messagepack implementation for embedded systems (mbed / arduino)
Dependents: hello_message_pack
pack_template.h
00001 /* 00002 * MessagePack packing routine template 00003 * 00004 * Copyright (C) 2008-2010 FURUHASHI Sadayuki 00005 * 00006 * Distributed under the Boost Software License, Version 1.0. 00007 * (See accompanying file LICENSE_1_0.txt or copy at 00008 * http://www.boost.org/LICENSE_1_0.txt) 00009 */ 00010 00011 #if MSGPACK_ENDIAN_LITTLE_BYTE 00012 #define TAKE8_8(d) ((uint8_t*)&d)[0] 00013 #define TAKE8_16(d) ((uint8_t*)&d)[0] 00014 #define TAKE8_32(d) ((uint8_t*)&d)[0] 00015 #define TAKE8_64(d) ((uint8_t*)&d)[0] 00016 #elif MSGPACK_ENDIAN_BIG_BYTE 00017 #define TAKE8_8(d) ((uint8_t*)&d)[0] 00018 #define TAKE8_16(d) ((uint8_t*)&d)[1] 00019 #define TAKE8_32(d) ((uint8_t*)&d)[3] 00020 #define TAKE8_64(d) ((uint8_t*)&d)[7] 00021 #else 00022 #error msgpack-c supports only big endian and little endian 00023 #endif 00024 00025 #ifndef msgpack_pack_inline_func 00026 #error msgpack_pack_inline_func template is not defined 00027 #endif 00028 00029 #ifndef msgpack_pack_user 00030 #error msgpack_pack_user type is not defined 00031 #endif 00032 00033 #ifndef msgpack_pack_append_buffer 00034 #error msgpack_pack_append_buffer callback is not defined 00035 #endif 00036 00037 00038 /* 00039 * Integer 00040 */ 00041 00042 #define msgpack_pack_real_uint8(x, d) \ 00043 do { \ 00044 if(d < (1<<7)) { \ 00045 /* fixnum */ \ 00046 msgpack_pack_append_buffer(x, &TAKE8_8(d), 1); \ 00047 } else { \ 00048 /* unsigned 8 */ \ 00049 unsigned char buf[2] = {0xcc, TAKE8_8(d)}; \ 00050 msgpack_pack_append_buffer(x, buf, 2); \ 00051 } \ 00052 } while(0) 00053 00054 #define msgpack_pack_real_uint16(x, d) \ 00055 do { \ 00056 if(d < (1<<7)) { \ 00057 /* fixnum */ \ 00058 msgpack_pack_append_buffer(x, &TAKE8_16(d), 1); \ 00059 } else if(d < (1<<8)) { \ 00060 /* unsigned 8 */ \ 00061 unsigned char buf[2] = {0xcc, TAKE8_16(d)}; \ 00062 msgpack_pack_append_buffer(x, buf, 2); \ 00063 } else { \ 00064 /* unsigned 16 */ \ 00065 unsigned char buf[3]; \ 00066 buf[0] = 0xcd; _msgpack_store16(&buf[1], (uint16_t)d); \ 00067 msgpack_pack_append_buffer(x, buf, 3); \ 00068 } \ 00069 } while(0) 00070 00071 #define msgpack_pack_real_uint32(x, d) \ 00072 do { \ 00073 if(d < (1<<8)) { \ 00074 if(d < (1<<7)) { \ 00075 /* fixnum */ \ 00076 msgpack_pack_append_buffer(x, &TAKE8_32(d), 1); \ 00077 } else { \ 00078 /* unsigned 8 */ \ 00079 unsigned char buf[2] = {0xcc, TAKE8_32(d)}; \ 00080 msgpack_pack_append_buffer(x, buf, 2); \ 00081 } \ 00082 } else { \ 00083 if(d < (1<<16)) { \ 00084 /* unsigned 16 */ \ 00085 unsigned char buf[3]; \ 00086 buf[0] = 0xcd; _msgpack_store16(&buf[1], (uint16_t)d); \ 00087 msgpack_pack_append_buffer(x, buf, 3); \ 00088 } else { \ 00089 /* unsigned 32 */ \ 00090 unsigned char buf[5]; \ 00091 buf[0] = 0xce; _msgpack_store32(&buf[1], (uint32_t)d); \ 00092 msgpack_pack_append_buffer(x, buf, 5); \ 00093 } \ 00094 } \ 00095 } while(0) 00096 00097 #define msgpack_pack_real_uint64(x, d) \ 00098 do { \ 00099 if(d < (1ULL<<8)) { \ 00100 if(d < (1ULL<<7)) { \ 00101 /* fixnum */ \ 00102 msgpack_pack_append_buffer(x, &TAKE8_64(d), 1); \ 00103 } else { \ 00104 /* unsigned 8 */ \ 