
OSHW for SFM
Dependencies: BufferedSoftSerial WS2812 beep mbed-rtos mbed
main.cpp
00001 #include "mbed.h" 00002 #include "rtos.h" 00003 #include "stdio.h" 00004 #include "stdlib.h" 00005 #include "beep.h" 00006 #include "WS2812.h" 00007 #include "BufferedSoftSerial.h" 00008 #include "pitches.h" 00009 00010 //PwmOut out(D9); //piezo buzzer 00011 DigitalIn btn_enroll(D6); 00012 DigitalIn btn_identify(D7); 00013 DigitalIn btn_delete(D8); 00014 00015 Serial pc(USBTX, USBRX); //debug port 00016 BufferedSoftSerial device(D2, D3); 00017 Beep beep(D9); 00018 00019 float melody[] = { NOTE_C7, NOTE_E7, NOTE_G7, NOTE_C8, NOTE_C8, NOTE_C8 }; 00020 float noteDuration[] = { 8.0, 8.0, 8.0, 4.0, 4.0, 8.0 }; 00021 00022 00023 void melodyStart(); 00024 void melodySuccess(); 00025 void melodyFail(); 00026 WS2812 ws(D11, 1, 7,15,10,15); 00027 int colorbuf[4] = { 0x00000033, 0x0000FFFF, 0x0000FF00, 0x00001199}; //blue, orange, green, red 00028 00029 int main() 00030 { 00031 00032 melodyStart(); 00033 00034 //pc.baud(115200); 00035 device.baud(19200); 00036 unsigned char IS[] = { 0x40, 0x11, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x51, 0x0A}; // Identify by Scan 00037 unsigned char ES[] = { 0x40, 0x05, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x79, 0xBE, 0x0A}; // Enroll by Scan 00038 unsigned char DA[] = { 0x40, 0x17, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x57, 0x0A}; // Delete all 00039 00040 00041 ws.useII(WS2812::GLOBAL); 00042 ws.setII(0xAA); 00043 00044 melodyStart(); 00045 00046 while(1) { 00047 pc.printf("%d %d %d\n\r", btn_enroll, btn_identify, btn_delete); 00048 if(btn_enroll) { 00049 //ws.write(&colorbuf[3]); 00050 device.write((unsigned char*)ES, sizeof(ES)); 00051 00052 for(int i=0; i<2; i++) { 00053 ws.write(&colorbuf[0]); 00054 wait(0.2); 00055 00056 while(1) { 00057 if(device.readable() > 0) 00058 break; 00059 } 00060 00061 00062 unsigned char rxBuffer[256]= {0}; 00063 int count = 0; 00064 00065 wait(0.2); 00066 00067 while(device.readable()>0) { 00068 rxBuffer[count] = (unsigned char)device.getc(); 00069 count++; 00070 } 00071 pc.printf("recv : "); 00072 for(int i=0; i<13; i++) 00073 pc.printf("%d ", rxBuffer[i]); 00074 pc.printf(" count : %d \n\r", count); 00075 00076 if((unsigned char)rxBuffer[10] == 0x62) { 00077 while(1) { 00078 if(device.readable()>0) 00079 break; 00080 } 00081 00082 int count = 0; 00083 00084 wait(0.2); 00085 00086 while(device.readable()) { 00087 rxBuffer[count] = (unsigned char)device.getc(); 00088 count++; 00089 } 00090 00091 unsigned int userID; 00092 userID = rxBuffer[5] << 24 | rxBuffer[4] << 16 | rxBuffer[3] << 8 | rxBuffer[2]; 00093 00094 pc.printf("userID : %d\n\r", userID); 00095 if((unsigned char)rxBuffer[10] == 0x61) { 00096 melodySuccess(); 00097 } else { 00098 melodyFail(); 00099 break; 00100 } 00101 } else { 00102 melodyFail(); 00103 break; 00104 } 00105 } 00106 } 00107 if(btn_identify) { 00108 //ws.write(&colorbuf[3]); 00109 device.write((unsigned char*)IS, sizeof(IS)); 00110 00111 00112 wait(0.2); 00113 00114 while(1) { 00115 if(device.readable() > 0) 00116 break; 00117 } 00118 00119 00120 unsigned char rxBuffer[256]= {0}; 00121 int count = 0; 00122 00123 wait(0.2); 00124 00125 while(device.readable()>0) { 00126 rxBuffer[count] = (unsigned char)device.getc(); 00127 count++; 00128 } 00129 pc.printf("recv : "); 00130 for(int i=0; i<13; i++) 00131 pc.printf("%d ", rxBuffer[i]); 00132 