messagepack implementation for embedded systems (mbed / arduino)
Dependents: hello_message_pack
object.h
00001 /* 00002 * MessagePack for C dynamic typing routine 00003 * 00004 * Copyright (C) 2008-2009 FURUHASHI Sadayuki 00005 * 00006 * Distributed under the Boost Software License, Version 1.0. 00007 * (See accompanying file LICENSE_1_0.txt or copy at 00008 * http://www.boost.org/LICENSE_1_0.txt) 00009 */ 00010 #ifndef MSGPACK_OBJECT_H 00011 #define MSGPACK_OBJECT_H 00012 00013 #include "zone.h" 00014 #include <stdio.h> 00015 00016 #ifdef __cplusplus 00017 extern "C" { 00018 #endif 00019 00020 00021 /** 00022 * @defgroup msgpack_object Dynamically typed object 00023 * @ingroup msgpack 00024 * @{ 00025 */ 00026 00027 typedef enum { 00028 MSGPACK_OBJECT_NIL = 0x00, 00029 MSGPACK_OBJECT_BOOLEAN = 0x01, 00030 MSGPACK_OBJECT_POSITIVE_INTEGER = 0x02, 00031 MSGPACK_OBJECT_NEGATIVE_INTEGER = 0x03, 00032 MSGPACK_OBJECT_FLOAT = 0x04, 00033 #if defined(MSGPACK_USE_LEGACY_NAME_AS_FLOAT) 00034 MSGPACK_OBJECT_DOUBLE = MSGPACK_OBJECT_FLOAT, /* obsolete */ 00035 #endif /* MSGPACK_USE_LEGACY_NAME_AS_FLOAT */ 00036 MSGPACK_OBJECT_STR = 0x05, 00037 MSGPACK_OBJECT_ARRAY = 0x06, 00038 MSGPACK_OBJECT_MAP = 0x07, 00039 MSGPACK_OBJECT_BIN = 0x08, 00040 MSGPACK_OBJECT_EXT = 0x09 00041 } msgpack_object_type; 00042 00043 00044 struct msgpack_object; 00045 struct msgpack_object_kv; 00046 00047 typedef struct { 00048 uint32_t size; 00049 struct msgpack_object* ptr; 00050 } msgpack_object_array; 00051 00052 typedef struct { 00053 uint32_t size; 00054 struct msgpack_object_kv* ptr; 00055 } msgpack_object_map; 00056 00057 typedef struct { 00058 uint32_t size; 00059 const char* ptr; 00060 } msgpack_object_str; 00061 00062 typedef struct { 00063 uint32_t size; 00064 const char* ptr; 00065 } msgpack_object_bin; 00066 00067 typedef struct { 00068 int8_t type; 00069 uint32_t size; 00070 const char* ptr; 00071 } msgpack_object_ext; 00072 00073 typedef union { 00074 bool boolean; 00075 uint64_t u64; 00076 int64_t i64; 00077 #if defined(MSGPACK_USE_LEGACY_NAME_AS_FLOAT) 00078 double dec; /* obsolete*/ 00079 #endif /* MSGPACK_USE_LEGACY_NAME_AS_FLOAT */ 00080 double f64; 00081 msgpack_object_array array; 00082 msgpack_object_map map; 00083 msgpack_object_str str; 00084 msgpack_object_bin bin; 00085 msgpack_object_ext ext; 00086 } msgpack_object_union; 00087 00088 typedef struct msgpack_object { 00089 msgpack_object_type type; 00090 msgpack_object_union via; 00091 } msgpack_object; 00092 00093 typedef struct msgpack_object_kv { 00094 msgpack_object key; 00095 msgpack_object val; 00096 } msgpack_object_kv; 00097 00098 MSGPACK_DLLEXPORT 00099 void msgpack_object_print(FILE* out, msgpack_object o); 00100 00101 MSGPACK_DLLEXPORT 00102 bool msgpack_object_equal(const msgpack_object x, const msgpack_object y); 00103 00104 /** @} */ 00105 00106 00107 #ifdef __cplusplus 00108 } 00109 #endif 00110 00111 #endif /* msgpack/object.h */
Generated on Tue Jul 12 2022 22:51:46 by
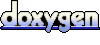