00105 unsigned char buf[2] = {0xcc, TAKE8_64(d)}; \ 00106 msgpack_pack_append_buffer(x, buf, 2); \ 00107 } \ 00108 } else { \ 00109 if(d < (1ULL<<16)) { \ 00110 /* unsigned 16 */ \ 00111 unsigned char buf[3]; \ 00112 buf[0] = 0xcd; _msgpack_store16(&buf[1], (uint16_t)d); \ 00113 msgpack_pack_append_buffer(x, buf, 3); \ 00114 } else if(d < (1ULL<<32)) { \ 00115 /* unsigned 32 */ \ 00116 unsigned char buf[5]; \ 00117 buf[0] = 0xce; _msgpack_store32(&buf[1], (uint32_t)d); \ 00118 msgpack_pack_append_buffer(x, buf, 5); \ 00119 } else { \ 00120 /* unsigned 64 */ \ 00121 unsigned char buf[9]; \ 00122 buf[0] = 0xcf; _msgpack_store64(&buf[1], d); \ 00123 msgpack_pack_append_buffer(x, buf, 9); \ 00124 } \ 00125 } \ 00126 } while(0) 00127 00128 #define msgpack_pack_real_int8(x, d) \ 00129 do { \ 00130 if(d < -(1<<5)) { \ 00131 /* signed 8 */ \ 00132 unsigned char buf[2] = {0xd0, TAKE8_8(d)}; \ 00133 msgpack_pack_append_buffer(x, buf, 2); \ 00134 } else { \ 00135 /* fixnum */ \ 00136 msgpack_pack_append_buffer(x, &TAKE8_8(d), 1); \ 00137 } \ 00138 } while(0) 00139 00140 #define msgpack_pack_real_int16(x, d) \ 00141 do { \ 00142 if(d < -(1<<5)) { \ 00143 if(d < -(1<<7)) { \ 00144 /* signed 16 */ \ 00145 unsigned char buf[3]; \ 00146 buf[0] = 0xd1; _msgpack_store16(&buf[1], (int16_t)d); \ 00147 msgpack_pack_append_buffer(x, buf, 3); \ 00148 } else { \ 00149 /* signed 8 */ \ 00150 unsigned char buf[2] = {0xd0, TAKE8_16(d)}; \ 00151 msgpack_pack_append_buffer(x, buf, 2); \ 00152 } \ 00153 } else if(d < (1<<7)) { \ 00154 /* fixnum */ \ 00155 msgpack_pack_append_buffer(x, &TAKE8_16(d), 1); \ 00156 } else { \ 00157 if(d < (1<<8)) { \ 00158 /* unsigned 8 */ \ 00159 unsigned char buf[2] = {0xcc, TAKE8_16(d)}; \ 00160 msgpack_pack_append_buffer(x, buf, 2); \ 00161 } else { \ 00162 /* unsigned 16 */ \ 00163 unsigned char buf[3]; \ 00164 buf[0] = 0xcd; _msgpack_store16(&buf[1], (uint16_t)d); \ 00165 msgpack_pack_append_buffer(x, buf, 3); \ 00166 } \ 00167 } \ 00168 } while(0) 00169 00170 #define msgpack_pack_real_int32(x, d) \ 00171 do { \ 00172 if(d < -(1<<5)) { \ 00173 if(d < -(1<<15)) { \ 00174 /* signed 32 */ \ 00175 unsigned char buf[5]; \ 00176 buf[0] = 0xd2; _msgpack_store32(&buf[1], (int32_t)d); \ 00177 msgpack_pack_append_buffer(x, buf, 5); \ 00178 } else if(d < -(1<<7)) { \ 00179 /* signed 16 */ \ 00180 unsigned char buf[3]; \ 00181 buf[0] = 0xd1; _msgpack_store16(&buf[1], (int16_t)d); \ 00182 msgpack_pack_append_buffer(x, buf, 3); \ 00183 } else { \ 00184 /* signed 8 */ \ 00185 unsigned char buf[2] = {0xd0, TAKE8_32(d)}; \ 00186 msgpack_pack_append_buffer(x, buf, 2); \ 00187 } \ 00188 } else if(d < (1<<7)) { \ 00189 /* fixnum */ \ 00190 msgpack_pack_append_buffer(x, &TAKE8_32(d), 1); \ 00191 } else { \ 00192 if(d < (1<<8)) { \ 00193 /* unsigned 8 */ \ 00194 unsigned char buf[2] = {0xcc, TAKE8_32(d)}; \ 00195 msgpack_pack_append_buffer(x, buf, 2); \ 00196 } else if(d < (1<<16)) { \ 00197 /* unsigned 16 */ \ 00198 unsigned char buf[3]; \ 00199 buf[0] = 0xcd; _msgpack_store16(&buf[1], (uint16_t)d); \ 00200 msgpack_pack_append_buffer(x, buf, 3); \ 00201 } else { \ 00202 /* unsigned 32 */ \ 00203 unsigned char buf[5]; \ 00204 buf[0] = 0xce; _msgpack_store32(&buf[1], (uint32_t)d); \ 