Software implemented real time clock driven by a Ticker. No external hardware (like DS1307 or DS3231 or etc.) is needed. Should work on any mbed platform where Ticker works.
Clock.h
00001 /* 00002 Clock.h 00003 00004 Created on: Mar 24, 2015 00005 Author: Zoltan Hudak 00006 00007 Copyright (c) 2015 Zoltan Hudak <hudakz@outlook.com> 00008 All rights reserved. 00009 00010 This program is free software: you can redistribute it and/or modify 00011 it under the terms of the GNU General Public License as published by 00012 the Free Software Foundation, either version 3 of the License, or 00013 (at your option) any later version. 00014 00015 This program is distributed in the hope that it will be useful, 00016 but WITHOUT ANY WARRANTY; without even the implied warranty of 00017 MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00018 GNU General Public License for more details. 00019 00020 You should have received a copy of the GNU General Public License 00021 along with this program. If not, see <http://www.gnu.org/licenses/>. 00022 */ 00023 #ifndef Clock_H_ 00024 #define Clock_H_ 00025 00026 #include "mbed.h" 00027 00028 class Clock 00029 { 00030 Ticker* _ticker; 00031 static time_t _time; 00032 static struct tm _tm; 00033 protected: 00034 static Callback<void ()> _onTick; 00035 public: 00036 Clock(int year, int mon, int mday, int hour, int min, int sec); 00037 Clock(); 00038 ~Clock(); 00039 void set(int year, int mon, int mday, int hour, int min, int sec); 00040 void set(tm& val); 00041 void set(time_t time); 00042 static time_t time(); 00043 int year(void); 00044 int mon(void); 00045 int mday(void); 00046 int wday(void); 00047 int hour(void); 00048 int min(void); 00049 int sec(void); 00050 static void tick(void); 00051 static time_t asTime(int year, int mon, int mday, int hour, int min, int sec); 00052 void attach(void (*fptr) (void)); 00053 template<typename T> void attach(T* tptr, void (T:: *mptr) (void)); 00054 void detach(); 00055 }; 00056 #endif /* Clock_H_ */
Generated on Sat Jul 23 2022 01:20:29 by
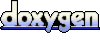