messagepack implementation for embedded systems (mbed / arduino)
Dependents: hello_message_pack
unpack.h
00001 /* 00002 * MessagePack for C unpacking routine 00003 * 00004 * Copyright (C) 2008-2009 FURUHASHI Sadayuki 00005 * 00006 * Distributed under the Boost Software License, Version 1.0. 00007 * (See accompanying file LICENSE_1_0.txt or copy at 00008 * http://www.boost.org/LICENSE_1_0.txt) 00009 */ 00010 #ifndef MSGPACK_UNPACKER_H 00011 #define MSGPACK_UNPACKER_H 00012 00013 #include "zone.h" 00014 #include "object.h" 00015 #include <string.h> 00016 00017 #ifdef __cplusplus 00018 extern "C" { 00019 #endif 00020 00021 00022 /** 00023 * @defgroup msgpack_unpack Deserializer 00024 * @ingroup msgpack 00025 * @{ 00026 */ 00027 00028 typedef struct msgpack_unpacked { 00029 msgpack_zone* zone; 00030 msgpack_object data; 00031 } msgpack_unpacked; 00032 00033 typedef enum { 00034 MSGPACK_UNPACK_SUCCESS = 2, 00035 MSGPACK_UNPACK_EXTRA_BYTES = 1, 00036 MSGPACK_UNPACK_CONTINUE = 0, 00037 MSGPACK_UNPACK_PARSE_ERROR = -1, 00038 MSGPACK_UNPACK_NOMEM_ERROR = -2 00039 } msgpack_unpack_return; 00040 00041 00042 MSGPACK_DLLEXPORT 00043 msgpack_unpack_return 00044 msgpack_unpack_next(msgpack_unpacked* result, 00045 const char* data, size_t len, size_t* off); 00046 00047 /** @} */ 00048 00049 00050 /** 00051 * @defgroup msgpack_unpacker Streaming deserializer 00052 * @ingroup msgpack 00053 * @{ 00054 */ 00055 00056 typedef struct msgpack_unpacker { 00057 char* buffer; 00058 size_t used; 00059 size_t free; 00060 size_t off; 00061 size_t parsed; 00062 msgpack_zone* z; 00063 size_t initial_buffer_size; 00064 void* ctx; 00065 } msgpack_unpacker; 00066 00067 00068 #ifndef MSGPACK_UNPACKER_INIT_BUFFER_SIZE 00069 #define MSGPACK_UNPACKER_INIT_BUFFER_SIZE (64*1024) 00070 #endif 00071 00072 /** 00073 * Initializes a streaming deserializer. 00074 * The initialized deserializer must be destroyed by msgpack_unpacker_destroy(msgpack_unpacker*). 00075 */ 00076 MSGPACK_DLLEXPORT 00077 bool msgpack_unpacker_init(msgpack_unpacker* mpac, size_t initial_buffer_size); 00078 00079 /** 00080 * Destroys a streaming deserializer initialized by msgpack_unpacker_init(msgpack_unpacker*, size_t). 00081 */ 00082 MSGPACK_DLLEXPORT 00083 void msgpack_unpacker_destroy(msgpack_unpacker* mpac); 00084 00085 00086 /** 00087 * Creates a streaming deserializer. 00088 * The created deserializer must be destroyed by msgpack_unpacker_free(msgpack_unpacker*). 00089 */ 00090 MSGPACK_DLLEXPORT 00091 msgpack_unpacker* msgpack_unpacker_new(size_t initial_buffer_size); 00092 00093 /** 00094 * Frees a streaming deserializer created by msgpack_unpacker_new(size_t). 00095 */ 00096 MSGPACK_DLLEXPORT 00097 void msgpack_unpacker_free(msgpack_unpacker* mpac); 00098 00099 00100 #ifndef MSGPACK_UNPACKER_RESERVE_SIZE 00101 #define MSGPACK_UNPACKER_RESERVE_SIZE (32*1024) 00102 #endif 00103 00104 /** 00105 * Reserves free space of the internal buffer. 00106 * Use this function to fill the internal buffer with 00107 * msgpack_unpacker_buffer(msgpack_unpacker*), 00108 * msgpack_unpacker_buffer_capacity(const msgpack_unpacker*) and 00109 * msgpack_unpacker_buffer_consumed(msgpack_unpacker*). 00110 */ 00111 static inline bool msgpack_unpacker_reserve_buffer(msgpack_unpacker* mpac, size_t size); 00112 00113 /** 00114 * Gets pointer to the free space of the internal buffer. 00115 * Use this function to fill the internal buffer with 00116 * msgpack_unpacker_reserve_buffer(msgpack_unpacker*, size_t), 00117 * msgpack_unpacker_buffer_capacity(const msgpack_unpacker*) and 00118 * msgpack_unpacker_buffer_consumed(msgpack_unpacker*). 00119 */ 00120 static inline char* msgpack_unpacker_buffer(msgpack_unpacker* mpac); 00121 00122 /** 00123 * Gets size of the free space of the internal buffer. 00124 * Use this function to fill the internal buffer with 00125 * msgpack_unpacker_reserve_buffer(msgpack_unpacker*, size_t), 00126 * msgpack_unpacker_buffer(const msgpack_unpacker*) and 00127 * msgpack_unpacker_buffer_consumed(msgpack_unpacker*). 00128 */ 00129 static inline size_t msgpack_unpacker_buffer_capacity(const msgpack_unpacker* mpac); 00130 00131 /** 00132 * Notifies the deserializer that the internal buffer filled. 