
program to test a possible memory leak when using NTP
Dependencies: NetServices mbed
AvailableMemory.h
00001 /** @file 00002 * Return the memory available for a malloc call. 00003 */ 00004 #ifndef SEGUNDO_UTILITIES_AVAILABLEMEMORY_H 00005 #define SEGUNDO_UTILITIES_AVAILABLEMEMORY_H 00006 00007 /** 00008 * Segundo Equipo 00009 */ 00010 namespace segundo { 00011 /** 00012 * A collection of utilities 00013 */ 00014 namespace Utilities { 00015 00016 /** Return the memory available for a malloc call. 00017 * This is done by a binary search approach 00018 * calling malloc/free starting with a maximum. 00019 * 00020 * Example: 00021 * @code 00022 * #include <stdio.h> 00023 * #include "AvailableMemory.h" 00024 * 00025 * int main() { 00026 * 00027 * printf("Available memory (bytes to nearest 256) : %d\n", AvailableMemory()); 00028 * printf("Available memory (exact bytes) : %d\n", AvailableMemory(1)); 00029 * 00030 * } 00031 * @endcode 00032 * @param resolution Resolution in number of bytes, 00033 * 1 will return the exact value, 00034 * default will return the available memory to the nearest 256 bytes 00035 * @param maximum Maximum amount of memory to check, default is 32K (0x8000) 00036 * @param disableInterrupts Disable interrupts whilst checking, default is true 00037 * @return Available memory in bytes accurate to within resolution 00038 */ 00039 int AvailableMemory(int resolution = 256, int maximum = 0x8000, bool disableInterrupts = true); 00040 00041 } // namespace Utilities 00042 } // namespace segundo 00043 00044 using namespace segundo::Utilities; 00045 00046 #endif // SEGUNDO_UTILITIES_AVAILABLEMEMORY_H
Generated on Thu Jul 14 2022 12:36:48 by
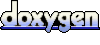