Mbed port of Stm32f4Cube LCD LOG for STM32F429I-DISCO
Dependents: DISCO-F429ZI_ExportTemplate1
lcd_log.c
00001 /** 00002 ****************************************************************************** 00003 * @file lcd_log.c 00004 * @author MCD Application Team 00005 * @version V1.0.0 00006 * @date 18-February-2014 00007 * @brief This file provides all the LCD Log firmware functions. 00008 * 00009 * The LCD Log module allows to automatically set a header and footer 00010 * on any application using the LCD display and allows to dump user, 00011 * debug and error messages by using the following macros: LCD_ErrLog(), 00012 * LCD_UsrLog() and LCD_DbgLog(). 00013 * 00014 * It supports also the scroll feature by embedding an internal software 00015 * cache for display. This feature allows to dump message sequentially 00016 * on the display even if the number of displayed lines is bigger than 00017 * the total number of line allowed by the display. 00018 * 00019 ****************************************************************************** 00020 * @attention 00021 * 00022 * <h2><center>© COPYRIGHT(c) 2014 STMicroelectronics</center></h2> 00023 * 00024 * Redistribution and use in source and binary forms, with or without modification, 00025 * are permitted provided that the following conditions are met: 00026 * 1. Redistributions of source code must retain the above copyright notice, 00027 * this list of conditions and the following disclaimer. 00028 * 2. Redistributions in binary form must reproduce the above copyright notice, 00029 * this list of conditions and the following disclaimer in the documentation 00030 * and/or other materials provided with the distribution. 00031 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00032 * may be used to endorse or promote products derived from this software 00033 * without specific prior written permission. 00034 * 00035 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00036 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00037 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00038 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00039 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00040 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00041 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00042 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00043 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00044 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00045 * 00046 ****************************************************************************** 00047 */ 00048 00049 /* Includes ------------------------------------------------------------------*/ 00050 #include <stdio.h> 00051 #include "lcd_log.h" 00052 00053 /** @addtogroup Utilities 00054 * @{ 00055 */ 