messagepack implementation for embedded systems (mbed / arduino)
Dependents: hello_message_pack
object_fwd.hpp
00001 // 00002 // MessagePack for C++ static resolution routine 00003 // 00004 // Copyright (C) 2008-2014 FURUHASHI Sadayuki and KONDO Takatoshi 00005 // 00006 // Distributed under the Boost Software License, Version 1.0. 00007 // (See accompanying file LICENSE_1_0.txt or copy at 00008 // http://www.boost.org/LICENSE_1_0.txt) 00009 // 00010 00011 #ifndef MSGPACK_OBJECT_FWD_HPP 00012 #define MSGPACK_OBJECT_FWD_HPP 00013 00014 #include "msgpack/versioning.hpp" 00015 #include "msgpack/zone.hpp" 00016 #include "../object.h" 00017 00018 #include <typeinfo> 00019 00020 namespace msgpack { 00021 00022 /// @cond 00023 MSGPACK_API_VERSION_NAMESPACE(v1) { 00024 /// @endcond 00025 00026 00027 namespace type { 00028 enum object_type { 00029 NIL = MSGPACK_OBJECT_NIL, 00030 BOOLEAN = MSGPACK_OBJECT_BOOLEAN, 00031 POSITIVE_INTEGER = MSGPACK_OBJECT_POSITIVE_INTEGER, 00032 NEGATIVE_INTEGER = MSGPACK_OBJECT_NEGATIVE_INTEGER, 00033 FLOAT = MSGPACK_OBJECT_FLOAT, 00034 #if defined(MSGPACK_USE_LEGACY_NAME_AS_FLOAT) 00035 DOUBLE = MSGPACK_OBJECT_DOUBLE, // obsolete 00036 #endif // MSGPACK_USE_LEGACY_NAME_AS_FLOAT 00037 STR = MSGPACK_OBJECT_STR, 00038 BIN = MSGPACK_OBJECT_BIN, 00039 ARRAY = MSGPACK_OBJECT_ARRAY, 00040 MAP = MSGPACK_OBJECT_MAP, 00041 EXT = MSGPACK_OBJECT_EXT 00042 }; 00043 } 00044 00045 00046 struct object; 00047 struct object_kv; 00048 00049 struct object_array { 00050 uint32_t size; 00051 msgpack::object* ptr; 00052 }; 00053 00054 struct object_map { 00055 uint32_t size; 00056 msgpack::object_kv* ptr; 00057 }; 00058 00059 struct object_str { 00060 uint32_t size; 00061 const char* ptr; 00062 }; 00063 00064 struct object_bin { 00065 uint32_t size; 00066 const char* ptr; 00067 }; 00068 00069 struct object_ext { 00070 int8_t type() const { return ptr[0]; } 00071 const char* data() const { return &ptr[1]; } 00072 uint32_t size; 00073 const char* ptr; 00074 }; 00075 00076 00077 #if !defined(MSGPACK_USE_CPP03) 00078 struct object; 00079 00080 namespace adaptor { 00081 template <typename T, typename Enabler = void> 00082 struct as; 00083 } // namespace adaptor 00084 00085 template <typename T> 00086 struct has_as { 00087 private: 00088 template <typename U> 00089 static auto check(U*) -> 00090 typename std::is_same< 00091 decltype(msgpack::adaptor::as<U>()(std::declval<msgpack::object>())), 00092 T>::type; 00093 template <typename> 00094 static std::false_type check(...); 00095 public: 00096 using type = decltype(check<T>(nullptr)); 00097 static constexpr bool value = type::value; 00098 }; 00099 00100 #endif // !defined(MSGPACK_USE_CPP03) 00101 00102 /// Object class that corresponding to MessagePack format object 00103 /** 00104 * See https://github.com/msgpack/msgpack-c/wiki/v1_1_cpp_object 00105 */ 00106 struct object { 00107 union union_type { 00108 bool boolean; 00109 uint64_t u64; 00110 int64_t i64; 00111 #if defined(MSGPACK_USE_LEGACY_NAME_AS_FLOAT) 00112 double dec; // obsolete 00113 #endif // MSGPACK_USE_LEGACY_NAME_AS_FLOAT 00114 double f64; 00115 msgpack::object_array array; 00116 msgpack::object_map map; 00117 msgpack::object_str str; 00118 msgpack::object_bin bin; 00119 msgpack::object_ext ext; 00120 }; 00121 00122 msgpack::type::object_type type; 00123 union_type via; 00124 00125 /// Cheking nil 00126 /** 00127 * @return If the object is nil, then return true, else return false. 00128 */ 00129 bool is_nil() const { return type == msgpack::type::NIL; } 00130 00131 #if defined(MSGPACK_USE_CPP03) 00132 00133 /// Get value as T 00134 /** 00135 * If the object can't be converted to T, msgpack::type_error would be thrown. 00136 * @tparam T The type you want to get. 00137 * @return The converted object. 00138 */ 00139 template <typename T> 00140 T as() const; 00141 00142 #else // defined(MSGPACK_USE_CPP03) 00143 00144 /// Get value as T 00145 /** 00146 * If the object can't be converted to T, msgpack::type_error would be thrown. 00147 * @tparam T The type you want to get. 00148 * @return The converted object. 