
program to test a possible memory leak when using NTP
Dependencies: NetServices mbed
main.cpp
00001 #include "mbed.h" 00002 00003 #include "EthernetNetIf.h" 00004 #include "NTPClient.h" 00005 00006 #include "AvailableMemory.h" 00007 00008 LocalFileSystem local("local"); 00009 00010 void updateTime() 00011 { 00012 time_t ctTime; 00013 time(&ctTime); 00014 printf("Current time is (UTC): %s\n", ctime(&ctTime)); 00015 00016 NTPClient ntp; 00017 Host server(IpAddr(), 123, "de.pool.ntp.org"); 00018 ntp.setTime(server); 00019 00020 printf("set time ok\n"); 00021 time(&ctTime); 00022 printf("Current time is (UTC): %s\n", ctime(&ctTime)); 00023 00024 } 00025 00026 int main() { 00027 printf("******** [%s] *********\n",__TIME__); 00028 printf("calculate free mem 1\n"); 00029 int i=AvailableMemory(1,0x8000,false); 00030 printf("free mem=%i\n",i); 00031 00032 // void* p1=malloc(8000); 00033 // void* p2=malloc(8000); 00034 00035 printf("setup\n"); 00036 EthernetNetIf eth; 00037 EthernetErr ethErr; 00038 printf("Setting up network...\n"); 00039 do { 00040 ethErr = eth.setup(); 00041 if (ethErr) printf("waiting for network...\n", ethErr); 00042 } while (ethErr != ETH_OK); 00043 00044 printf("setup ok\n"); 00045 00046 updateTime(); 00047 00048 // free(p1); 00049 // free(p2); 00050 printf("calculate free mem 4\n"); 00051 i=AvailableMemory(1,0x100,false); 00052 printf("free mem=%i\n",i); 00053 i=AvailableMemory(1,0x8000,false); 00054 printf("free mem=%i\n",i); 00055 }
Generated on Thu Jul 14 2022 12:36:48 by
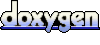