00056 00057 /** @addtogroup STM32_EVAL 00058 * @{ 00059 */ 00060 00061 /** @addtogroup Common 00062 * @{ 00063 */ 00064 00065 /** @defgroup LCD_LOG 00066 * @brief LCD Log LCD_Application module 00067 * @{ 00068 */ 00069 00070 /** @defgroup LCD_LOG_Private_Types 00071 * @{ 00072 */ 00073 /** 00074 * @} 00075 */ 00076 00077 00078 /** @defgroup LCD_LOG_Private_Defines 00079 * @{ 00080 */ 00081 00082 /** 00083 * @} 00084 */ 00085 00086 /* Define the display window settings */ 00087 #define YWINDOW_MIN 2 00088 00089 /** @defgroup LCD_LOG_Private_Macros 00090 * @{ 00091 */ 00092 /** 00093 * @} 00094 */ 00095 00096 00097 /** @defgroup LCD_LOG_Private_Variables 00098 * @{ 00099 */ 00100 00101 LCD_LOG_line LCD_CacheBuffer [LCD_CACHE_DEPTH]; 00102 uint32_t LCD_LineColor; 00103 uint16_t LCD_CacheBuffer_xptr; 00104 uint16_t LCD_CacheBuffer_yptr_top; 00105 uint16_t LCD_CacheBuffer_yptr_bottom; 00106 00107 uint16_t LCD_CacheBuffer_yptr_top_bak; 00108 uint16_t LCD_CacheBuffer_yptr_bottom_bak; 00109 00110 FunctionalState LCD_CacheBuffer_yptr_invert; 00111 FunctionalState LCD_ScrollActive; 00112 FunctionalState LCD_Lock; 00113 FunctionalState LCD_Scrolled; 00114 uint16_t LCD_ScrollBackStep; 00115 00116 /** 00117 * @} 00118 */ 00119 00120 00121 /** @defgroup LCD_LOG_Private_FunctionPrototypes 00122 * @{ 00123 */ 00124 00125 /** 00126 * @} 00127 */ 00128 00129 00130 /** @defgroup LCD_LOG_Private_Functions 00131 * @{ 00132 */ 00133 00134 00135 /** 00136 * @brief Initializes the LCD Log module 00137 * @param None 00138 * @retval None 00139 */ 00140 00141 void LCD_LOG_Init ( void) 00142 { 00143 /* Deinit LCD cache */ 00144 LCD_LOG_DeInit(); 00145 00146 /* Clear the LCD */ 00147 BSP_LCD_Clear(LCD_LOG_BACKGROUND_COLOR); 00148 } 00149 00150 /** 00151 * @brief DeInitializes the LCD Log module. 00152 * @param None 00153 * @retval None 00154 */ 00155 void LCD_LOG_DeInit(void) 00156 { 00157 LCD_LineColor = LCD_LOG_TEXT_COLOR; 00158 LCD_CacheBuffer_xptr = 0; 00159 LCD_CacheBuffer_yptr_top = 0; 00160 LCD_CacheBuffer_yptr_bottom = 0; 00161 00162 LCD_CacheBuffer_yptr_top_bak = 0; 00163 LCD_CacheBuffer_yptr_bottom_bak = 0; 00164 00165 LCD_CacheBuffer_yptr_invert= ENABLE; 00166 LCD_ScrollActive = DISABLE; 00167 LCD_Lock = DISABLE; 00168 LCD_Scrolled = DISABLE; 00169 LCD_ScrollBackStep = 0; 00170 } 00171 00172 /** 00173 * @brief Display the application header on the LCD screen 00174 * @param header: pointer to the string to be displayed 00175 * @retval None 00176 */ 00177 void LCD_LOG_SetHeader (uint8_t *header) 00178 { 00179 /* Set the LCD Font */ 00180 BSP_LCD_SetFont (&LCD_LOG_HEADER_FONT); 00181 00182 BSP_LCD_SetTextColor(LCD_LOG_SOLID_BACKGROUND_COLOR); 00183 BSP_LCD_FillRect(0, 0, BSP_LCD_GetXSize(), LCD_LOG_HEADER_FONT.Height + 8); 00184 00185 /* Set the LCD