messagepack implementation for embedded systems (mbed / arduino)
Dependents: hello_message_pack
vector_char.hpp
00001 // 00002 // MessagePack for C++ static resolution routine 00003 // 00004 // Copyright (C) 2014-2015 KONDO Takatoshi 00005 // 00006 // Distributed under the Boost Software License, Version 1.0. 00007 // (See accompanying file LICENSE_1_0.txt or copy at 00008 // http://www.boost.org/LICENSE_1_0.txt) 00009 // 00010 #ifndef MSGPACK_TYPE_VECTOR_CHAR_HPP 00011 #define MSGPACK_TYPE_VECTOR_CHAR_HPP 00012 00013 #include "msgpack/versioning.hpp" 00014 #include "msgpack/adaptor/adaptor_base.hpp" 00015 #include "msgpack/adaptor/check_container_size.hpp" 00016 00017 #include <vector> 00018 00019 namespace msgpack { 00020 00021 /// @cond 00022 MSGPACK_API_VERSION_NAMESPACE(v1) { 00023 /// @endcond 00024 00025 namespace adaptor { 00026 00027 template <typename Alloc> 00028 struct convert<std::vector<char, Alloc> > { 00029 msgpack::object const& operator()(msgpack::object const& o, std::vector<char, Alloc>& v) const { 00030 switch (o.type) { 00031 case msgpack::type::BIN: 00032 v.resize(o.via.bin.size); 00033 std::memcpy(&v.front(), o.via.bin.ptr, o.via.bin.size); 00034 break; 00035 case msgpack::type::STR: 00036 v.resize(o.via.str.size); 00037 std::memcpy(&v.front(), o.via.str.ptr, o.via.str.size); 00038 break; 00039 default: 00040 throw msgpack::type_error(); 00041 break; 00042 } 00043 return o; 00044 } 00045 }; 00046 00047 template <typename Alloc> 00048 struct pack<std::vector<char, Alloc> > { 00049 template <typename Stream> 00050 msgpack::packer<Stream> & operator()(msgpack::packer<Stream> & o, const std::vector<char, Alloc>& v) const { 00051 uint32_t size = checked_get_container_size(v.size()); 00052 o.pack_bin(size); 00053 o.pack_bin_body(&v.front(), size); 00054 00055 return o; 00056 } 00057 }; 00058 00059 template <typename Alloc> 00060 struct object<std::vector<char, Alloc> > { 00061 void operator()(msgpack::object& o, const std::vector<char, Alloc>& v) const { 00062 uint32_t size = checked_get_container_size(v.size()); 00063 o.type = msgpack::type::BIN; 00064 o.via.bin.ptr = &v.front(); 00065 o.via.bin.size = size; 00066 } 00067 }; 00068 00069 template <typename Alloc> 00070 struct object_with_zone<std::vector<char, Alloc> > { 00071 void operator()(msgpack::object::with_zone& o, const std::vector<char, Alloc>& v) const { 00072 uint32_t size = checked_get_container_size(v.size()); 00073 o.type = msgpack::type::BIN; 00074 char* ptr = static_cast<char*>(o.zone.allocate_align(size)); 00075 o.via.bin.ptr = ptr; 00076 o.via.bin.size = size; 00077 std::memcpy(ptr, &v.front(), size); 00078 } 00079 }; 00080 00081 } // namespace adaptor 00082 00083 /// @cond 00084 } // MSGPACK_API_VERSION_NAMESPACE(v1) 00085 /// @endcond 00086 00087 } // namespace msgpack 00088 00089 #endif // MSGPACK_TYPE_VECTOR_CHAR_HPP
Generated on Tue Jul 12 2022 22:51:46 by
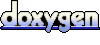