Mbed port of the Simple Plain Xml parser. See http://code.google.com/p/spxml/ for more details. This library uses less memory and is much better suited to streaming data than TinyXML (doesn\'t use as much C++ features, and especially works without streams). See http://mbed.org/users/hlipka/notebook/xml-parsing/ for usage examples.
Dependents: spxmltest_weather VFD_fontx2_weather weather_LCD_display News_LCD_display ... more
spdomparser.hpp
00001 /* 00002 * Copyright 2007 Stephen Liu 00003 * LGPL, see http://code.google.com/p/spxml/ 00004 * For license terms, see the file COPYING along with this library. 00005 */ 00006 00007 #ifndef __spdomparser_hpp__ 00008 #define __spdomparser_hpp__ 00009 00010 class SP_XmlNode; 00011 class SP_XmlDocument; 00012 class SP_XmlElementNode; 00013 class SP_XmlDocDeclNode; 00014 class SP_XmlDocTypeNode; 00015 class SP_XmlPullParser; 00016 class SP_XmlStringBuffer; 00017 00018 /// parse string to xml node tree 00019 class SP_XmlDomParser { 00020 public: 00021 SP_XmlDomParser(); 00022 ~SP_XmlDomParser(); 00023 00024 /// append more input xml source 00025 /// @return how much byte has been consumed 00026 int append( const char * source, int len ); 00027 00028 /// @return NOT NULL : the detail error message 00029 /// @return NULL : no error 00030 const char * getError (); 00031 00032 /// get the parse result 00033 const SP_XmlDocument * getDocument() const; 00034 00035 void setIgnoreWhitespace( int ignoreWhitespace ); 00036 00037 int getIgnoreWhitespace(); 00038 00039 const char * getEncoding(); 00040 00041 private: 00042 void buildTree(); 00043 00044 SP_XmlDomParser( SP_XmlDomParser & ); 00045 SP_XmlDomParser & operator=( SP_XmlDomParser & ); 00046 00047 SP_XmlPullParser * mParser; 00048 SP_XmlDocument * mDocument; 00049 SP_XmlElementNode * mCurrent; 00050 }; 00051 00052 /// serialize xml node tree to string 00053 class SP_XmlDomBuffer { 00054 public: 00055 SP_XmlDomBuffer( const SP_XmlNode * node, int indent = 1 ); 00056 SP_XmlDomBuffer( const char * encoding, const SP_XmlNode * node, int indent = 1 ); 00057 ~SP_XmlDomBuffer(); 00058 00059 const char * getBuffer() const; 00060 int getSize() const; 00061 00062 private: 00063 SP_XmlDomBuffer( SP_XmlDomBuffer & ); 00064 SP_XmlDomBuffer & operator=( SP_XmlDomBuffer & ); 00065 00066 static void dumpDocDecl( const char * encoding, 00067 const SP_XmlDocDeclNode * docDecl, 00068 SP_XmlStringBuffer * buffer, int level ); 00069 static void dumpDocType( const char * encoding, 00070 const SP_XmlDocTypeNode * docType, 00071 SP_XmlStringBuffer * buffer, int level ); 00072 static void dump( const char * encoding, 00073 const SP_XmlNode * node, 00074 SP_XmlStringBuffer * buffer, int level ); 00075 static void dumpElement( const char * encoding, 00076 const SP_XmlNode * node, 00077 SP_XmlStringBuffer * buffer, int level ); 00078 00079 SP_XmlStringBuffer * mBuffer; 00080 }; 00081 00082 #endif 00083
Generated on Sun Jul 17 2022 09:10:11 by
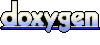