
A simple Pong game with STM32F407VET6 black board (Seeed Arch Max) and ILI9341 320x240 TFT display.
Dependencies: mbed ILI9341_STM32F4
Ball.cpp
00001 #include "Ball.h" 00002 #include "Racket.h" 00003 00004 /** 00005 * @brief 00006 * @note 00007 * @param 00008 * @retval 00009 */ 00010 Ball::Ball(int s /*= 8*/, float v /*= 8*/, uint16_t clr /*= TFT_WHITE*/ ) : 00011 size(s), 00012 velocity(v), 00013 color(clr), 00014 xPos(320 / 2), 00015 yPos(240 / 2), 00016 xPosOld(320 / 2), 00017 yPosOld(240 / 2), 00018 xDir(-1), 00019 yDir(1) 00020 { } 00021 00022 /** 00023 * @brief 00024 * @note 00025 * @param 00026 * @retval 00027 */ 00028 void Ball::home() 00029 { 00030 xPos = 320 / 2; 00031 yPos = 240 / 2; 00032 xPosOld = xPos; 00033 yPosOld = yPos; 00034 xDir = -1; 00035 yDir = 1; 00036 } 00037 00038 /** 00039 * @brief 00040 * @note 00041 * @param 00042 * @retval 00043 */ 00044 bool Ball::move(Racket* rkt) 00045 { 00046 xPosOld = xPos; 00047 yPosOld = yPos; 00048 xPos += xDir * velocity; 00049 yPos += yDir * velocity; 00050 00051 // hit by racket? 00052 if 00053 ( 00054 rkt->xPos - rkt->width / 2 < xPos + size / 2 && 00055 xPos + size / 2 < rkt->xPos && 00056 rkt->yPos - rkt->height / 2 < yPos + size / 2 && 00057 yPos - size / 2 < rkt->yPos + rkt->height / 2 00058 ) { 00059 xPos = rkt->xPos - rkt->width / 2 - size / 2; 00060 xDir = -fabs(xDir); // force it to be negative 00061 } 00062 00063 // missed racket? 00064 if (xPos > 320) { 00065 xPos = 320 / 2; 00066 yPos = 240 / 2; 00067 xDir = -1; 00068 yDir = 1; 00069 return true; 00070 } 00071 00072 // hit left wall? 00073 if (xPos - size / 2 < 0) { 00074 xPos = size / 2; 00075 xDir = fabs(xDir); // force it to be positive 00076 } 00077 00078 // hit bottom wall? 00079 if (yPos + size / 2 > 240) { 00080 yPos = 240 - size / 2; 00081 yDir = -fabs(yDir); // force it to be negative 00082 } 00083 00084 // hit top wall? 00085 if (yPos - size / 2 < 0) { 00086 yPos = size / 2; 00087 yDir = fabs(yDir); // force it to be positive 00088 } 00089 00090 // make sure that length of dir stays at 1 00091 vec2_norm(xDir, yDir); 00092 00093 return false; 00094 } 00095 00096 /** 00097 * @brief 00098 * @note 00099 * @param 00100 * @retval 00101 */ 00102 void Ball::paint() 00103 { 00104 tft_boxfill(xPosOld - size / 2, yPosOld - size / 2, xPosOld + size / 2, yPosOld + size / 2, TFT_BLACK); // hide ball at old position 00105 tft_boxfill(xPos - size / 2, yPos - size / 2, xPos + size / 2, yPos + size / 2, TFT_WHITE); // draw ball at new position 00106 } 00107 00108 /** 00109 * @brief 00110 * @note 00111 * @param 00112 * @retval 00113 */ 00114 void Ball::vec2_norm(float& x, float& y) 00115 { 00116 // sets vector's length to 1 (which means that x + y = 1) 00117 float length = sqrt((x * x) + (y * y)); 00118 if (length != 0.0f) { 00119 length = 1.0f / length; 00120 x *= length; 00121 y *= length; 00122 } 00123 }
Generated on Mon Jul 25 2022 11:48:50 by
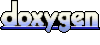