Library for Manchester encoding using UART's hardware.
Dependents: ManchesterUART_Transmitter ManchesterUART_Receiver
ManchesterUART.h
00001 /* 00002 ****************************************************************************** 00003 * @file ManchesterUART.h 00004 * @author Zoltan Hudak 00005 * @version 00006 * @date 2017-Nov-22 00007 * @brief Manchester code over UART for mbed 00008 ****************************************************************************** 00009 * @attention 00010 * 00011 * <h2><center>© COPYRIGHT(c) 2017 Zoltan Hudak <hudakz@outlook.com> 00012 * 00013 * All rights reserved. 00014 00015 This program is free software: you can redistribute it and/or modify 00016 it under the terms of the GNU General Public License as published by 00017 the Free Software Foundation, either version 3 of the License, or 00018 (at your option) any later version. 00019 00020 This program is distributed in the hope that it will be useful, 00021 but WITHOUT ANY WARRANTY; without even the implied warranty of 00022 MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00023 GNU General Public License for more details. 00024 00025 You should have received a copy of the GNU General Public License 00026 along with this program. If not, see <http://www.gnu.org/licenses/>. 00027 */ 00028 /* 00029 * This library implements Manchester code using UART serial connection. Each 00030 * data byte is encoded into two bytes representing two nibbles of the original 00031 * data byte. These two bytes are then sent over UART serial link connection. 00032 * The receiver reconstructs the original data byte from the two bytes received. 00033 * A start and stop pattern are sent to signify the begin and end of a message. 00034 * 00035 * The library is based on the article published by Adrian Mills: 00036 * http://www.quickbuilder.co.uk/qb/articles/Manchester_encoding_using_RS232.pdf 00037 */ 00038 #ifndef MANCHESTERUART_H 00039 #define MANCHESTERUART_H 00040 00041 #include "mbed.h" 00042 #include "ManchesterMsg.h" 00043 00044 #define START 0xF0 // start pattern 00045 00046 #define STOP 0x0F // stop pattern 00047 class ManchesterUART 00048 { 00049 enum Error 00050 { 00051 NO_ERROR, 00052 ILLEGAL_CODE, 00053 RX_TIMEOUT, 00054 BUF_OVERRUN 00055 }; 00056 public: 00057 /** 00058 * @brief Creates a ManchesterUART object 00059 * @note 00060 * @param txPin Pin name of transmitter line 00061 * @param rxPin Pin name of receiver line 00062 * @param preamble Number of start patterns at the begin of each transmission 00063 * @param baudrate Communication bit rate in bits per second. Defaults to 115200bps 00064 * @param timeout Receive timeout in seconds. Defaults to 5 seconds. 00065 * @retval 00066 */ 00067 ManchesterUART 00068 ( 00069 PinName txPin, 00070 PinName rxPin, 00071 int baudrate = 9600, /* bits/sec */ 00072 uint8_t preamble = 8, /* number of start patterns */ 00073 float rxTimeout = 5 /* seconds */ 00074 ) : 00075 _serial(txPin, rxPin, baudrate), 00076 _rxTimeout(rxTimeout), 00077 _preamble(preamble), 00078 _error(NO_ERROR) 00079 { } 00080 00081 ~ ManchesterUART(void) { } 00082 void transmit(ManchesterMsg& msg); 00083 void attach(Callback<void ()> func, SerialBase::IrqType type = SerialBase::RxIrq) { _serial.attach(func, type); } 00084 bool receive(ManchesterMsg& msg); 00085 bool readable() { return _serial.readable(); } 00086 void baud(int baudrate) { _serial.baud(baudrate); } 00087 void setPreamble(uint8_t length) { _preamble = length; } 00088 void setRxTimeout(int seconds) { _rxTimeout = seconds; } 00089 Error lastError() { return _error; } 00090 private: 00091 Serial _serial; 00092 char* _data; // data array 00093 size_t _len; // data length in bytes 00094 size_t _maxLen; // data maximum length 00095 Timeout _timeout; // timeout 00096 float _rxTimeout; // timeout time in seconds 00097 uint8_t _preamble; // number of start patterns 00098 Error _error; // error flag 00099 void rxTimeout(void); 00100 void transmitByte(uint8_t data); 00101 uint8_t receiveByte(void); 00102 uint8_t getNibble(uint8_t nibble); 00103 }; 00104 #endif // MANCHESTERUART_H
Generated on Tue Jul 12 2022 21:49:11 by
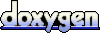