
Hiber
Embed:
(wiki syntax)
Show/hide line numbers
MAXM8.h
00001 #ifndef MAXM8_H_INCLUDED 00002 #define MAXM8_H_INCLUDED 00003 #include <stdbool.h> 00004 00005 #define MAX_BUFFER_SIZE 90 00006 #define MAX_FIELDS_NUMBER 25 00007 #define FALSE 0 00008 #define TRUE 1 00009 #define UNDEFINED 3 00010 00011 00012 enum minmea_sentence_id { 00013 MINMEA_INVALID = -1, 00014 MINMEA_UNKNOWN = 0, 00015 NMEA_SENTENCE_RMC, 00016 NMEA_SENTENCE_GGA, 00017 NMEA_SENTENCE_VTG, 00018 }; 00019 00020 struct minmea_coord { 00021 long integer; 00022 long decimal; 00023 uint8_t cardeal; 00024 }; 00025 00026 struct minmea_date { 00027 int day; 00028 int month; 00029 int year; 00030 }; 00031 00032 struct minmea_time { 00033 int hours; 00034 int minutes; 00035 int seconds; 00036 int microseconds; 00037 }; 00038 00039 struct minmea_sentence { 00040 struct minmea_time time; 00041 struct minmea_coord latitude; 00042 struct minmea_coord longitude; 00043 struct minmea_date date; 00044 00045 bool valid; 00046 int altitude; 00047 int speed; 00048 int fix_quality; 00049 int num_of_satellites_tracked; 00050 00051 }; 00052 00053 bool MAXM8_NMEA_Putc(char uart_char, char *uart_buffer_prt); 00054 bool MAXM8_NMEA_Parser(struct minmea_sentence *gps_frame,int *isPositionDataValid,char *uart_buffer_prt); 00055 00056 #endif // MAXM8_H_INCLUDED
Generated on Mon Aug 1 2022 15:56:19 by
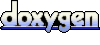