messagepack implementation for embedded systems (mbed / arduino)
Dependents: hello_message_pack
nil.hpp
00001 // 00002 // MessagePack for C++ static resolution routine 00003 // 00004 // Copyright (C) 2008-2009 FURUHASHI Sadayuki 00005 // 00006 // Distributed under the Boost Software License, Version 1.0. 00007 // (See accompanying file LICENSE_1_0.txt or copy at 00008 // http://www.boost.org/LICENSE_1_0.txt) 00009 // 00010 #ifndef MSGPACK_TYPE_NIL_HPP 00011 #define MSGPACK_TYPE_NIL_HPP 00012 00013 #include "msgpack/versioning.hpp" 00014 #include "msgpack/adaptor/adaptor_base.hpp" 00015 00016 namespace msgpack { 00017 00018 /// @cond 00019 MSGPACK_API_VERSION_NAMESPACE(v1) { 00020 /// @endcond 00021 00022 namespace type { 00023 00024 struct nil_t { }; 00025 00026 #if !defined(MSGPACK_DISABLE_LEGACY_NIL) 00027 00028 typedef nil_t nil; 00029 00030 #endif // !defined(MSGPACK_DISABLE_LEGACY_NIL) 00031 00032 inline bool operator<(nil_t const& lhs, nil_t const& rhs) { 00033 return &lhs < &rhs; 00034 } 00035 00036 inline bool operator==(nil_t const& lhs, nil_t const& rhs) { 00037 return &lhs == &rhs; 00038 } 00039 00040 } // namespace type 00041 00042 namespace adaptor { 00043 00044 template <> 00045 struct convert<type::nil_t> { 00046 msgpack::object const& operator()(msgpack::object const& o, type::nil_t&) const { 00047 if(o.type != msgpack::type::NIL) { 00048 // throw msgpack::type_error(); 00049 } 00050 return o; 00051 } 00052 }; 00053 00054 template <> 00055 struct pack<type::nil_t> { 00056 template <typename Stream> 00057 msgpack::packer<Stream> & operator()(msgpack::packer<Stream> & o, const type::nil_t&) const { 00058 o.pack_nil(); 00059 return o; 00060 } 00061 }; 00062 00063 template <> 00064 struct object<type::nil_t> { 00065 void operator()(msgpack::object& o, type::nil_t) const { 00066 o.type = msgpack::type::NIL; 00067 } 00068 }; 00069 00070 template <> 00071 struct object_with_zone<type::nil_t> { 00072 void operator()(msgpack::object::with_zone& o, type::nil_t v) const { 00073 static_cast<msgpack::object&>(o) << v; 00074 } 00075 }; 00076 00077 } // namespace adaptor 00078 00079 template <> 00080 inline void msgpack::object::as<void>() const 00081 { 00082 msgpack::type::nil_t v; 00083 convert(v); 00084 } 00085 00086 /// @cond 00087 } // MSGPACK_API_VERSION_NAMESPACE(v1) 00088 /// @endcond 00089 00090 } // namespace msgpack 00091 00092 #endif // MSGPACK_TYPE_NIL_HPP
Generated on Tue Jul 12 2022 22:51:46 by
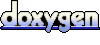