messagepack implementation for embedded systems (mbed / arduino)
Dependents: hello_message_pack
check_container_size.hpp
00001 // 00002 // MessagePack for C++ static resolution routine 00003 // 00004 // Copyright (C) 2015 KONDO Takatoshi 00005 // 00006 // Distributed under the Boost Software License, Version 1.0. 00007 // (See accompanying file LICENSE_1_0.txt or copy at 00008 // http://www.boost.org/LICENSE_1_0.txt) 00009 // 00010 #ifndef MSGPACK_CHECK_CONTAINER_SIZE_HPP 00011 #define MSGPACK_CHECK_CONTAINER_SIZE_HPP 00012 00013 #include "msgpack/versioning.hpp" 00014 #include <stdexcept> 00015 00016 namespace msgpack { 00017 00018 /// @cond 00019 MSGPACK_API_VERSION_NAMESPACE(v1) { 00020 /// @endcond 00021 00022 struct container_size_overflow : public std::runtime_error { 00023 explicit container_size_overflow(const std::string& msg) 00024 :std::runtime_error(msg) {} 00025 #if !defined(MSGPACK_USE_CPP03) 00026 explicit container_size_overflow(const char* msg): 00027 std::runtime_error(msg) {} 00028 #endif // !defined(MSGPACK_USE_CPP03) 00029 }; 00030 00031 namespace detail { 00032 00033 template <std::size_t N> 00034 inline void check_container_size(std::size_t size) { 00035 if (size > 0xffffffff) throw container_size_overflow("container size overflow"); 00036 } 00037 00038 template <> 00039 inline void check_container_size<4>(std::size_t /*size*/) { 00040 } 00041 00042 template <std::size_t N> 00043 inline void check_container_size_for_ext(std::size_t size) { 00044 if (size > 0xffffffff) throw container_size_overflow("container size overflow"); 00045 } 00046 00047 template <> 00048 inline void check_container_size_for_ext<4>(std::size_t size) { 00049 // if (size > 0xfffffffe) throw container_size_overflow("container size overflow"); 00050 } 00051 00052 } // namespace detail 00053 00054 template <typename T> 00055 inline uint32_t checked_get_container_size(T size) { 00056 detail::check_container_size<sizeof(T)>(size); 00057 return static_cast<uint32_t>(size); 00058 } 00059 00060 00061 /// @cond 00062 } // MSGPACK_API_VERSION_NAMESPACE(v1) 00063 /// @endcond 00064 00065 } // namespace msgpack 00066 00067 #endif // MSGPACK_CHECK_CONTAINER_SIZE_HPP
Generated on Tue Jul 12 2022 22:51:44 by
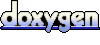