Library DS2782 Stand-Alone Fuel Gauge IC
Embed:
(wiki syntax)
Show/hide line numbers
ds2782.h
00001 /* 00002 @file ds2782.h 00003 00004 @brief DS2782 Stand-Alone Fuel Gauge IC 00005 Breakout I2C Library 00006 00007 @Author lukasz uszko(luszko@op.pl) 00008 00009 Tested on FRDM-KL46Z and FRDM-KL25Z 00010 00011 Copyright (c) 2014 lukasz uszko 00012 Released under the MIT License (see http://mbed.org/license/mit) 00013 00014 Documentation regarding the MAX9611 might be found here: 00015 http://www.maximintegrated.com/en/products/power/battery-management/DS2782.html 00016 00017 and some very useful tutorials: 00018 http://www.maximintegrated.com/en/products/power/battery-management/DS2782.html/tb_tab2 00019 http://www.maximintegrated.com/en/app-notes/index.mvp/id/3584 00020 http://www.maximintegrated.com/en/app-notes/index.mvp/id/3463 00021 00022 */ 00023 00024 00025 #ifndef DS2782_H 00026 #define DS2782_H 00027 00028 #include "mbed.h" 00029 00030 #define DS2782_I2C_ADDRESS 0x34<<1 00031 00032 00033 00034 00035 00036 00037 class DS2782{ 00038 00039 00040 /**********private members and methods********************************/ 00041 private: 00042 00043 typedef enum { 00044 STATUS=0x01, /*STATUS - Status Register*/ 00045 RAAC_MSB_REG, /*RAAC - Remaining Active Absolute Capacity MSB*/ 00046 RAAC_LSB_REG, 00047 RSAC_MSB_REG, /*RSAC - Remaining Standby Absolute Capacity MSB*/ 00048 RSAC_LSB_REG, 00049 RARC_REG, /*RARC - Remaining Active Relative Capacity*/ 00050 RSRC_REG, /*RSRC - Remaining Standby Relative Capacity*/ 00051 IAVG_MSB_REG, /*IAVG - Average Current Register MSB*/ 00052 IAVG_LSB_REG, 00053 TEMP_MSB_REG, /*TEMP - Temperature Register MSB*/ 00054 TEMP_LSB_REG, 00055 VOLT_MSB_REG, /*VOLT - Voltage Register MSB*/ 00056 VOLT_LSB_REG, 00057 CURRENT_MSB_REG, /*CURRENT - Current Register MSB*/ 00058 CURRENT_LSB_REG, 00059 ACR_MSB_REG, /*ACR - Accumulated Current Register MSB*/ 00060 ACR_LSB_REG, 00061 ACRL_MSB_REG, /*Low Accumulated Current Register MSB*/ 00062 ACRL_LSB_REG, 00063 AS_REG, /*AS - Age Scalar*/ 00064 SFR_REG, /*SFR - Special Feature Register*/ 00065 FULL_MSB_REG, /*FULL - Full Capacity MSB*/ 00066 FULL_LSB_REG, 00067 AE_MSB_REG, /*AE - Active Empty MSB*/ 00068 AE_LSB_REG, 00069 SE_MSB_REG, /*SE - Standby Empty MSB*/ 00070 SE_LSB_REG, 00071 EEPROM_REG= 0x1F, /*EEPROM - EEPROM Register */ 00072 USR_EEPROM_REG= 0x20, /*User EEPROM, Lockable, Block 0 [20 to 2F]*/ 00073 ADD_USR_EEPROM_REG=0x30, /*Additional User EEPROM, Lockable, Block 0 [30 to 37]*/ 00074 PARAM_EEPROM_REG=0x60, /*Parameter EEPROM, Lockable, Block 1 [60 to 7F]*/ 00075 UNIQUE_ID_REG =0xF0, /*Unique ID [F0 to F7]*/ 00076 FUNC_COMMAND_REG= 0xFE /*Function Command Register */ 00077 00078 }RegAddr; 00079 00080 typedef enum { 00081 00082 CONTROL = 0x60, //Control Register 00083 AB =0x61, //Accumulation Bias 00084 AC_MSB = 0x62, //Aging Capacity MSB 00085 AC_LSB = 0x63, //Aging Capacity LSB 00086 VCHG = 0x64, //Charge Voltage 00087 IMIN =0x65, //Minimum Charge Current 00088 VAE = 0x66, //Active Empty Voltage 00089 IAE = 0x67, //Active Empty Current 00090 ACTIVE_EMPTY_40, 00091 RSNSP, //Sense Resistor Prime 00092 FULL_40_MSB, 00093 FULL_40_LSB, 00094 FULL_3040_SLOPE, 00095 FULL_2030_SLOPE, 00096 FULL_1020_SLOPE, 00097 FULL_0010_SLOPE, 00098 AE_3040_SLOPE, 00099 AE_2030_SLOPE, 00100 AE_1020_SLOPE, 00101 AE_0010_SLOPE, 00102 SE_3040_SLOPE, 00103 SE_2030_SLOPE, 00104 SE_1020_SLOPE, 00105 SE_0010_SLOPE, 00106 RSGAIN_MSB, //Sense Resistor Gain MSB 00107 RSGAIN_LSB, //Sense Resistor Gain LSB 00108 RSTC, //Sense Resistor Temp. Coeff. 