pc.printf(" count : %d \n\r", count); 00133 00134 if((unsigned char)rxBuffer[10] == 0x62) { 00135 while(1) { 00136 if(device.readable()>0) 00137 break; 00138 } 00139 00140 int count = 0; 00141 00142 wait(0.2); 00143 00144 while(device.readable()) { 00145 rxBuffer[count] = (unsigned char)device.getc(); 00146 count++; 00147 } 00148 00149 unsigned int userID; 00150 userID = rxBuffer[5] << 24 | rxBuffer[4] << 16 | rxBuffer[3] << 8 | rxBuffer[2]; 00151 00152 pc.printf("userID : %d\n\r", userID); 00153 if((unsigned char)rxBuffer[10] == 0x61) { 00154 melodySuccess(); 00155 } else { 00156 melodyFail(); 00157 00158 } 00159 } else { 00160 melodyFail(); 00161 00162 } 00163 00164 } 00165 if(btn_delete) { 00166 //ws.write(&colorbuf[3]); 00167 device.write((unsigned char*)DA, sizeof(DA)); 00168 00169 00170 wait(0.2); 00171 00172 while(1) { 00173 if(device.readable() > 0) 00174 break; 00175 } 00176 00177 00178 unsigned char rxBuffer[256]= {0}; 00179 int count = 0; 00180 00181 wait(0.2); 00182 00183 while(device.readable()>0) { 00184 rxBuffer[count] = (unsigned char)device.getc(); 00185 count++; 00186 } 00187 pc.printf("recv : "); 00188 for(int i=0; i<13; i++) 00189 pc.printf("%d ", rxBuffer[i]); 00190 pc.printf(" count : %d \n\r", count); 00191 00192 if((unsigned char)rxBuffer[10] == 0x61) { 00193 melodySuccess(); 00194 } else { 00195 melodyFail(); 00196 00197 } 00198 } 00199 00200 00201 00202 00203 /* 00204 WS2812 ws(D11, 1, 7,15,10,15); 00205 beep.beep(4186.0, 1.0); 00206 wait(1.0); 00207 ws.write(&colorbuf[0]); 00208 wait(0.5); 00209 ws.write(&colorbuf[1]); 00210 wait(0.5); 00211 ws.write(&colorbuf[2]); 00212 wait(0.5); 00213 ws.write(&colorbuf[3]); 00214 wait(0.5); 00215 ws.write(&colorbuf[4]); 00216 wait(0.5); 00217 ws.write(&colorbuf[5]); 00218 wait(0.5); 00219 // if(sfm.writeable()) 00220 00221 00222 device.write((unsigned char*)buff, 13); 00223 00224 wait(5); 00225 */ 00226 //ws.write(&colorbuf[0]); 00227 //wait(0.1); 00228 } 00229 00230 } 00231 00232 void melodyStart() 00233 { 00234 beep.beep(melody[0], 1.0 / (float)noteDuration[0]); 00235 wait((1.0/(float)noteDuration[0])*1.30); 00236 beep.nobeep(); 00237 00238 beep.beep(melody[1], 1.0 / (float)noteDuration[1]); 00239 wait((1.0/(float)noteDuration[0])*1.30); 00240 beep.nobeep(); 00241 00242 beep.beep(melody[2], 1.0 / (float)noteDuration[2]); 00243 wait((1.0/(float)noteDuration[0])*1.30); 00244 beep.nobeep(); 00245 00246 beep.beep(melody[3], 1.0 / (float)noteDuration[3]); 00247 wait((1.0/(float)noteDuration[0])*1.30); 00248 beep.nobeep(); 00249 } 00250 00251 void melodySuccess() 00252 { 00253 ws.write(&colorbuf[2]); 00254 00255 beep.beep(melody[0], 1.0 / noteDuration[0]); 00256 wait((1.0/noteDuration[0])*1.30); 00257 beep.nobeep(); 00258 00259 beep.beep(melody[1], 1.0 / noteDuration[1]); 00260 wait((1.0/noteDuration[0])*1.30); 00261 beep.nobeep(); 00262 00263 beep.beep(melody[2], 1.0 / noteDuration[2]); 00264 wait((1.0/noteDuration[0])*1.30); 00265 beep.nobeep(); 00266 ws.write(&colorbuf[0]); 00267 } 00268 void melodyFail() 00269 { 00270 ws.write(&colorbuf[3]); 00271 beep.beep(melody[4], 1.0 / noteDuration[4]); 00272 wait((1.0/noteDuration[0])*1.30); 00273 beep.nobeep(); 00274 00275 beep.beep(melody[5], 1.0 / noteDuration[5]); 00276 wait((1.0/noteDuration[0])*1.30); 00277 beep.nobeep(); 00278 ws.write(&colorbuf[0]); 00279 }
Generated on Fri Jul 15 2022 22:33:56 by
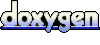