
HelloMQTT porting to FRDM-K64F+AppShield
Dependencies: C12832 EthernetInterface MQTT mbed-rtos mbed
Fork of HelloMQTT by
main.cpp
00001 /******************************************************************************* 00002 * Copyright (c) 2014 IBM Corp. 00003 * 00004 * All rights reserved. This program and the accompanying materials 00005 * are made available under the terms of the Eclipse Public License v1.0 00006 * and Eclipse Distribution License v1.0 which accompany this distribution. 00007 * 00008 * The Eclipse Public License is available at 00009 * http://www.eclipse.org/legal/epl-v10.html 00010 * and the Eclipse Distribution License is available at 00011 * http://www.eclipse.org/org/documents/edl-v10.php. 00012 * 00013 * Contributors: 00014 * Ian Craggs - initial API and implementation and/or initial documentation 00015 *******************************************************************************/ 00016 00017 /** 00018 This is a sample program to illustrate the use of the MQTT Client library 00019 on the mbed platform. The Client class requires two classes which mediate 00020 access to system interfaces for networking and timing. As long as these two 00021 classes provide the required public programming interfaces, it does not matter 00022 what facilities they use underneath. In this program, they use the mbed 00023 system libraries. 00024 00025 */ 00026 00027 00028 #include "C12832.h" 00029 //C12832 lcd(p5, p7, p6, p8, p11); 00030 // Using Arduino pin notation 00031 C12832 lcd(D11, D13, D12, D7, D10); 00032 00033 #include "MQTTEthernet.h" 00034 #include "MQTTClient.h" 00035 00036 int arrivedcount = 0; 00037 00038 00039 void messageArrived(MQTT::MessageData& md) 00040 { 00041 MQTT::Message &message = md.message; 00042 lcd.cls(); 00043 lcd.locate(0,3); 00044 printf("Message arrived: qos %d, retained %d, dup %d, packetid %d\n", message.qos, message.retained, message.dup, message.id); 00045 printf("Payload %.*s\n", message.payloadlen, (char*)message.payload); 00046 ++arrivedcount; 00047 lcd.puts((char*)message.payload); 00048 } 00049 00050 00051 int main(int argc, char* argv[]) 00052 { 00053 MQTTEthernet ipstack = MQTTEthernet(); 00054 float version = 0.47; 00055 char* topic = "mbed-sample"; 00056 00057 lcd.printf("Version is %f\n", version); 00058 printf("Version is %f\n", version); 00059 00060 MQTT::Client<MQTTEthernet, Countdown> client = MQTT::Client<MQTTEthernet, Countdown>(ipstack); 00061 00062 char* hostname = "m2m.eclipse.org"; 00063 int port = 1883; 00064 lcd.printf("Connecting to %s:%d\n", hostname, port); 00065 int rc = ipstack.connect(hostname, port); 00066 if (rc != 0) 00067 lcd.printf("rc from TCP connect is %d\n", rc); 00068 00069 MQTTPacket_connectData data = MQTTPacket_connectData_initializer; 00070 data.MQTTVersion = 3; 00071 data.clientID.cstring = "mbed-sample"; 00072 data.username.cstring = "testuser"; 00073 data.password.cstring = "testpassword"; 00074 if ((rc = client.connect(data)) != 0) 00075 lcd.printf("rc from MQTT connect is %d\n", rc); 00076 00077 if ((rc = client.subscribe(topic, MQTT::QOS1, messageArrived)) != 0) 00078 lcd.printf("rc from MQTT subscribe is %d\n", rc); 00079 00080 MQTT::Message message; 00081 00082 // QoS 0 00083 char buf[100]; 00084 sprintf(buf, "Hello World! QoS 0 message from app version %f\n", version); 00085 message.qos = MQTT::QOS0; 00086 message.retained = false; 00087 message.dup = false; 00088 message.payload = (void*)buf; 00089 message.payloadlen = strlen(buf)+1; 00090 rc = client.publish(topic, message); 00091 while (arrivedcount < 1) 00092 client.yield(100); 00093 00094 // QoS 1 00095 sprintf(buf, "Hello World! QoS 1 message from app version %f\n", version); 00096 message.qos = MQTT::QOS1; 00097 message.payloadlen = strlen(buf)+1; 00098 rc = client.publish(topic, message); 00099 while (arrivedcount < 2) 00100 client.yield(100); 00101 00102 // QoS 2 00103 sprintf(buf, "Hello World! QoS 2 message from app version %f\n", version); 00104 message.qos = MQTT::QOS2; 00105 message.payloadlen = strlen(buf)+1; 00106 rc = client.publish(topic, message); 00107 while (arrivedcount < 3) 00108 client.yield(100); 00109 00110 // n * QoS 2 00111 for (int i = 1; i <= 10; ++i) 00112 { 00113 sprintf(buf, "Hello World! QoS 2 message number %d from app version %f\n", i, version); 00114 message.qos = MQTT::QOS2; 00115 message.payloadlen = strlen(buf)+1; 00116 rc = client.publish(topic, message); 00117 while (arrivedcount < i + 3) 00118 client.yield(100); 00119 } 00120 00121 if ((rc = client.unsubscribe(topic)) != 0) 00122 printf("rc from unsubscribe was %d\n", rc); 00123 00124 if ((rc = client.disconnect()) != 0) 00125 printf("rc from disconnect was %d\n", rc); 00126 00127 ipstack.disconnect(); 00128 00129 lcd.cls(); 00130 lcd.locate(0,3); 00131 lcd.printf("Version %.2f: finish %d msgs\n", version, arrivedcount); 00132 printf("Finishing with %d messages received\n", arrivedcount); 00133 00134 return 0; 00135 }
Generated on Mon Jul 18 2022 08:29:03 by
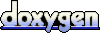