
program to test a possible memory leak when using NTP
Dependencies: NetServices mbed
AvailableMemory.cpp
00001 #include "AvailableMemory.h" 00002 #include <stdlib.h> 00003 #include <stdio.h> 00004 00005 namespace segundo { 00006 namespace Utilities { 00007 00008 int AvailableMemory(int resolution, int maximum, bool disableInterrupts) { 00009 00010 if (resolution < 1) resolution = 1; 00011 if (maximum < 0) maximum = 0; 00012 00013 int low = 0; 00014 int high = maximum + 1; 00015 00016 if (disableInterrupts) __disable_irq(); 00017 00018 while (high - low > resolution) { 00019 int mid = (low + high) / 2; 00020 printf("try malloc %i bytes\n",mid); 00021 void* p = malloc(mid); 00022 if (p == NULL) { 00023 high = mid; 00024 } else { 00025 free(p); 00026 low = mid; 00027 } 00028 } 00029 00030 if (disableInterrupts) __enable_irq(); 00031 00032 return low; 00033 } 00034 00035 } // namespace Utilities 00036 } // namespace segundo
Generated on Thu Jul 14 2022 12:36:48 by
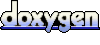