00205 msgpack_pack_append_buffer(x, buf, 5); \ 00206 } \ 00207 } \ 00208 } while(0) 00209 00210 #define msgpack_pack_real_int64(x, d) \ 00211 do { \ 00212 if(d < -(1LL<<5)) { \ 00213 if(d < -(1LL<<15)) { \ 00214 if(d < -(1LL<<31)) { \ 00215 /* signed 64 */ \ 00216 unsigned char buf[9]; \ 00217 buf[0] = 0xd3; _msgpack_store64(&buf[1], d); \ 00218 msgpack_pack_append_buffer(x, buf, 9); \ 00219 } else { \ 00220 /* signed 32 */ \ 00221 unsigned char buf[5]; \ 00222 buf[0] = 0xd2; _msgpack_store32(&buf[1], (int32_t)d); \ 00223 msgpack_pack_append_buffer(x, buf, 5); \ 00224 } \ 00225 } else { \ 00226 if(d < -(1<<7)) { \ 00227 /* signed 16 */ \ 00228 unsigned char buf[3]; \ 00229 buf[0] = 0xd1; _msgpack_store16(&buf[1], (int16_t)d); \ 00230 msgpack_pack_append_buffer(x, buf, 3); \ 00231 } else { \ 00232 /* signed 8 */ \ 00233 unsigned char buf[2] = {0xd0, TAKE8_64(d)}; \ 00234 msgpack_pack_append_buffer(x, buf, 2); \ 00235 } \ 00236 } \ 00237 } else if(d < (1<<7)) { \ 00238 /* fixnum */ \ 00239 msgpack_pack_append_buffer(x, &TAKE8_64(d), 1); \ 00240 } else { \ 00241 if(d < (1LL<<16)) { \ 00242 if(d < (1<<8)) { \ 00243 /* unsigned 8 */ \ 00244 unsigned char buf[2] = {0xcc, TAKE8_64(d)}; \ 00245 msgpack_pack_append_buffer(x, buf, 2); \ 00246 } else { \ 00247 /* unsigned 16 */ \ 00248 unsigned char buf[3]; \ 00249 buf[0] = 0xcd; _msgpack_store16(&buf[1], (uint16_t)d); \ 00250 msgpack_pack_append_buffer(x, buf, 3); \ 00251 } \ 00252 } else { \ 00253 if(d < (1LL<<32)) { \ 00254 /* unsigned 32 */ \ 00255 unsigned char buf[5]; \ 00256 buf[0] = 0xce; _msgpack_store32(&buf[1], (uint32_t)d); \ 00257 msgpack_pack_append_buffer(x, buf, 5); \ 00258 } else { \ 00259 /* unsigned 64 */ \ 00260 unsigned char buf[9]; \ 00261 buf[0] = 0xcf; _msgpack_store64(&buf[1], d); \ 00262 msgpack_pack_append_buffer(x, buf, 9); \ 00263 } \ 00264 } \ 00265 } \ 00266 } while(0) 00267 00268 00269 #ifdef msgpack_pack_inline_func_fixint 00270 00271 msgpack_pack_inline_func_fixint(_uint8)(msgpack_pack_user x, uint8_t d) 00272 { 00273 unsigned char buf[2] = {0xcc, TAKE8_8(d)}; 00274 msgpack_pack_append_buffer(x, buf, 2); 00275 } 00276 00277 msgpack_pack_inline_func_fixint(_uint16)(msgpack_pack_user x, uint16_t d) 00278 { 00279 unsigned char buf[3]; 00280 buf[0] = 0xcd; _msgpack_store16(&buf[1], d); 00281 msgpack_pack_append_buffer(x, buf, 3); 00282 } 00283 00284 msgpack_pack_inline_func_fixint(_uint32)(msgpack_pack_user x, uint32_t d) 00285 { 00286 unsigned char buf[5]; 00287 buf[0] = 0xce; _msgpack_store32(&buf[1], d); 00288 msgpack_pack_append_buffer(x, buf, 5); 00289 } 00290 00291 msgpack_pack_inline_func_fixint(_uint64)(msgpack_pack_user x, uint64_t d) 00292 { 00293 unsigned char buf[9]; 00294 buf[0] = 0xcf; _msgpack_store64(&buf[1], d); 00295 msgpack_pack_append_buffer(x, buf, 9); 00296 } 00297 00298 msgpack_pack_inline_func_fixint(_int8)(msgpack_pack_user x, int8_t d) 00299 { 00300 unsigned char buf[2] = {0xd0, TAKE8_8(d)}; 00301 msgpack_pack_append_buffer(x, buf, 2); 00302 } 00303 00304 msgpack_pack_inline_func_fixint(_int16)(msgpack_pack_user x, int16_t d) 00305 { 00306 unsigned char buf[3]; 00307 buf[0] = 0xd1; _msgpack_store16(&buf[1], d); 00308 