00109 FRSGAIN_MSB, //Factory Gain MSB 00110 FRSGAIN_LSB, //Factory Gain LSB 00111 I2C_SLAVE_ADDR= 0x7E //2-Wire Slave Address 00112 }ParamEepromReg; 00113 00114 00115 00116 00117 00118 00119 00120 /** Write data to the given register 00121 * 00122 * @returns 00123 * 1 on success, 00124 * 0 on error 00125 */ 00126 bool write(uint8_t regAddress, uint8_t* data,int dataLength); 00127 00128 /** Write data to the given register 00129 * @param register Address 00130 * @param data to read 00131 * @param length of data to read 00132 * @returns 00133 * 1 on success, 00134 * 0 on error 00135 */ 00136 bool read(uint8_t regAddress, uint8_t* data,int length); 00137 00138 /** merge two bytes in one word 00139 * @param 1st byte 00140 * @param 2nd byte 00141 * @returns 16 bit word 00142 */ 00143 inline uint16_t get16BitData(uint8_t msbByte,uint8_t lsbByte){ 00144 uint16_t data16Bit= (msbByte<<8)|(lsbByte); 00145 return data16Bit; 00146 } 00147 00148 /** divide 16 bit word to 2 8bit bytes 00149 * @param 1st byte 00150 * @param buf 00151 */ 00152 inline void fillBuf(uint16_t varVal, uint8_t* buf){ 00153 buf[0]= ((varVal>>8)&0xFF); 00154 buf[1]= ((varVal)&0xFF); 00155 } 00156 00157 I2C mI2c; 00158 uint8_t mI2cAddr; 00159 00160 00161 /**********protected members and methods********************************/ 00162 protected: 00163 float mTemperature; 00164 float mCurrent; 00165 float mVoltage; 00166 00167 /**********public methods********************************/ 00168 public: 00169 00170 typedef enum { 00171 PORF = 0x02, //Power-On Reset Flag – Useful for reset detection, see text below. 00172 UVF =0x04, //Under-Voltage Flag 00173 LEARNF = 0x10, //Learn Flag – When set to 1, a charge cycle can be used to learn battery capacity. 00174 SEF = 0x20, //Standby Empty Flag 00175 AEF = 0x40, //Active Empty Flag 00176 CHGTF =0x80, //Charge Termination Flag 00177 }StatusReg ; 00178 00179 /** Create an SI7020 instance 00180 * @param sda pin 00181 * @param scl pin 00182 * @param address: I2C slave address 00183 */ 00184 DS2782(PinName sda, PinName scl,int i2cFrequencyHz=100000,uint8_t address = DS2782_I2C_ADDRESS); 00185 00186 00187 /** Initialization: set member values and configuration registers, ought to be invoked in the body of constructor 00188 * @returns 00189 * true on success, 00190 * false on error 00191 */ 00192 bool initDS2782(void); 00193 00194 /** Read temperature from the sensor. 00195 * @param none 00196 * @returns 00197 * 1 on success, 00198 * 0 on error 00199 */ 00200 bool readTemperature(void); 00201 bool readCurrent(void); 00202 bool readVoltage(void); 00203 bool setACRRegister(uint16_t reg); // set to 0 clears LEARNF and other flags 00204 bool setEepromBlockRegister(ParamEepromReg reg, uint8_t * value, uint8_t length); 00205 uint8_t readStatusReg(void); 00206 float readAcrReg(void); 00207 uint8_t readRarcReg(void); 00208 00209 00210 // setters-getters 00211 float getTemperature(void); 00212 float getCurrent(void); 00213 float getVoltage(void); 00214 00215 }; 00216 00217 #endif
Generated on Wed Aug 17 2022 10:58:41 by
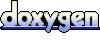