Display text on LCD displays (even on multiple ones). Allow to create windows (frames) on display, and to combine them (split, add, duplicate, scroll). See http://mbed.org/users/hlipka/notebook/lcdwindow/ for more information.
subwindow.cpp
00001 /* 00002 * mbed LCDWindow library 00003 * Copyright (c) 2010 Hendrik Lipka 00004 * 00005 * Permission is hereby granted, free of charge, to any person obtaining a copy 00006 * of this software and associated documentation files (the "Software"), to deal 00007 * in the Software without restriction, including without limitation the rights 00008 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00009 * copies of the Software, and to permit persons to whom the Software is 00010 * furnished to do so, subject to the following conditions: 00011 * 00012 * The above copyright notice and this permission notice shall be included in 00013 * all copies or substantial portions of the Software. 00014 * 00015 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00016 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00017 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00018 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00019 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00020 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00021 * THE SOFTWARE. 00022 */ 00023 00024 #include "subwindow.h" 00025 00026 #include "string.h" 00027 00028 SubWindow::SubWindow(Window* lcd, const unsigned int columnOffset, const unsigned int rowOffset, const unsigned int columns, const unsigned int rows) 00029 :Window() { 00030 _lcd=lcd; 00031 _columnOffset=columnOffset; 00032 _rowOffset=rowOffset; 00033 _columns=columns; 00034 _rows=rows; 00035 00036 } 00037 00038 void SubWindow::character(int column, int row, int c) 00039 { 00040 if (column>_columns || row>_rows) 00041 return; 00042 00043 _lcd->character(column+_columnOffset, row+_rowOffset, c); 00044 } 00045 00046 void SubWindow::writeText(const unsigned int column, const unsigned int row, const char* text) { 00047 if (row>_rows) 00048 return; 00049 00050 char* text2=new char[_columns-column+1]; 00051 int i=0; 00052 while (i<_columns-column+1) { 00053 text2[i]=text[i]; 00054 if (text[i]=='\0') 00055 break; 00056 i++; 00057 } 00058 text2[_columns-column]='\0'; 00059 00060 _lcd->writeText(column+_columnOffset, row+_rowOffset, text2); 00061 delete [] text2; 00062 } 00063 00064 void SubWindow::clear() { 00065 char* spaces=new char[_columns+1]; 00066 memset(spaces,32,_columns); 00067 spaces[_columns]=0; 00068 for (int i=0;i<_rows;i++) { 00069 _lcd->writeText(_columnOffset,_rowOffset+i,spaces); 00070 } 00071 delete spaces; 00072 }
Generated on Tue Jul 12 2022 20:45:22 by
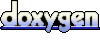