msgpack_pack_append_buffer(x, buf, 3); 00309 } 00310 00311 msgpack_pack_inline_func_fixint(_int32)(msgpack_pack_user x, int32_t d) 00312 { 00313 unsigned char buf[5]; 00314 buf[0] = 0xd2; _msgpack_store32(&buf[1], d); 00315 msgpack_pack_append_buffer(x, buf, 5); 00316 } 00317 00318 msgpack_pack_inline_func_fixint(_int64)(msgpack_pack_user x, int64_t d) 00319 { 00320 unsigned char buf[9]; 00321 buf[0] = 0xd3; _msgpack_store64(&buf[1], d); 00322 msgpack_pack_append_buffer(x, buf, 9); 00323 } 00324 00325 #undef msgpack_pack_inline_func_fixint 00326 #endif 00327 00328 00329 msgpack_pack_inline_func(_uint8)(msgpack_pack_user x, uint8_t d) 00330 { 00331 msgpack_pack_real_uint8(x, d); 00332 } 00333 00334 msgpack_pack_inline_func(_uint16)(msgpack_pack_user x, uint16_t d) 00335 { 00336 msgpack_pack_real_uint16(x, d); 00337 } 00338 00339 msgpack_pack_inline_func(_uint32)(msgpack_pack_user x, uint32_t d) 00340 { 00341 msgpack_pack_real_uint32(x, d); 00342 } 00343 00344 msgpack_pack_inline_func(_uint64)(msgpack_pack_user x, uint64_t d) 00345 { 00346 msgpack_pack_real_uint64(x, d); 00347 } 00348 00349 msgpack_pack_inline_func(_int8)(msgpack_pack_user x, int8_t d) 00350 { 00351 msgpack_pack_real_int8(x, d); 00352 } 00353 00354 msgpack_pack_inline_func(_int16)(msgpack_pack_user x, int16_t d) 00355 { 00356 msgpack_pack_real_int16(x, d); 00357 } 00358 00359 msgpack_pack_inline_func(_int32)(msgpack_pack_user x, int32_t d) 00360 { 00361 msgpack_pack_real_int32(x, d); 00362 } 00363 00364 msgpack_pack_inline_func(_int64)(msgpack_pack_user x, int64_t d) 00365 { 00366 msgpack_pack_real_int64(x, d); 00367 } 00368 00369 msgpack_pack_inline_func(_char)(msgpack_pack_user x, char d) 00370 { 00371 #if defined(CHAR_MIN) 00372 #if CHAR_MIN < 0 00373 msgpack_pack_real_int8(x, d); 00374 #else 00375 msgpack_pack_real_uint8(x, d); 00376 #endif 00377 #else 00378 #error CHAR_MIN is not defined 00379 #endif 00380 } 00381 00382 msgpack_pack_inline_func(_signed_char)(msgpack_pack_user x, signed char d) 00383 { 00384 msgpack_pack_real_int8(x, d); 00385 } 00386 00387 msgpack_pack_inline_func(_unsigned_char)(msgpack_pack_user x, unsigned char d) 00388 { 00389 msgpack_pack_real_uint8(x, d); 00390 } 00391 00392 #ifdef msgpack_pack_inline_func_cint 00393 00394 msgpack_pack_inline_func_cint(_short)(msgpack_pack_user x, short d) 00395 { 00396 #if defined(SIZEOF_SHORT) 00397 #if SIZEOF_SHORT == 2 00398 msgpack_pack_real_int16(x, d); 00399 #elif SIZEOF_SHORT == 4 00400 msgpack_pack_real_int32(x, d); 00401 #else 00402 msgpack_pack_real_int64(x, d); 00403 #endif 00404 00405 #elif defined(SHRT_MAX) 00406 #if SHRT_MAX == 0x7fff 00407 msgpack_pack_real_int16(x, d); 00408 #elif SHRT_MAX == 0x7fffffff 00409 msgpack_pack_real_int32(x, d); 00410 #else 00411 msgpack_pack_real_int64(x, d); 00412 #endif 00413 00414 #else 00415 if(sizeof(short) == 2) { 00416 msgpack_pack_real_int16(x, d); 00417 } else if(sizeof(short) == 4) { 00418 msgpack_pack_real_int32(x, d); 00419 } else { 00420 msgpack_pack_real_int64(x, d); 00421 } 00422 #endif 00423 } 00424 00425 msgpack_pack_inline_func_cint(_int)(msgpack_pack_user x, int d) 00426 { 00427 #if defined(SIZEOF_INT) 00428 #if SIZEOF_INT == 2 00429 msgpack_pack_real_int16(x, d); 00430 #elif