00149 */ 00150 template <typename T> 00151 typename std::enable_if<msgpack::has_as<T>::value, T>::type as() const; 00152 00153 /// Get value as T 00154 /** 00155 * If the object can't be converted to T, msgpack::type_error would be thrown. 00156 * @tparam T The type you want to get. 00157 * @return The converted object. 00158 */ 00159 template <typename T> 00160 typename std::enable_if<!msgpack::has_as<T>::value, T>::type as() const; 00161 00162 #endif // defined(MSGPACK_USE_CPP03) 00163 00164 /// Convert the object 00165 /** 00166 * If the object can't be converted to T, msgpack::type_error would be thrown. 00167 * @tparam T The type of v. 00168 * @param v The value you want to get. `v` is output parameter. `v` is overwritten by converted value from the object. 00169 * @return The reference of `v`. 00170 */ 00171 template <typename T> 00172 T& convert(T& v) const; 00173 00174 00175 #if !defined(MSGPACK_DISABLE_LEGACY_CONVERT) 00176 /// Convert the object (obsolete) 00177 /** 00178 * If the object can't be converted to T, msgpack::type_error would be thrown. 00179 * @tparam T The type of v. 00180 * @param v The pointer of the value you want to get. `v` is output parameter. `*v` is overwritten by converted value from the object. 00181 * @return The pointer of `v`. 00182 */ 00183 template <typename T> 00184 T* convert(T* v) const; 00185 #endif // !defined(MSGPACK_DISABLE_LEGACY_CONVERT) 00186 00187 /// Convert the object if not nil 00188 /** 00189 * If the object is not nil and can't be converted to T, msgpack::type_error would be thrown. 00190 * @tparam T The type of v. 00191 * @param v The value you want to get. `v` is output parameter. `v` is overwritten by converted value from the object if the object is not nil. 00192 * @return If the object is nil, then return false, else return true. 00193 */ 00194 template <typename T> 00195 bool convert_if_not_nil(T& v) const; 00196 00197 /// Default constructor. The object is set to nil. 00198 object(); 00199 00200 /// Copy constructor. Object is shallow copied. 00201 object(const msgpack_object& o); 00202 00203 /// Construct object from T 00204 /** 00205 * If `v` is the type that is corresponding to MessegePack format str, bin, ext, array, or map, 00206 * you need to call `object(const T& v, msgpack::zone& z)` instead of this constructor. 00207 * 00208 * @tparam T The type of `v`. 00209 * @param v The value you want to convert. 00210 */ 00211 template <typename T> 00212 explicit object(const T& v); 00213 00214 /// Construct object from T 00215 /** 00216 * The object is constructed on the zone `z`. 00217 * See https://github.com/msgpack/msgpack-c/wiki/v1_1_cpp_object 00218 * 00219 * @tparam T The type of `v`. 00220 * @param v The value you want to convert. 00221 * @param z The zone that is used by the object. 00222 */ 00223 template <typename T> 00224 object(const T& v, msgpack::zone& z); 00225 00226 /// Construct object from T (obsolete) 00227 /** 00228 * The object is constructed on the zone `z`. 00229 * Use `object(const T& v, msgpack::zone& z)` instead of this constructor. 00230 * See https://github.com/msgpack/msgpack-c/wiki/v1_1_cpp_object 00231 * 00232 * @tparam T The type of `v`. 00233 * @param v The value you want to convert. 00234 * @param z The pointer to the zone that is used by the object. 00235 */ 00236 template <typename T> 00237 object(const T& v, msgpack::zone* z); 00238 00239 template <typename T> 00240 object& operator=(const T& v); 00241 00242 operator msgpack_object() const; 00243 00244 struct with_zone; 00245 00246 private: 00247 struct implicit_type; 00248 00249 public: 00250 implicit_type convert() const; 00251 }; 00252 00253 class type_error : public std::bad_cast { }; 00254 00255 struct object_kv { 00256 msgpack::object key; 00257 msgpack::object val; 00258 }; 00259 00260 struct object::with_zone : object { 00261 with_zone(msgpack::zone& z) : zone(z) { } 00262 msgpack::zone& zone; 00263 private: 00264 with_zone(); 00265 }; 00266 00267 /// @cond 00268 } // MSGPACK_API_VERSION_NAMESPACE(v1) 00269 /// @endcond 00270 00271 } // namespace msgpack 00272 00273 #endif // MSGPACK_OBJECT_FWD_HPP
Generated on Tue Jul 12 2022 22:51:46 by
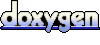