fix the problem http request with '\0' and fixx query string in url
Fork of mbed-http by
http_response_parser.h
00001 /* 00002 * PackageLicenseDeclared: Apache-2.0 00003 * Copyright (c) 2017 ARM Limited 00004 * 00005 * Licensed under the Apache License, Version 2.0 (the "License"); 00006 * you may not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * http://www.apache.org/licenses/LICENSE-2.0 00010 * 00011 * Unless required by applicable law or agreed to in writing, software 00012 * distributed under the License is distributed on an "AS IS" BASIS, 00013 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 * See the License for the specific language governing permissions and 00015 * limitations under the License. 00016 */ 00017 00018 #ifndef _HTTP_RESPONSE_PARSER_H_ 00019 #define _HTTP_RESPONSE_PARSER_H_ 00020 00021 #include "http_parser.h" 00022 #include "http_response.h" 00023 00024 class HttpResponseParser { 00025 public: 00026 HttpResponseParser(HttpResponse* a_response, Callback<void(const char *at, size_t length)> a_body_callback = 0) 00027 : response(a_response), body_callback(a_body_callback) 00028 { 00029 settings = new http_parser_settings(); 00030 00031 settings->on_message_begin = &HttpResponseParser::on_message_begin_callback; 00032 settings->on_url = &HttpResponseParser::on_url_callback; 00033 settings->on_status = &HttpResponseParser::on_status_callback; 00034 settings->on_header_field = &HttpResponseParser::on_header_field_callback; 00035 settings->on_header_value = &HttpResponseParser::on_header_value_callback; 00036 settings->on_headers_complete = &HttpResponseParser::on_headers_complete_callback; 00037 settings->on_chunk_header = &HttpResponseParser::on_chunk_header_callback; 00038 settings->on_chunk_complete = &HttpResponseParser::on_chunk_complete_callback; 00039 settings->on_body = &HttpResponseParser::on_body_callback; 00040 settings->on_message_complete = &HttpResponseParser::on_message_complete_callback; 00041 00042 // Construct the http_parser object 00043 parser = new http_parser(); 00044 http_parser_init(parser, HTTP_RESPONSE); 00045 parser->data = (void*)this; 00046 } 00047 00048 ~HttpResponseParser() { 00049 if (parser) { 00050 delete parser; 00051 } 00052 if (settings) { 00053 delete settings; 00054 } 00055 } 00056 00057 size_t execute(const char* buffer, size_t buffer_size) { 00058 return http_parser_execute(parser, settings, buffer, buffer_size); 00059 } 00060 00061 void finish() { 00062 http_parser_execute(parser, settings, NULL, 0); 00063 } 00064 00065 private: 00066 // Member functions 00067 int on_message_begin(http_parser* parser) { 00068 return 0; 00069 } 00070 00071 int on_url(http_parser* parser, const char *at, size_t length) { 00072 return 0; 00073 } 00074 00075 int on_status(http_parser* parser, const char *at, size_t length) { 00076 string s(at, length); 00077 response->set_status(parser->status_code, s); 00078 return 0; 00079 } 00080 00081 int on_header_field(http_parser* parser, const char *at, size_t length) { 00082 string s(at, length); 00083 response->set_header_field(s); 00084 return 0; 00085 } 00086 00087 int on_header_value(http_parser* parser, const char *at, size_t length) { 00088 string s(at, length); 00089 response->set_header_value(s); 00090 return 0; 00091 } 00092 00093 int on_headers_complete(http_parser* parser) { 00094 response->set_headers_complete(); 00095 return 0; 00096 } 00097 00098 int on_body(http_parser* parser, const char *at, size_t length) { 00099 response->increase_body_length(length); 00100 00101 if (body_callback) { 00102 body_callback(at, length); 00103 return 0; 00104 } 00105 00106 response->set_body(at, length); 00107 return 0; 00108 } 00109 00110 int on_message_complete(http_parser* parser) { 00111 response->set_message_complete(); 00112 00113 return 0; 00114 } 00115 00116 int on_chunk_header(http_parser* parser) { 00117 response->set_chunked(); 00118 00119 return 0; 00120 } 00121 00122 int on_chunk_complete(http_parser* parser) { 00123 return 0; 00124 } 00125 00126 // Static http_parser callback functions 00127 static int on_message_begin_callback(http_parser* parser) { 00128 return ((HttpResponseParser*)parser->data)->on_message_begin(parser); 00129 } 00130 00131 static int on_url_callback(http_parser* parser, const char *at, size_t length) { 00132 return ((HttpResponseParser*)parser->data)->on_url(parser, at, length); 00133 } 00134 00135 static int on_status_callback(http_parser* parser, const char *at, size_t length) { 00136 return ((HttpResponseParser*)parser->data)->on_status(parser, at, length); 00137 } 00138 00139 static int on_header_field_callback(http_parser* parser, const char *at, size_t length) { 00140 return ((HttpResponseParser*)parser->data)->on_header_field(parser, at, length); 00141 } 00142 00143 static int on_header_value_callback(http_parser* parser, const char *at, size_t length) { 00144 return ((HttpResponseParser*)parser->data)->on_header_value(parser, at, length); 00145 } 00146 00147 static int on_headers_complete_callback(http_parser* parser) { 00148 return ((HttpResponseParser*)parser->data)->on_headers_complete(parser); 00149 } 00150 00151 static int on_body_callback(http_parser* parser, const char *at, size_t length) { 00152 return ((HttpResponseParser*)parser->data)->on_body(parser, at, length); 00153 } 00154 00155 static int on_message_complete_callback(http_parser* parser) { 00156 return ((HttpResponseParser*)parser->data)->on_message_complete(parser); 00157 } 00158 00159 static int on_chunk_header_callback(http_parser* parser) { 00160 return ((HttpResponseParser*)parser->data)->on_chunk_header(parser); 00161 } 00162 00163 static int on_chunk_complete_callback(http_parser* parser) { 00164 return ((HttpResponseParser*)parser->data)->on_chunk_complete(parser); 00165 } 00166 00167 HttpResponse* response; 00168 Callback<void(const char *at, size_t length)> body_callback; 00169 http_parser* parser; 00170 http_parser_settings* settings; 00171 }; 00172 00173 #endif // _HTTP_RESPONSE_PARSER_H_
Generated on Fri Jul 22 2022 08:07:18 by
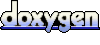