Text Color */ 00186 BSP_LCD_SetTextColor(LCD_LOG_SOLID_TEXT_COLOR); 00187 BSP_LCD_SetBackColor(LCD_LOG_SOLID_BACKGROUND_COLOR); 00188 00189 BSP_LCD_DisplayStringAt(0, LCD_LOG_HEADER_FONT.Height / 2, header, CENTER_MODE); 00190 00191 BSP_LCD_SetBackColor(LCD_LOG_BACKGROUND_COLOR); 00192 BSP_LCD_SetTextColor(LCD_LOG_TEXT_COLOR); 00193 BSP_LCD_SetFont (&LCD_LOG_TEXT_FONT); 00194 } 00195 00196 /** 00197 * @brief Display the application footer on the LCD screen 00198 * @param footer: pointer to the string to be displayed 00199 * @retval None 00200 */ 00201 void LCD_LOG_SetFooter(uint8_t *footer) 00202 { 00203 /* Set the LCD Font */ 00204 BSP_LCD_SetFont (&LCD_LOG_FOOTER_FONT); 00205 00206 BSP_LCD_SetTextColor(LCD_LOG_SOLID_BACKGROUND_COLOR); 00207 BSP_LCD_FillRect(0, BSP_LCD_GetYSize() - LCD_LOG_FOOTER_FONT.Height - 4, BSP_LCD_GetXSize(), LCD_LOG_FOOTER_FONT.Height + 4); 00208 00209 /* Set the LCD Text Color */ 00210 BSP_LCD_SetTextColor(LCD_LOG_SOLID_TEXT_COLOR); 00211 BSP_LCD_SetBackColor(LCD_LOG_SOLID_BACKGROUND_COLOR); 00212 00213 BSP_LCD_DisplayStringAt(0, BSP_LCD_GetYSize() - LCD_LOG_FOOTER_FONT.Height, footer, CENTER_MODE); 00214 00215 BSP_LCD_SetBackColor(LCD_LOG_BACKGROUND_COLOR); 00216 BSP_LCD_SetTextColor(LCD_LOG_TEXT_COLOR); 00217 BSP_LCD_SetFont (&LCD_LOG_TEXT_FONT); 00218 } 00219 00220 /** 00221 * @brief Clear the Text Zone 00222 * @param None 00223 * @retval None 00224 */ 00225 void LCD_LOG_ClearTextZone(void) 00226 { 00227 uint8_t i=0; 00228 00229 for (i= 0 ; i < YWINDOW_SIZE; i++) 00230 { 00231 BSP_LCD_ClearStringLine(i + YWINDOW_MIN); 00232 } 00233 00234 LCD_LOG_DeInit(); 00235 } 00236 00237 /** 00238 * @brief Redirect the printf to the LCD 00239 * @param c: character to be displayed 00240 * @param f: output file pointer 00241 * @retval None 00242 */ 00243 LCD_LOG_PUTCHAR 00244 { 00245 00246 sFONT *cFont = BSP_LCD_GetFont(); 00247 uint32_t idx; 00248 00249 if(LCD_Lock == DISABLE) 00250 { 00251 if(LCD_ScrollActive == ENABLE) 00252 { 00253 LCD_CacheBuffer_yptr_bottom = LCD_CacheBuffer_yptr_bottom_bak; 00254 LCD_CacheBuffer_yptr_top = LCD_CacheBuffer_yptr_top_bak; 00255 LCD_ScrollActive = DISABLE; 00256 LCD_Scrolled = DISABLE; 00257 LCD_ScrollBackStep = 0; 00258 00259 } 00260 00261 if(( LCD_CacheBuffer_xptr < (BSP_LCD_GetXSize()) /cFont->Width ) && ( ch != '\n')) 00262 { 00263 LCD_CacheBuffer[LCD_CacheBuffer_yptr_bottom].line[LCD_CacheBuffer_xptr++] = (uint16_t)ch; 00264 } 00265 else 00266 { 00267 if(LCD_CacheBuffer_yptr_top >= LCD_CacheBuffer_yptr_bottom) 00268 { 00269 00270 if(LCD_CacheBuffer_yptr_invert == DISABLE) 00271 { 00272 LCD_CacheBuffer_yptr_top++; 00273 00274 if(LCD_CacheBuffer_yptr_top == LCD_CACHE_DEPTH) 00275 { 00276 LCD_CacheBuffer_yptr_top = 0; 00277 } 00278 } 00279 else 00280 { 00281 