
Sample MQTT-SN program
Dependencies: C12832 MQTTSN mbed-rtos mbed
main.cpp
00001 /******************************************************************************* 00002 * Copyright (c) 2014, 2015 IBM Corp. 00003 * 00004 * All rights reserved. This program and the accompanying materials 00005 * are made available under the terms of the Eclipse Public License v1.0 00006 * and Eclipse Distribution License v1.0 which accompany this distribution. 00007 * 00008 * The Eclipse Public License is available at 00009 * http://www.eclipse.org/legal/epl-v10.html 00010 * and the Eclipse Distribution License is available at 00011 * http://www.eclipse.org/org/documents/edl-v10.php. 00012 * 00013 * Contributors: 00014 * Ian Craggs - initial API and implementation and/or initial documentation 00015 *******************************************************************************/ 00016 00017 /** 00018 This is a sample program to illustrate the use of the MQTTSN Client library 00019 on the mbed platform. The Client class requires two classes which mediate 00020 access to system interfaces for networking and timing. As long as these two 00021 classes provide the required public programming interfaces, it does not matter 00022 what facilities they use underneath. In this program, they use the mbed 00023 system libraries. 00024 00025 */ 00026 00027 #define WARN printf 00028 00029 #include "EthernetInterface.h" 00030 #include "C12832.h" 00031 00032 #if defined(TARGET_LPC1768) 00033 #warning "Compiling for mbed LPC1768" 00034 #include "LPC1768.h" 00035 #elif defined(TARGET_K64F) 00036 #warning "Compiling for mbed K64F" 00037 #include "K64F.h" 00038 #endif 00039 00040 #define MQTTSNCLIENT_QOS2 1 00041 #include "MQTTSNUDP.h" 00042 #include "MQTTSNClient.h" 00043 00044 int arrivedcount = 0; 00045 00046 00047 void off() 00048 { 00049 r = g = b = 1.0; // 1 is off, 0 is full brightness 00050 } 00051 00052 void red() 00053 { 00054 r = 0.7; g = 1.0; b = 1.0; // 1 is off, 0 is full brightness 00055 } 00056 00057 void yellow() 00058 { 00059 r = 0.7; g = 0.7; b = 1.0; // 1 is off, 0 is full brightness 00060 } 00061 00062 void green() 00063 { 00064 r = 1.0; g = 0.7; b = 1.0; // 1 is off, 0 is full brightness 00065 } 00066 00067 00068 void messageArrived(MQTTSN::MessageData& md) 00069 { 00070 MQTTSN::Message &message = md.message; 00071 lcd.cls(); 00072 lcd.locate(0,3); 00073 printf("Message arrived: qos %d, retained %d, dup %d, packetid %d\n", message.qos, message.retained, message.dup, message.id); 00074 printf("Payload %.*s\n", message.payloadlen, (char*)message.payload); 00075 ++arrivedcount; 00076 lcd.puts((char*)message.payload); 00077 } 00078 00079 00080 int main(int argc, char* argv[]) 00081 { 00082 MQTTSNUDP ipstack = MQTTSNUDP(); 00083 float version = 0.47; 00084 char* topic = "mbed-sample"; 00085 00086 lcd.printf("Version is %f\n", version); 00087 printf("Version is %f\n", version); 00088 00089 MQTTSN::Client<MQTTSNUDP, Countdown> client = MQTTSN::Client<MQTTSNUDP, Countdown>(ipstack); 00090 00091 EthernetInterface eth; 00092 eth.init(); // Use DHCP 00093 eth.connect(); 00094 00095 char* hostname = "9.20.230.78"; 00096 int port = 20000; 00097 lcd.printf("Connecting to %s:%d\n", hostname, port); 00098 int rc = ipstack.connect(hostname, port); 00099 if (rc != 0) 00100 lcd.printf("rc from TCP connect is %d\n", rc); 00101 else 00102 green(); 00103 00104 MQTTSNPacket_connectData data = MQTTSNPacket_connectData_initializer; 00105 data.clientID.cstring = "mbed-sample"; 00106 data.duration = 60; 00107 if ((rc = client.connect(data)) != 0) 00108 lcd.printf("rc from MQTT connect is %d\n", rc); 00109 00110 MQTTSN_topicid topicid; 00111 topicid.type = MQTTSN_TOPIC_TYPE_NORMAL; 00112 topicid.data.long_.name = topic; 00113 topicid.data.long_.len = strlen(topic); 00114 MQTTSN::QoS grantedQoS; 00115 if ((rc = client.subscribe(topicid, MQTTSN::QOS1, grantedQoS, messageArrived)) != 0) 00116 lcd.printf("rc from MQTT subscribe is %d\n", rc); 00117 00118 MQTTSN::Message message; 00119 00120 // QoS 0 00121 char buf[100]; 00122 sprintf(buf, "Hello World! QoS 0 message from app version %f\n", version); 00123 message.qos = MQTTSN::QOS0; 00124 message.retained = false; 00125 message.dup = false; 00126 message.payload = (void*)buf; 00127 message.payloadlen = strlen(buf)+1; 00128 rc = client.publish(topicid, message); 00129 while (arrivedcount < 1) 00130 client.yield(100); 00131 00132 // QoS 1 00133 sprintf(buf, "Hello World! QoS 1 message from app version %f\n", version); 00134 message.qos = MQTTSN::QOS1; 00135 message.payloadlen = strlen(buf)+1; 00136 rc = client.publish(topicid, message); 00137 while (arrivedcount < 2) 00138 client.yield(100); 00139 00140 // QoS 2 00141 sprintf(buf, "Hello World! QoS 2 message from app version %f\n", version); 00142 message.qos = MQTTSN::QOS2; 00143 message.payloadlen = strlen(buf)+1; 00144 rc = client.publish(topicid, message); 00145 while (arrivedcount < 3) 00146 client.yield(100); 00147 00148 // n * QoS 2 00149 for (int i = 1; i <= 10; ++i) 00150 { 00151 sprintf(buf, "Hello World! QoS 2 message number %d from app version %f\n", i, version); 00152 message.qos = MQTTSN::QOS2; 00153 message.payloadlen = strlen(buf)+1; 00154 rc = client.publish(topicid, message); 00155 while (arrivedcount < i + 3) 00156 client.yield(100); 00157 } 00158 00159 if ((rc = client.unsubscribe(topicid)) != 0) 00160 printf("rc from unsubscribe was %d\n", rc); 00161 00162 if ((rc = client.disconnect()) != 0) 00163 printf("rc from disconnect was %d\n", rc); 00164 00165 ipstack.disconnect(); 00166 00167 lcd.cls(); 00168 lcd.locate(0,3); 00169 lcd.printf("Version %.2f: finish %d msgs\n", version, arrivedcount); 00170 printf("Finishing with %d messages received\n", arrivedcount); 00171 00172 return 0; 00173 }
Generated on Wed Jul 13 2022 08:22:23 by
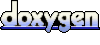