
Example program of using USB mass storage device (USB flash disk) with STM32F407VET6 boards (compatible with Seed Arch Max). This is a fork of https://os.mbed.com/users/va009039/code/F401RE-USBHostMSD_HelloWorld/
Dependencies: mbed FATFileSystem USBHost-STM32F4
main.cpp
00001 #include "USBHostMSD.h" 00002 00003 DigitalOut led1(LED1); 00004 00005 int main() 00006 { 00007 printf("Starting..\r\n"); 00008 00009 USBHostMSD msd("usb"); 00010 00011 printf("Connecting the device\r\n"); 00012 00013 if (!msd.connect()) { 00014 error("USB mass storage device not found.\n"); 00015 } 00016 00017 FILE* fp = fopen("/usb/test1.txt", "a"); 00018 if (fp) { 00019 fprintf(fp, "Hello from mbed.\n"); 00020 for (int i = 0; i < 21; i++) { 00021 fprintf(fp, " %d", i); 00022 led1 = !led1; 00023 } 00024 00025 fprintf(fp, "\n"); 00026 fclose(fp); 00027 } 00028 00029 fp = fopen("/usb/test1.txt", "r"); 00030 if (fp) { 00031 int n = 0; 00032 while (1) { 00033 int c = fgetc(fp); 00034 if (c == EOF) { 00035 break; 00036 } 00037 00038 printf("%c", c); 00039 n++; 00040 led1 = !led1; 00041 } 00042 00043 fclose(fp); 00044 printf("%d bytes\n", n); 00045 } 00046 00047 while (1) { 00048 led1 = !led1; 00049 wait_ms(200); 00050 } 00051 }
Generated on Sat Jul 23 2022 19:57:37 by
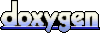