LCD_CacheBuffer_yptr_invert= DISABLE; 00282 } 00283 } 00284 00285 for(idx = LCD_CacheBuffer_xptr ; idx < (BSP_LCD_GetXSize()) /cFont->Width; idx++) 00286 { 00287 LCD_CacheBuffer[LCD_CacheBuffer_yptr_bottom].line[LCD_CacheBuffer_xptr++] = ' '; 00288 } 00289 LCD_CacheBuffer[LCD_CacheBuffer_yptr_bottom].color = LCD_LineColor; 00290 00291 LCD_CacheBuffer_xptr = 0; 00292 00293 LCD_LOG_UpdateDisplay (); 00294 00295 LCD_CacheBuffer_yptr_bottom ++; 00296 00297 if (LCD_CacheBuffer_yptr_bottom == LCD_CACHE_DEPTH) 00298 { 00299 LCD_CacheBuffer_yptr_bottom = 0; 00300 LCD_CacheBuffer_yptr_top = 1; 00301 LCD_CacheBuffer_yptr_invert = ENABLE; 00302 } 00303 00304 if( ch != '\n') 00305 { 00306 LCD_CacheBuffer[LCD_CacheBuffer_yptr_bottom].line[LCD_CacheBuffer_xptr++] = (uint16_t)ch; 00307 } 00308 00309 } 00310 } 00311 return ch; 00312 } 00313 00314 /** 00315 * @brief Update the text area display 00316 * @param None 00317 * @retval None 00318 */ 00319 void LCD_LOG_UpdateDisplay (void) 00320 { 00321 uint8_t cnt = 0 ; 00322 uint16_t length = 0 ; 00323 uint16_t ptr = 0, index = 0; 00324 00325 if((LCD_CacheBuffer_yptr_bottom < (YWINDOW_SIZE -1)) && 00326 (LCD_CacheBuffer_yptr_bottom >= LCD_CacheBuffer_yptr_top)) 00327 { 00328 BSP_LCD_SetTextColor(LCD_CacheBuffer[cnt + LCD_CacheBuffer_yptr_bottom].color); 00329 BSP_LCD_DisplayStringAtLine ((YWINDOW_MIN + LCD_CacheBuffer_yptr_bottom), 00330 (uint8_t *)(LCD_CacheBuffer[cnt + LCD_CacheBuffer_yptr_bottom].line)); 00331 } 00332 else 00333 { 00334 00335 if(LCD_CacheBuffer_yptr_bottom < LCD_CacheBuffer_yptr_top) 00336 { 00337 /* Virtual length for rolling */ 00338 length = LCD_CACHE_DEPTH + LCD_CacheBuffer_yptr_bottom ; 00339 } 00340 else 00341 { 00342 length = LCD_CacheBuffer_yptr_bottom; 00343 } 00344 00345 ptr = length - YWINDOW_SIZE + 1; 00346 00347 for (cnt = 0 ; cnt < YWINDOW_SIZE ; cnt ++) 00348 { 00349 00350 index = (cnt + ptr )% LCD_CACHE_DEPTH ; 00351 00352 BSP_LCD_SetTextColor(LCD_CacheBuffer[index].color); 00353 BSP_LCD_DisplayStringAtLine ((cnt + YWINDOW_MIN), 00354 (uint8_t *)(LCD_CacheBuffer[index].line)); 00355 00356 } 00357 } 00358 00359 } 00360 00361 #if( LCD_SCROLL_ENABLED == 1) 00362 /** 00363 * @brief Display previous text frame 00364 * @param None 00365 * @retval Status 00366 */ 00367 ErrorStatus LCD_LOG_ScrollBack(void) 00368 { 00369 00370 if(LCD_ScrollActive == DISABLE) 00371 { 00372 00373 LCD_CacheBuffer_yptr_bottom_bak = LCD_CacheBuffer_yptr_bottom; 00374 LCD_CacheBuffer_yptr_top_bak = LCD_CacheBuffer_yptr_top; 00375 00376 00377 if(LCD_CacheBuffer_yptr_bottom > LCD_CacheBuffer_yptr_top) 00378 { 00379 00380 if ((LCD_CacheBuffer_yptr_bottom - LCD_CacheBuffer_yptr_top) <= YWINDOW_SIZE) 00381 { 00382 LCD_Lock = DISABLE; 00383 return ERROR; 00384 } 00385 } 00386 LCD_ScrollActive = ENABLE; 00387 00388 