touch screen handler for the microchip AR1020
Embed:
(wiki syntax)
Show/hide line numbers
AreaTouchHandler.cpp
00001 /* 00002 * mbed AR1020 library 00003 * Copyright (c) 2010 Hendrik Lipka 00004 * 00005 * Permission is hereby granted, free of charge, to any person obtaining a copy 00006 * of this software and associated documentation files (the "Software"), to deal 00007 * in the Software without restriction, including without limitation the rights 00008 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00009 * copies of the Software, and to permit persons to whom the Software is 00010 * furnished to do so, subject to the following conditions: 00011 * 00012 * The above copyright notice and this permission notice shall be included in 00013 * all copies or substantial portions of the Software. 00014 * 00015 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00016 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00017 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00018 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00019 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00020 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00021 * THE SOFTWARE. 00022 */ 00023 00024 #include "AreaTouchHandler.h" 00025 #include "touchevent.h" 00026 00027 using namespace std; 00028 00029 class area 00030 { 00031 public: 00032 int top,bottom,left,right,command; 00033 area(int top, int bottom, int left, int right, int command) 00034 { 00035 this->top=top; 00036 this->bottom=bottom; 00037 this->left=left; 00038 this->right=right; 00039 this->command=command; 00040 } 00041 }; 00042 00043 AreaTouchHandler::AreaTouchHandler(TouchPanel* panel) 00044 { 00045 panel->attachPenDown(this,&AreaTouchHandler::down); 00046 panel->attachPenMove(this,&AreaTouchHandler::move); 00047 panel->attachPenUp(this,&AreaTouchHandler::up); 00048 } 00049 00050 void AreaTouchHandler::addArea(int top, int bottom, int left, int right, int commandCode) 00051 { 00052 _areas.push_back(new area(top,bottom,left,right,commandCode)); 00053 } 00054 00055 uint32_t AreaTouchHandler::down(uint32_t arg) 00056 { 00057 TouchEvent *te=(TouchEvent*)arg; 00058 // printf("d %i/%i\n",te->x,te->y); 00059 _x=te->x; 00060 _y=te->y; 00061 _samples=1; 00062 int c=findCommand(); 00063 _called=false; 00064 if (0!=c) 00065 { 00066 _called=true; 00067 _callback.call(c); 00068 } 00069 return 0; 00070 } 00071 uint32_t AreaTouchHandler::move(uint32_t arg) 00072 { 00073 if (_called) 00074 return 0; 00075 TouchEvent *te=(TouchEvent*)arg; 00076 // printf("m %i/%i\n",te->x,te->y); 00077 _x=(te->x+_x*_samples)/(_samples+1); 00078 _y=(te->y+_y*_samples)/(_samples+1); 00079 _samples++; 00080 int c=findCommand(); 00081 if (0!=c) 00082 { 00083 _called=true; 00084 _callback.call(c); 00085 } 00086 return 0; 00087 } 00088 uint32_t AreaTouchHandler::up(uint32_t arg) 00089 { 00090 _x=0; 00091 _y=0; 00092 _samples=0; 00093 _called=false; 00094 return 0; 00095 } 00096 00097 int AreaTouchHandler::findCommand() 00098 { 00099 if (_called) 00100 return 0; 00101 if (_samples<5) 00102 return 0; 00103 for (list<area*>::iterator it = _areas.begin(); it != _areas.end(); it++) { 00104 area* a=*it; 00105 if (_x>=a->left && _x<=a->right && _y>=a->top && _y<=a->bottom) 00106 return a->command; 00107 } 00108 return 0; 00109 }
Generated on Tue Jul 12 2022 18:15:37 by
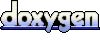