
test2
Dependencies: C12832 mbed-http
Fork of Mbed-to-cybozu-kintone_get_sample by
main.cpp
00001 #include "mbed.h" 00002 #include <sstream> 00003 #include "easy-connect.h" 00004 #include "https_request.h" 00005 #include "ssl_ca_pem.h" 00006 #include "C12832.h" 00007 00008 C12832 lcd(D11, D13, D12, D7, D10); 00009 DigitalIn up(A2); 00010 DigitalIn down(A3); 00011 DigitalIn left(A4); 00012 DigitalIn right(A5); 00013 DigitalIn fire(D4); 00014 PwmOut spkr(D6); 00015 00016 Serial pc(USBTX,USBRX); 00017 00018 const char API_TOKEN[] = "Lyqz2ABnQPaNy6DXGincIfC3ulrnldLJROxnPvUD"; 00019 const char URL[] = "https://owtd6.cybozu.com/k/v1/record.json"; 00020 00021 int app_id = 120; 00022 00023 // JSON simplicity parser 00024 char* j_paser( const char *buf , char *word , char *out ) 00025 { 00026 int i = 0; 00027 char *p; 00028 char _word[64] = "\"\0"; 00029 00030 strcat(_word , word ); 00031 strcat(_word , "\"" ); 00032 00033 p = strstr( (char*)buf , _word ) + 2 + strlen(_word); 00034 00035 while( (p[i] != ',')&&(p[i] != '\n')&&(p[i] != '"') ) 00036 { 00037 out[i] = p[i]; 00038 i++; 00039 } 00040 out[i] = '\0'; 00041 00042 return p; 00043 } 00044 00045 // main() runs in its own thread in the OS 00046 int main() { 00047 NetworkInterface* network = NULL; 00048 00049 pc.baud(115200); 00050 00051 lcd.cls(); 00052 lcd.locate(0,3); 00053 lcd.printf("Network Connect.."); 00054 00055 pc.printf("\r\n----- Start -----\r\n"); 00056 00057 network = easy_connect(true); // If true, prints out connection details. 00058 if (!network) { 00059 lcd.printf("NG"); 00060 pc.printf("\r\n----- Network Error -----\r\n"); 00061 return -1; 00062 } 00063 00064 lcd.printf("OK"); 00065 pc.printf("\r\n----- Network Connected -----\r\n"); 00066 00067 wait(2.0); 00068 00069 while(1){ 00070 int id = 1; 00071 00072 // ID select 00073 lcd.cls(); 00074 lcd.locate(0,3); 00075 lcd.printf("Please select id."); 00076 while(fire == 0){ 00077 lcd.locate(0,14); 00078 lcd.printf("id = "); 00079 if( up == 1) id++; 00080 if( down == 1) id--; 00081 lcd.printf("%d ",id); 00082 wait(0.2); 00083 } 00084 00085 lcd.cls(); 00086 lcd.locate(0,3); 00087 lcd.printf("It is polling with the selected id."); 00088 00089 // Set url 00090 std::stringstream ss_url; 00091 std::string s_url(URL); 00092 00093 ss_url << s_url << "?app=" << app_id << "&id=" << id; 00094 00095 string url = ss_url.str(); 00096 00097 pc.printf("%s\r\n",url.c_str()); 00098 00099 while(1){ 00100 pc.printf("\r\n----- HTTPS GET request -----\r\n"); 00101 HttpsRequest* get_req = new HttpsRequest(network, SSL_CA_PEM, HTTP_GET, url.c_str()); 00102 00103 get_req->set_header("X-Cybozu-API-Token", API_TOKEN); 00104 00105 HttpResponse* get_res = get_req->send(); 00106 00107 pc.printf("\n----- HTTPS GET response [%d]-----\n",get_res->get_status_code()); 00108 00109 if(get_res->get_status_code() == 200){ 00110 pc.printf("\n----- HTTPS GET response 200 -----\n"); 00111 const char* body = get_res->get_body_as_string().c_str(); 00112 00113 pc.printf("%s\r\n",body); 00114 00115 // Response JSON parse 00116 char value[256]; 00117 00118 char* p = j_paser(body,"日付",value); 00119 j_paser(p,"value",value); 00120 printf("date:%s\r\n",value); 00121 00122 lcd.cls(); 00123 lcd.locate(0,3); 00124 lcd.printf("Date:%s",value); 00125 00126 p = j_paser(body,"文字列__1行_",value); 00127 j_paser(p,"value",value); 00128 printf("%s\r\n",value); 00129 00130 lcd.locate(0,14); 00131 lcd.printf("name:%s",value); 00132 00133 // Sound an alert 00134 spkr.period(1.0/5000.0); 00135 spkr=0.5; 00136 wait(1.0); 00137 spkr=0.0; 00138 00139 delete get_req; 00140 00141 break; 00142 } 00143 00144 delete get_req; 00145 00146 wait(10.0); 00147 } 00148 00149 while(fire == 0); 00150 wait(1.0); 00151 } 00152 } 00153
Generated on Wed Jul 13 2022 21:37:50 by
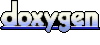