messagepack implementation for embedded systems (mbed / arduino)
Dependents: hello_message_pack
adaptor_base.hpp
00001 // 00002 // MessagePack for C++ static resolution routine 00003 // 00004 // Copyright (C) 2015 KONDO Takatoshi 00005 // 00006 // Distributed under the Boost Software License, Version 1.0. 00007 // (See accompanying file LICENSE_1_0.txt or copy at 00008 // http://www.boost.org/LICENSE_1_0.txt) 00009 // 00010 #ifndef MSGPACK_ADAPTOR_BASE_HPP 00011 #define MSGPACK_ADAPTOR_BASE_HPP 00012 00013 #include "msgpack/object_fwd.hpp" 00014 00015 namespace msgpack { 00016 00017 /// @cond 00018 MSGPACK_API_VERSION_NAMESPACE(v1) { 00019 /// @endcond 00020 00021 template <typename Stream> 00022 class packer; 00023 00024 namespace adaptor { 00025 00026 // Adaptor functors 00027 00028 template <typename T, typename Enabler = void> 00029 struct convert { 00030 msgpack::object const& operator()(msgpack::object const& o, T& v) const; 00031 }; 00032 00033 template <typename T, typename Enabler = void> 00034 struct pack { 00035 template <typename Stream> 00036 msgpack::packer<Stream> & operator()(msgpack::packer<Stream> & o, T const& v) const; 00037 }; 00038 00039 template <typename T, typename Enabler = void> 00040 struct object { 00041 void operator()(msgpack::object& o, T const& v) const; 00042 }; 00043 00044 template <typename T, typename Enabler = void> 00045 struct object_with_zone { 00046 void operator()(msgpack::object::with_zone& o, T const& v) const; 00047 }; 00048 00049 } // namespace adaptor 00050 00051 // operators 00052 00053 template <typename T> 00054 inline 00055 msgpack::object const& operator>> (msgpack::object const& o, T& v) { 00056 return adaptor::convert<T>()(o, v); 00057 } 00058 00059 template <typename Stream, typename T> 00060 inline 00061 msgpack::packer<Stream> & operator<< (msgpack::packer<Stream>& o, T const& v) { 00062 return adaptor::pack<T>()(o, v); 00063 } 00064 00065 template <typename T> 00066 inline 00067 void operator<< (msgpack::object& o, T const& v) { 00068 adaptor::object<T>()(o, v); 00069 } 00070 00071 template <typename T> 00072 inline 00073 void operator<< (msgpack::object::with_zone& o, T const& v) { 00074 adaptor::object_with_zone<T>()(o, v); 00075 } 00076 00077 /// @cond 00078 } // MSGPACK_API_VERSION_NAMESPACE(v1) 00079 /// @endcond 00080 00081 } // namespace msgpack 00082 00083 00084 #endif // MSGPACK_ADAPTOR_BASE_HPP
Generated on Tue Jul 12 2022 22:51:44 by
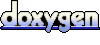