if((LCD_CacheBuffer_yptr_bottom > LCD_CacheBuffer_yptr_top)&& 00389 (LCD_Scrolled == DISABLE )) 00390 { 00391 LCD_CacheBuffer_yptr_bottom--; 00392 LCD_Scrolled = ENABLE; 00393 } 00394 00395 } 00396 00397 if(LCD_ScrollActive == ENABLE) 00398 { 00399 LCD_Lock = ENABLE; 00400 00401 if(LCD_CacheBuffer_yptr_bottom > LCD_CacheBuffer_yptr_top) 00402 { 00403 00404 if((LCD_CacheBuffer_yptr_bottom - LCD_CacheBuffer_yptr_top) < YWINDOW_SIZE ) 00405 { 00406 LCD_Lock = DISABLE; 00407 return ERROR; 00408 } 00409 00410 LCD_CacheBuffer_yptr_bottom --; 00411 } 00412 else if(LCD_CacheBuffer_yptr_bottom <= LCD_CacheBuffer_yptr_top) 00413 { 00414 00415 if((LCD_CACHE_DEPTH - LCD_CacheBuffer_yptr_top + LCD_CacheBuffer_yptr_bottom) < YWINDOW_SIZE) 00416 { 00417 LCD_Lock = DISABLE; 00418 return ERROR; 00419 } 00420 LCD_CacheBuffer_yptr_bottom --; 00421 00422 if(LCD_CacheBuffer_yptr_bottom == 0xFFFF) 00423 { 00424 LCD_CacheBuffer_yptr_bottom = LCD_CACHE_DEPTH - 2; 00425 } 00426 } 00427 LCD_ScrollBackStep++; 00428 LCD_LOG_UpdateDisplay(); 00429 LCD_Lock = DISABLE; 00430 } 00431 return SUCCESS; 00432 } 00433 00434 /** 00435 * @brief Display next text frame 00436 * @param None 00437 * @retval Status 00438 */ 00439 ErrorStatus LCD_LOG_ScrollForward(void) 00440 { 00441 00442 if(LCD_ScrollBackStep != 0) 00443 { 00444 if(LCD_ScrollActive == DISABLE) 00445 { 00446 00447 LCD_CacheBuffer_yptr_bottom_bak = LCD_CacheBuffer_yptr_bottom; 00448 LCD_CacheBuffer_yptr_top_bak = LCD_CacheBuffer_yptr_top; 00449 00450 if(LCD_CacheBuffer_yptr_bottom > LCD_CacheBuffer_yptr_top) 00451 { 00452 00453 if ((LCD_CacheBuffer_yptr_bottom - LCD_CacheBuffer_yptr_top) <= YWINDOW_SIZE) 00454 { 00455 LCD_Lock = DISABLE; 00456 return ERROR; 00457 } 00458 } 00459 LCD_ScrollActive = ENABLE; 00460 00461 if((LCD_CacheBuffer_yptr_bottom > LCD_CacheBuffer_yptr_top)&& 00462 (LCD_Scrolled == DISABLE )) 00463 { 00464 LCD_CacheBuffer_yptr_bottom--; 00465 LCD_Scrolled = ENABLE; 00466 } 00467 00468 } 00469 00470 if(LCD_ScrollActive == ENABLE) 00471 { 00472 LCD_Lock = ENABLE; 00473 LCD_ScrollBackStep--; 00474 00475 if(++LCD_CacheBuffer_yptr_bottom == LCD_CACHE_DEPTH) 00476 { 00477 LCD_CacheBuffer_yptr_bottom = 0; 00478 } 00479 00480 LCD_LOG_UpdateDisplay(); 00481 LCD_Lock = DISABLE; 00482 00483 } 00484 return SUCCESS; 00485 } 00486 else // LCD_ScrollBackStep == 0 00487 { 00488 LCD_Lock = DISABLE; 00489 return ERROR; 00490 } 00491 } 00492 #endif /* LCD_SCROLL_ENABLED */ 00493 00494 /** 00495 * @} 00496 */ 00497 00498 /** 00499 * @} 00500 */ 00501 00502 /** 00503 * @} 00504 */ 00505 00506 /** 00507 * @} 00508 */ 00509 00510 /** 00511 * @} 00512 */ 00513 00514 00515 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/ 00516 00517
Generated on Tue Jul 12 2022 21:06:48 by
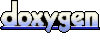