00133 * Use this function to fill the internal buffer with 00134 * msgpack_unpacker_reserve_buffer(msgpack_unpacker*, size_t), 00135 * msgpack_unpacker_buffer(msgpack_unpacker*) and 00136 * msgpack_unpacker_buffer_capacity(const msgpack_unpacker*). 00137 */ 00138 static inline void msgpack_unpacker_buffer_consumed(msgpack_unpacker* mpac, size_t size); 00139 00140 00141 /** 00142 * Deserializes one object. 00143 * Returns true if it successes. Otherwise false is returned. 00144 * @param pac pointer to an initialized msgpack_unpacked object. 00145 */ 00146 MSGPACK_DLLEXPORT 00147 msgpack_unpack_return msgpack_unpacker_next(msgpack_unpacker* mpac, msgpack_unpacked* pac); 00148 00149 /** 00150 * Initializes a msgpack_unpacked object. 00151 * The initialized object must be destroyed by msgpack_unpacked_destroy(msgpack_unpacker*). 00152 * Use the object with msgpack_unpacker_next(msgpack_unpacker*, msgpack_unpacked*) or 00153 * msgpack_unpack_next(msgpack_unpacked*, const char*, size_t, size_t*). 00154 */ 00155 static inline void msgpack_unpacked_init(msgpack_unpacked* result); 00156 00157 /** 00158 * Destroys a streaming deserializer initialized by msgpack_unpacked(). 00159 */ 00160 static inline void msgpack_unpacked_destroy(msgpack_unpacked* result); 00161 00162 /** 00163 * Releases the memory zone from msgpack_unpacked object. 00164 * The released zone must be freed by msgpack_zone_free(msgpack_zone*). 00165 */ 00166 static inline msgpack_zone* msgpack_unpacked_release_zone(msgpack_unpacked* result); 00167 00168 00169 MSGPACK_DLLEXPORT 00170 int msgpack_unpacker_execute(msgpack_unpacker* mpac); 00171 00172 MSGPACK_DLLEXPORT 00173 msgpack_object msgpack_unpacker_data(msgpack_unpacker* mpac); 00174 00175 MSGPACK_DLLEXPORT 00176 msgpack_zone* msgpack_unpacker_release_zone(msgpack_unpacker* mpac); 00177 00178 MSGPACK_DLLEXPORT 00179 void msgpack_unpacker_reset_zone(msgpack_unpacker* mpac); 00180 00181 MSGPACK_DLLEXPORT 00182 void msgpack_unpacker_reset(msgpack_unpacker* mpac); 00183 00184 static inline size_t msgpack_unpacker_message_size(const msgpack_unpacker* mpac); 00185 00186 00187 /** @} */ 00188 00189 00190 // obsolete 00191 MSGPACK_DLLEXPORT 00192 msgpack_unpack_return 00193 msgpack_unpack(const char* data, size_t len, size_t* off, 00194 msgpack_zone* result_zone, msgpack_object* result); 00195 00196 00197 00198 00199 static inline size_t msgpack_unpacker_parsed_size(const msgpack_unpacker* mpac); 00200 00201 MSGPACK_DLLEXPORT 00202 bool msgpack_unpacker_flush_zone(msgpack_unpacker* mpac); 00203 00204 MSGPACK_DLLEXPORT 00205 bool msgpack_unpacker_expand_buffer(msgpack_unpacker* mpac, size_t size); 00206 00207 static inline bool msgpack_unpacker_reserve_buffer(msgpack_unpacker* mpac, size_t size) 00208 { 00209 if(mpac->free >= size) { return true; } 00210 return msgpack_unpacker_expand_buffer(mpac, size); 00211 } 00212 00213 static inline char* msgpack_unpacker_buffer(msgpack_unpacker* mpac) 00214 { 00215 return mpac->buffer + mpac->used; 00216 } 00217 00218 static inline size_t msgpack_unpacker_buffer_capacity(const msgpack_unpacker* mpac) 00219 { 00220 return mpac->free; 00221 } 00222 00223 static inline void msgpack_unpacker_buffer_consumed(msgpack_unpacker* mpac, size_t size) 00224 { 00225 mpac->used += size; 00226 mpac->free -= size; 00227 } 00228 00229 static inline size_t msgpack_unpacker_message_size(const msgpack_unpacker* mpac) 00230 { 00231 return mpac->parsed - mpac->off + mpac->used; 00232 } 00233 00234 static inline size_t msgpack_unpacker_parsed_size(const msgpack_unpacker* mpac) 00235 { 00236 return mpac->parsed; 00237 } 00238 00239 00240 static inline void msgpack_unpacked_init(msgpack_unpacked* result) 00241 { 00242 memset(result, 0, sizeof(msgpack_unpacked)); 00243 } 00244 00245 static inline void msgpack_unpacked_destroy(msgpack_unpacked* result) 00246 { 00247 if(result->zone != NULL) { 00248 msgpack_zone_free(result->zone); 00249 result->zone = NULL; 00250 memset(&result->data, 0, sizeof(msgpack_object)); 00251 } 00252 } 00253 00254 static inline msgpack_zone* msgpack_unpacked_release_zone(msgpack_unpacked* result) 00255 { 00256 if(result->zone != NULL) { 00257 msgpack_zone* z = result->zone; 00258 result->zone = NULL; 00259 return z; 00260 } 00261 return NULL; 00262 } 00263 00264 00265 #ifdef __cplusplus 00266 } 00267 #endif 00268 00269 #endif /* msgpack/unpack.h */
Generated on Tue Jul 12 2022 22:51:46 by
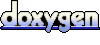