SIZEOF_INT == 4 00431 msgpack_pack_real_int32(x, d); 00432 #else 00433 msgpack_pack_real_int64(x, d); 00434 #endif 00435 00436 #elif defined(INT_MAX) 00437 #if INT_MAX == 0x7fff 00438 msgpack_pack_real_int16(x, d); 00439 #elif INT_MAX == 0x7fffffff 00440 msgpack_pack_real_int32(x, d); 00441 #else 00442 msgpack_pack_real_int64(x, d); 00443 #endif 00444 00445 #else 00446 if(sizeof(int) == 2) { 00447 msgpack_pack_real_int16(x, d); 00448 } else if(sizeof(int) == 4) { 00449 msgpack_pack_real_int32(x, d); 00450 } else { 00451 msgpack_pack_real_int64(x, d); 00452 } 00453 #endif 00454 } 00455 00456 msgpack_pack_inline_func_cint(_long)(msgpack_pack_user x, long d) 00457 { 00458 #if defined(SIZEOF_LONG) 00459 #if SIZEOF_LONG == 2 00460 msgpack_pack_real_int16(x, d); 00461 #elif SIZEOF_LONG == 4 00462 msgpack_pack_real_int32(x, d); 00463 #else 00464 msgpack_pack_real_int64(x, d); 00465 #endif 00466 00467 #elif defined(LONG_MAX) 00468 #if LONG_MAX == 0x7fffL 00469 msgpack_pack_real_int16(x, d); 00470 #elif LONG_MAX == 0x7fffffffL 00471 msgpack_pack_real_int32(x, d); 00472 #else 00473 msgpack_pack_real_int64(x, d); 00474 #endif 00475 00476 #else 00477 if(sizeof(long) == 2) { 00478 msgpack_pack_real_int16(x, d); 00479 } else if(sizeof(long) == 4) { 00480 msgpack_pack_real_int32(x, d); 00481 } else { 00482 msgpack_pack_real_int64(x, d); 00483 } 00484 #endif 00485 } 00486 00487 msgpack_pack_inline_func_cint(_long_long)(msgpack_pack_user x, long long d) 00488 { 00489 #if defined(SIZEOF_LONG_LONG) 00490 #if SIZEOF_LONG_LONG == 2 00491 msgpack_pack_real_int16(x, d); 00492 #elif SIZEOF_LONG_LONG == 4 00493 msgpack_pack_real_int32(x, d); 00494 #else 00495 msgpack_pack_real_int64(x, d); 00496 #endif 00497 00498 #elif defined(LLONG_MAX) 00499 #if LLONG_MAX == 0x7fffL 00500 msgpack_pack_real_int16(x, d); 00501 #elif LLONG_MAX == 0x7fffffffL 00502 msgpack_pack_real_int32(x, d); 00503 #else 00504 msgpack_pack_real_int64(x, d); 00505 #endif 00506 00507 #else 00508 if(sizeof(long long) == 2) { 00509 msgpack_pack_real_int16(x, d); 00510 } else if(sizeof(long long) == 4) { 00511 msgpack_pack_real_int32(x, d); 00512 } else { 00513 msgpack_pack_real_int64(x, d); 00514 } 00515 #endif 00516 } 00517 00518 msgpack_pack_inline_func_cint(_unsigned_short)(msgpack_pack_user x, unsigned short d) 00519 { 00520 #if defined(SIZEOF_SHORT) 00521 #if SIZEOF_SHORT == 2 00522 msgpack_pack_real_uint16(x, d); 00523 #elif SIZEOF_SHORT == 4 00524 msgpack_pack_real_uint32(x, d); 00525 #else 00526 msgpack_pack_real_uint64(x, d); 00527 #endif 00528 00529 #elif defined(USHRT_MAX) 00530 #if USHRT_MAX == 0xffffU 00531 msgpack_pack_real_uint16(x, d); 00532 #elif USHRT_MAX == 0xffffffffU 00533 msgpack_pack_real_uint32(x, d); 00534 #else 00535 msgpack_pack_real_uint64(x, d); 00536 #endif 00537 00538 #else 00539 if(sizeof(unsigned short) == 2) { 00540 msgpack_pack_real_uint16(x, d); 00541 } else if(sizeof(unsigned short) == 4) { 00542 msgpack_pack_real_uint32(x, d); 00543 } else { 00544 msgpack_pack_real_uint64(x, d); 00545 } 00546 #endif 00547 } 00548 00549 msgpack_pack_inline_func_cint(_unsigned_int)(msgpack_pack_user x, unsigned int d) 00550 { 00551 #if defined(SIZEOF_INT) 00552 #if SIZEOF_INT == 2 00553 