
/** 03_pwm_servo * This program moves the servo back and forth * within the full range of pulse width = 1.0 - 2.0 ms. * * Hardware requirements: * - FRDM-KL25Z board * - Servo connected to D3, +5V and GND */
main.cpp
00001 /** 03_pwm_servo 00002 * This program moves the servo back and forth 00003 * within the full range of pulse width = 1.0 - 2.0 ms. 00004 * 00005 * Hardware requirements: 00006 * - FRDM-KL25Z board 00007 * - Servo connected to D3, +5V and GND 00008 */ 00009 00010 #include "mbed.h" 00011 PwmOut servo(D3); 00012 00013 int main() { 00014 servo.period_ms(20); //Period = 20 ms (f=50 Hz) 00015 while(true) { 00016 for(int pw=1000; pw <= 2000; pw=pw+20) { 00017 servo.pulsewidth_us(pw); //Set new servo position 00018 wait_ms(200); 00019 } 00020 wait_ms(1000); //Wait before reverse direction 00021 for(int pw=2000; pw >= 1000; pw=pw-20) { 00022 servo.pulsewidth_us(pw); //Set new servo position 00023 wait_ms(200); 00024 } 00025 wait_ms(1000); 00026 } 00027 }
Generated on Thu Aug 4 2022 10:56:37 by
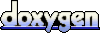