messagepack implementation for embedded systems (mbed / arduino)
Dependents: hello_message_pack
set.hpp
00001 // 00002 // MessagePack for C++ static resolution routine 00003 // 00004 // Copyright (C) 2008-2015 FURUHASHI Sadayuki 00005 // 00006 // Distributed under the Boost Software License, Version 1.0. 00007 // (See accompanying file LICENSE_1_0.txt or copy at 00008 // http://www.boost.org/LICENSE_1_0.txt) 00009 // 00010 #ifndef MSGPACK_TYPE_SET_HPP 00011 #define MSGPACK_TYPE_SET_HPP 00012 00013 #include "msgpack/versioning.hpp" 00014 #include "msgpack/adaptor/adaptor_base.hpp" 00015 #include "msgpack/adaptor/check_container_size.hpp" 00016 00017 #include <set> 00018 00019 namespace msgpack { 00020 00021 /// @cond 00022 MSGPACK_API_VERSION_NAMESPACE(v1) { 00023 /// @endcond 00024 00025 namespace adaptor { 00026 00027 #if !defined(MSGPACK_USE_CPP03) 00028 00029 template <typename T, typename Compare, typename Alloc> 00030 struct as<std::set<T, Compare, Alloc>, typename std::enable_if<msgpack::has_as<T>::value>::type> { 00031 std::set<T, Compare, Alloc> operator()(msgpack::object const& o) const { 00032 if (o.type != msgpack::type::ARRAY) { throw msgpack::type_error(); } 00033 msgpack::object* p = o.via.array.ptr + o.via.array.size; 00034 msgpack::object* const pbegin = o.via.array.ptr; 00035 std::set<T, Compare, Alloc> v; 00036 while (p > pbegin) { 00037 --p; 00038 v.insert(p->as<T>()); 00039 } 00040 return v; 00041 } 00042 }; 00043 00044 #endif // !defined(MSGPACK_USE_CPP03) 00045 00046 template <typename T, typename Compare, typename Alloc> 00047 struct convert<std::set<T, Compare, Alloc> > { 00048 msgpack::object const& operator()(msgpack::object const& o, std::set<T, Compare, Alloc>& v) const { 00049 if (o.type != msgpack::type::ARRAY) { throw msgpack::type_error(); } 00050 msgpack::object* p = o.via.array.ptr + o.via.array.size; 00051 msgpack::object* const pbegin = o.via.array.ptr; 00052 std::set<T, Compare, Alloc> tmp; 00053 while (p > pbegin) { 00054 --p; 00055 tmp.insert(p->as<T>()); 00056 } 00057 #if __cplusplus >= 201103L 00058 v = std::move(tmp); 00059 #else 00060 tmp.swap(v); 00061 #endif 00062 return o; 00063 } 00064 }; 00065 00066 template <typename T, typename Compare, typename Alloc> 00067 struct pack<std::set<T, Compare, Alloc> > { 00068 template <typename Stream> 00069 msgpack::packer<Stream> & operator()(msgpack::packer<Stream> & o, const std::set<T, Compare, Alloc>& v) const { 00070 uint32_t size = checked_get_container_size(v.size()); 00071 o.pack_array(size); 00072 for (typename std::set<T, Compare, Alloc>::const_iterator it(v.begin()), it_end(v.end()); 00073 it != it_end; ++it) { 00074 o.pack(*it); 00075 } 00076 return o; 00077 } 00078 }; 00079 00080 template <typename T, typename Compare, typename Alloc> 00081 struct object_with_zone<std::set<T, Compare, Alloc> > { 00082 void operator()(msgpack::object::with_zone& o, const std::set<T, Compare, Alloc>& v) const { 00083 o.type = msgpack::type::ARRAY; 00084 if (v.empty()) { 00085 o.via.array.ptr = nullptr; 00086 o.via.array.size = 0; 00087 } 00088 else { 00089 uint32_t size = checked_get_container_size(v.size()); 00090 msgpack::object* p = static_cast<msgpack::object*>(o.zone.allocate_align(sizeof(msgpack::object)*size)); 00091 msgpack::object* const pend = p + size; 00092 o.via.array.ptr = p; 00093 o.via.array.size = size; 00094 typename std::set<T, Compare, Alloc>::const_iterator it(v.begin()); 00095 do { 00096 *p = msgpack::object(*it, o.zone); 00097 ++p; 00098 ++it; 00099 } while(p < pend); 00100 } 00101 } 00102 }; 00103 00104 #if !defined(MSGPACK_USE_CPP03) 00105 00106 template <typename T, typename Compare, typename Alloc> 00107 struct as<std::multiset<T, Compare, Alloc>, typename std::enable_if<msgpack::has_as<T>::value>::type> { 00108 std::multiset<T, Compare, Alloc> operator()(msgpack::object const& o) const { 00109 if (o.type != msgpack::type::ARRAY) { throw msgpack::type_error(); } 00110 msgpack::object* p = o.via.array.ptr + o.via.array.size; 00111 msgpack::object* const pbegin = o.via.array.ptr; 00112 std::multiset<T, Compare, Alloc> v; 00113 while (p > pbegin) { 00114 --p; 00115 v.insert(p->as<T>()); 00116 } 00117 return v; 00118 } 00119 }; 00120 00121 #endif // !defined(MSGPACK_USE_CPP03) 00122 00123 template <typename T, typename Compare, typename Alloc> 00124 struct convert<std::multiset<T, Compare, Alloc> > { 00125 msgpack::object const& operator()(msgpack::object const& o, std::multiset<T, Compare, Alloc>& v) const { 00126 if (o.type != msgpack::type::ARRAY) { throw msgpack::type_error(); } 00127 msgpack::object* p = o.via.array.ptr + o.via.array.size; 00128 msgpack::object* const pbegin = o.via.array.ptr; 00129 std::multiset<T, Compare, Alloc> tmp; 00130 while (p > pbegin) { 00131 --p; 00132 tmp.insert(p->as<T>()); 00133 } 00134 #if __cplusplus >= 201103L 00135 v = std::move(tmp); 00136 #else 00137 tmp.swap(v); 00138 #endif 00139 return o; 00140 } 00141 }; 00142 00143 template <typename T, typename Compare, typename Alloc> 00144 struct pack<std::multiset<T, Compare, Alloc> > { 00145 template <typename Stream> 00146 msgpack::packer<Stream> & operator()(msgpack::packer<Stream> & o, const std::multiset<T, Compare, Alloc>& v) const { 00147 uint32_t size = checked_get_container_size(v.size()); 00148 o.pack_array(size); 00149 for (typename std::multiset<T, Compare, Alloc>::const_iterator it(v.begin()), it_end(v.end()); 00150 it != it_end; ++it) { 00151 o.pack(*it); 00152 } 00153 return o; 00154 } 00155 }; 00156 00157 template <typename T, typename Compare, typename Alloc> 00158 struct object_with_zone<std::multiset<T, Compare, Alloc> > { 00159 void operator()(msgpack::object::with_zone& o, const std::multiset<T, Compare, Alloc>& v) const { 00160 o.type = msgpack::type::ARRAY; 00161 if (v.empty()) { 00162 o.via.array.ptr = nullptr; 00163 o.via.array.size = 0; 00164 } else { 00165 uint32_t size = checked_get_container_size(v.size()); 00166 msgpack::object* p = static_cast<msgpack::object*>(o.zone.allocate_align(sizeof(msgpack::object)*size)); 00167 msgpack::object* const pend = p + size; 00168 o.via.array.ptr = p; 00169 o.via.array.size = size; 00170 typename std::multiset<T, Compare, Alloc>::const_iterator it(v.begin()); 00171 do { 00172 *p = msgpack::object(*it, o.zone); 00173 ++p; 00174 ++it; 00175 } while(p < pend); 00176 } 00177 } 00178 }; 00179 00180 } // namespace adaptor 00181 00182 /// @cond 00183 } // MSGPACK_API_VERSION_NAMESPACE(v1) 00184 /// @endcond 00185 00186 } // namespace msgpack 00187 00188 #endif // MSGPACK_TYPE_SET_HPP
Generated on Tue Jul 12 2022 22:51:46 by
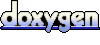