msgpack_pack_real_uint16(x, d); 00554 #elif SIZEOF_INT == 4 00555 msgpack_pack_real_uint32(x, d); 00556 #else 00557 msgpack_pack_real_uint64(x, d); 00558 #endif 00559 00560 #elif defined(UINT_MAX) 00561 #if UINT_MAX == 0xffffU 00562 msgpack_pack_real_uint16(x, d); 00563 #elif UINT_MAX == 0xffffffffU 00564 msgpack_pack_real_uint32(x, d); 00565 #else 00566 msgpack_pack_real_uint64(x, d); 00567 #endif 00568 00569 #else 00570 if(sizeof(unsigned int) == 2) { 00571 msgpack_pack_real_uint16(x, d); 00572 } else if(sizeof(unsigned int) == 4) { 00573 msgpack_pack_real_uint32(x, d); 00574 } else { 00575 msgpack_pack_real_uint64(x, d); 00576 } 00577 #endif 00578 } 00579 00580 msgpack_pack_inline_func_cint(_unsigned_long)(msgpack_pack_user x, unsigned long d) 00581 { 00582 #if defined(SIZEOF_LONG) 00583 #if SIZEOF_LONG == 2 00584 msgpack_pack_real_uint16(x, d); 00585 #elif SIZEOF_LONG == 4 00586 msgpack_pack_real_uint32(x, d); 00587 #else 00588 msgpack_pack_real_uint64(x, d); 00589 #endif 00590 00591 #elif defined(ULONG_MAX) 00592 #if ULONG_MAX == 0xffffUL 00593 msgpack_pack_real_uint16(x, d); 00594 #elif ULONG_MAX == 0xffffffffUL 00595 msgpack_pack_real_uint32(x, d); 00596 #else 00597 msgpack_pack_real_uint64(x, d); 00598 #endif 00599 00600 #else 00601 if(sizeof(unsigned long) == 2) { 00602 msgpack_pack_real_uint16(x, d); 00603 } else if(sizeof(unsigned long) == 4) { 00604 msgpack_pack_real_uint32(x, d); 00605 } else { 00606 msgpack_pack_real_uint64(x, d); 00607 } 00608 #endif 00609 } 00610 00611 msgpack_pack_inline_func_cint(_unsigned_long_long)(msgpack_pack_user x, unsigned long long d) 00612 { 00613 #if defined(SIZEOF_LONG_LONG) 00614 #if SIZEOF_LONG_LONG == 2 00615 msgpack_pack_real_uint16(x, d); 00616 #elif SIZEOF_LONG_LONG == 4 00617 msgpack_pack_real_uint32(x, d); 00618 #else 00619 msgpack_pack_real_uint64(x, d); 00620 #endif 00621 00622 #elif defined(ULLONG_MAX) 00623 #if ULLONG_MAX == 0xffffUL 00624 msgpack_pack_real_uint16(x, d); 00625 #elif ULLONG_MAX == 0xffffffffUL 00626 msgpack_pack_real_uint32(x, d); 00627 #else 00628 msgpack_pack_real_uint64(x, d); 00629 #endif 00630 00631 #else 00632 if(sizeof(unsigned long long) == 2) { 00633 msgpack_pack_real_uint16(x, d); 00634 } else if(sizeof(unsigned long long) == 4) { 00635 msgpack_pack_real_uint32(x, d); 00636 } else { 00637 msgpack_pack_real_uint64(x, d); 00638 } 00639 #endif 00640 } 00641 00642 #undef msgpack_pack_inline_func_cint 00643 #endif 00644 00645 00646 00647 /* 00648 * Float 00649 */ 00650 00651 msgpack_pack_inline_func(_float)(msgpack_pack_user x, float d) 00652 { 00653 unsigned char buf[5]; 00654 union { float f; uint32_t i; } mem; 00655 mem.f = d; 00656 buf[0] = 0xca; _msgpack_store32(&buf[1], mem.i); 00657 msgpack_pack_append_buffer(x, buf, 5); 00658 } 00659 00660 msgpack_pack_inline_func(_double)(msgpack_pack_user x, double d) 00661 { 00662 unsigned char buf[9]; 00663 union { double f; uint64_t i; } mem; 00664 mem.f = d; 00665 buf[0] = 0xcb; 00666 #if defined(TARGET_OS_IPHONE) 00667 // ok 00668 #elif defined(__arm__) && !