////////////////// 00007 00008 #define DESIRED_STEPS_PER_SEC (1000/STEP_CYCLE) //1000ms / 20ms(1step주기) ,50, 초당 스텝수 00009 #define MOTOR_STEPS_PER_REV 360/MOTOR_STEP_ANGLE // 모터 1회전 스텝수 00010 #define MICRO_STEP_PER_REV (MOTOR_STEPS_PER_REV*MICRO_STEP_DIV)//1회전당 총 마이크로 스텝수 00011 #define DESIRED_MICRO_STEPS_PER_SEC (MICRO_STEP_DIV*DESIRED_STEPS_PER_SEC) // 1600 , 초당 마이크로 스텝수 00012 #define SAMPLE_TIME (1000000L/DESIRED_MICRO_STEPS_PER_SEC) //625 , 샘플타임 00013 00014 class DRV8825 00015 { 00016 00017 BusOut _bus; 00018 Timer tmr; 00019 00020 private: 00021 void step(); 00022 int Calculation(double distance); 00023 00024 int32_t _steps; 00025 enum {CW=0,CCW=1} _dir; 00026 int InputStep; 00027 bool start; 00028 double radian, degree; 00029 int micro_step; 00030 00031 00032 public: 00033 DRV8825(PinName pin1, PinName pin2); 00034 void Move(double distance); 00035 }; 00036 00037 ////////////////////////////////////Private//////////////////////////////////// 00038 void DRV8825::step() 00039 { 00040 _bus= _dir ? 0b11:0b01; //_dir이 1면 11반환 , _dir이 거짓이면 01반환 00041 wait_us(1); //minimum pulse width 00042 _bus= _dir ? 0b10:0b00; 00043 _steps+= _dir? -1: 1; // updating current steps 00044 } 00045 00046 int DRV8825::Calculation(double distance) 00047 { 00048 radian=0, degree = 0; 00049 radian = distance / Pulley_radius; //theta값을 구한다 00050 //pc.printf("radian= %f\n", radian); 00051 degree = radian * (180/ 3.141592); //회전할 각도를 구한다 00052 //pc.printf("degree= %f\n", degree); 00053 micro_step = int(degree / (MOTOR_STEP_ANGLE / MICRO_STEP_DIV)); //돌아가야할 마이크로스텝수를 구한다. 00054 00055 return micro_step; 00056 } 00057 00058 00059 ////////////////////////////////////Public//////////////////////////////////// 00060 DRV8825::DRV8825(PinName pin1, PinName pin2): _bus(pin1,pin2) 00061 { 00062 _dir =CW; 00063 _steps=0; 00064 } 00065 00066 00067 void DRV8825::Move(double distance) 00068 { 00069 00070 InputStep = Calculation(distance); 00071 start = true; //회전 시작 flag 00072 00073 if(InputStep>0) _dir = CW; 00074 else if(InputStep<0) _dir = CCW; 00075 tmr.start(); 00076 while(start) { 00077 if(tmr.read_us()>SAMPLE_TIME) { 00078 tmr.reset(); 00079 step(); //1step 이동 00080 00081 00082 if(_steps*_steps >= InputStep*InputStep) { //특정 스텝수만큼 이동하면 stop 00083 start = false; 00084 _steps = 0; 00085 } 00086 } 00087 } 00088 };
Generated on Thu Aug 4 2022 08:57:10 by
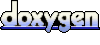