(__ARM_EABI__) // arm-oabi 00669 // https://github.com/msgpack/msgpack-perl/pull/1 00670 mem.i = (mem.i & 0xFFFFFFFFUL) << 32UL | (mem.i >> 32UL); 00671 #endif 00672 _msgpack_store64(&buf[1], mem.i); 00673 msgpack_pack_append_buffer(x, buf, 9); 00674 } 00675 00676 00677 /* 00678 * Nil 00679 */ 00680 00681 msgpack_pack_inline_func(_nil)(msgpack_pack_user x) 00682 { 00683 static const unsigned char d = 0xc0; 00684 msgpack_pack_append_buffer(x, &d, 1); 00685 } 00686 00687 00688 /* 00689 * Boolean 00690 */ 00691 00692 msgpack_pack_inline_func(_true)(msgpack_pack_user x) 00693 { 00694 static const unsigned char d = 0xc3; 00695 msgpack_pack_append_buffer(x, &d, 1); 00696 } 00697 00698 msgpack_pack_inline_func(_false)(msgpack_pack_user x) 00699 { 00700 static const unsigned char d = 0xc2; 00701 msgpack_pack_append_buffer(x, &d, 1); 00702 } 00703 00704 00705 /* 00706 * Array 00707 */ 00708 00709 msgpack_pack_inline_func(_array)(msgpack_pack_user x, size_t n) 00710 { 00711 if(n < 16) { 00712 unsigned char d = 0x90 | (uint8_t)n; 00713 msgpack_pack_append_buffer(x, &d, 1); 00714 } else if(n < 65536) { 00715 unsigned char buf[3]; 00716 buf[0] = 0xdc; _msgpack_store16(&buf[1], (uint16_t)n); 00717 msgpack_pack_append_buffer(x, buf, 3); 00718 } else { 00719 unsigned char buf[5]; 00720 buf[0] = 0xdd; _msgpack_store32(&buf[1], (uint32_t)n); 00721 msgpack_pack_append_buffer(x, buf, 5); 00722 } 00723 } 00724 00725 00726 /* 00727 * Map 00728 */ 00729 00730 msgpack_pack_inline_func(_map)(msgpack_pack_user x, size_t n) 00731 { 00732 if(n < 16) { 00733 unsigned char d = 0x80 | (uint8_t)n; 00734 msgpack_pack_append_buffer(x, &TAKE8_8(d), 1); 00735 } else if(n < 65536) { 00736 unsigned char buf[3]; 00737 buf[0] = 0xde; _msgpack_store16(&buf[1], (uint16_t)n); 00738 msgpack_pack_append_buffer(x, buf, 3); 00739 } else { 00740 unsigned char buf[5]; 00741 buf[0] = 0xdf; _msgpack_store32(&buf[1], (uint32_t)n); 00742 msgpack_pack_append_buffer(x, buf, 5); 00743 } 00744 } 00745 00746 00747 /* 00748 * Str 00749 */ 00750 00751 msgpack_pack_inline_func(_str)(msgpack_pack_user x, size_t l) 00752 { 00753 if(l < 32) { 00754 unsigned char d = 0xa0 | (uint8_t)l; 00755 msgpack_pack_append_buffer(x, &TAKE8_8(d), 1); 00756 } else if(l < 256) { 00757 unsigned char buf[2]; 00758 buf[0] = 0xd9; buf[1] = (uint8_t)l; 00759 msgpack_pack_append_buffer(x, buf, 2); 00760 } else if(l < 65536) { 00761 unsigned char buf[3]; 00762 buf[0] = 0xda; _msgpack_store16(&buf[1], (uint16_t)l); 00763 msgpack_pack_append_buffer(x, buf, 3); 00764 } else { 00765 unsigned char buf[5]; 00766 buf[0] = 0xdb; _msgpack_store32(&buf[1], (uint32_t)l); 00767 msgpack_pack_append_buffer(x, buf, 5); 00768 } 00769 } 00770 00771 msgpack_pack_inline_func(_str_body)(msgpack_pack_user x, const void* b, size_t l) 00772 { 00773 msgpack_pack_append_buffer(x, (const unsigned char*)b, l); 00774 } 00775 00776 /* 00777 * Raw (V4) 00778 */ 00779 00780 msgpack_pack_inline_func(_v4raw)(msgpack_pack_user x, size_t l) 00781 { 00782 if(l < 32) { 00783 unsigned char d = 0xa0 | (uint8_t)l; 00784 msgpack_pack_append_buffer(x, &TAKE8_8(d), 1); 00785 } else if(l < 65536) { 00786 unsigned char buf[3]; 00787 buf[0] = 0xda; _msgpack_store16(&buf[1], (uint16_t)l); 00788 msgpack_pack_append_buffer(x, buf, 3); 00789 } else { 00790 unsigned char buf[5]; 00791 buf[0] = 0xdb; _msgpack_store32(&buf[1], (uint32_t)l); 00792 msgpack_pack_append_buffer(x, buf, 5); 00793 } 00794 } 00795 00796 msgpack_pack_inline_func(_v4raw_body)(msgpack_pack_user x, const void* b, size_t l) 00797 { 00798 msgpack_pack_append_buffer(x, (const unsigned char*)b, l); 00799 } 00800 00801 /* 00802 * Bin 00803 */ 00804 00805 msgpack_pack_inline_func(_bin)(msgpack_pack_user x, size_t l) 00806 { 00807 if(l < 256) { 00808 unsigned char buf[2]; 00809 buf[0] = 0xc4; buf[1] = (uint8_t)l; 00810 msgpack_pack_append_buffer(x, buf, 2); 00811 } else if(l < 65536) { 00812 unsigned char buf[3]; 00813 buf[0] = 0xc5; _msgpack_store16(&buf[1], (uint16_t)l); 00814 msgpack_pack_append_buffer(x, buf, 3); 00815 } else { 00816 unsigned char buf[5]; 00817 buf[0] = 0xc6; _msgpack_store32(&buf[1], (uint32_t)l); 00818 msgpack_pack_append_buffer(x, buf, 5); 00819 } 00820 } 00821 00822 msgpack_pack_inline_func(_bin_body)(msgpack_pack_user x, const void* b, size_t l) 00823 { 00824 msgpack_pack_append_buffer(x, (const unsigned char*)b, l); 00825 } 00826 00827 /* 00828 * Ext 00829 */ 00830 00831 msgpack_pack_inline_func(_ext)(msgpack_pack_user x, size_t l, int8_t type) 00832 { 00833 switch(l) { 00834 case 1: { 00835 unsigned char buf[2]; 00836 buf[0] = 0xd4; 00837 buf[1] = type; 00838 msgpack_pack_append_buffer(x, buf, 2); 00839 } break; 00840 case 2: { 00841 unsigned char buf[2]; 00842 buf[0] = 0xd5; 00843 buf[1] = type; 00844 msgpack_pack_append_buffer(x, buf, 2); 00845 } break; 00846 case 4: { 00847 unsigned char buf[2]; 00848 buf[0] = 0xd6; 00849 buf[1] = type; 00850 msgpack_pack_append_buffer(x, buf, 2); 00851 } break; 00852 case 8: { 00853 unsigned char buf[2]; 00854 buf[0] = 0xd7; 00855 buf[1] = type; 00856 msgpack_pack_append_buffer(x, buf, 2); 00857 } break; 00858 case 16: { 00859 unsigned char buf[2]; 00860 buf[0] = 0xd8; 00861 buf[1] = type; 00862 msgpack_pack_append_buffer(x, buf, 2); 00863 } break; 00864 default: 00865 if(l < 256) { 00866 unsigned char buf[3]; 00867 buf[0] = 0xc7; 00868 buf[1] = (unsigned char)l; 00869 buf[2] = type; 00870 msgpack_pack_append_buffer(x, buf, 3); 00871 } else if(l < 65536) { 00872 unsigned char buf[4]; 00873 buf[0] = 0xc8; 00874 _msgpack_store16(&buf[1], l); 00875 buf[3] = type; 00876 msgpack_pack_append_buffer(x, buf, 4); 00877 } else { 00878 unsigned char buf[6]; 00879 buf[0] = 0xc9; 00880 _msgpack_store32(&buf[1], l); 00881 buf[5] = type; 00882 msgpack_pack_append_buffer(x, buf, 6); 00883 } 00884 break; 00885 } 00886 } 00887 00888 msgpack_pack_inline_func(_ext_body)(msgpack_pack_user x, const void* b, size_t l) 00889 { 00890 msgpack_pack_append_buffer(x, (const unsigned char*)b, l); 00891 } 00892 00893 #undef msgpack_pack_inline_func 00894 #undef msgpack_pack_user 00895 #undef msgpack_pack_append_buffer 00896 00897 #undef TAKE8_8 00898 #undef TAKE8_16 00899 #undef TAKE8_32 00900 #undef TAKE8_64 00901 00902 #undef msgpack_pack_real_uint8 00903 #undef msgpack_pack_real_uint16 00904 #undef msgpack_pack_real_uint32 00905 #undef msgpack_pack_real_uint64 00906 #undef msgpack_pack_real_int8 00907 #undef msgpack_pack_real_int16 00908 #undef msgpack_pack_real_int32 00909 #undef msgpack_pack_real_int64 00910
Generated on Tue Jul 12 2022 22:51:46 by
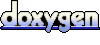