
This is a for debugging \\\\\\\"BLUE USB\\\\\\\". You can connect with HCI mode. How to connect White Wizard Board TANK *White Wizard Board - Motor Driver Board * p 21 - IN_R1 * p 22 - IN_R2 * p 23 - IN_L2 * p 24 - IN_L1
TestShell.cpp
00001 00002 /* 00003 Copyright (c) 2010 Peter Barrett 00004 00005 Permission is hereby granted, free of charge, to any person obtaining a copy 00006 of this software and associated documentation files (the "Software"), to deal 00007 in the Software without restriction, including without limitation the rights 00008 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00009 copies of the Software, and to permit persons to whom the Software is 00010 furnished to do so, subject to the following conditions: 00011 00012 The above copyright notice and this permission notice shall be included in 00013 all copies or substantial portions of the Software. 00014 00015 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00016 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00017 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00018 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00019 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00020 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00021 THE SOFTWARE. 00022 */ 00023 00024 #include "mbed.h" 00025 00026 #include <stdio.h> 00027 #include <stdlib.h> 00028 #include <stdio.h> 00029 #include <string.h> 00030 00031 #include "Utils.h" 00032 #include "USBHost.h" 00033 #include "hci.h" 00034 //#include "hci_private.h" 00035 00036 DigitalOut myled1(LED1); 00037 DigitalOut myled2(LED2); 00038 DigitalOut myled3(LED3); 00039 DigitalOut myled4(LED4); 00040 00041 DigitalOut pin11(p21); 00042 DigitalOut pin12(p22); 00043 DigitalOut pin13(p23); 00044 DigitalOut pin14(p24); 00045 00046 void printf(const BD_ADDR* addr) 00047 { 00048 const u8* a = addr->addr; 00049 printf("%02X:%02X:%02X:%02X:%02X:%02X",a[5],a[4],a[3],a[2],a[1],a[0]); 00050 } 00051 00052 #define MAX_HCL_SIZE 260 00053 #define MAX_ACL_SIZE 400 00054 00055 class HCITransportUSB : public HCITransport 00056 { 00057 int _device; 00058 u8* _hciBuffer; 00059 u8* _aclBuffer; 00060 00061 public: 00062 void Open(int device, u8* hciBuffer, u8* aclBuffer) 00063 { 00064 _device = device; 00065 _hciBuffer = hciBuffer; 00066 _aclBuffer = aclBuffer; 00067 USBInterruptTransfer(_device,0x81,_hciBuffer,MAX_HCL_SIZE,HciCallback,this); 00068 USBBulkTransfer(_device,0x82,_aclBuffer,MAX_ACL_SIZE,AclCallback,this); 00069 } 00070 00071 static void HciCallback(int device, int endpoint, int status, u8* data, int len, void* userData) 00072 { 00073 HCI* t = ((HCITransportUSB*)userData)->_target; 00074 if (t) 00075 t->HCIRecv(data,len); 00076 USBInterruptTransfer(device,0x81,data,MAX_HCL_SIZE,HciCallback,userData); 00077 } 00078 00079 static void AclCallback(int device, int endpoint, int status, u8* data, int len, void* userData) 00080 { 00081 00082 //-----y.k----- 00083 printf("ACLRecv "); 00084 int i = 0; 00085 //u8 = unsigned char 00086 printf(" : data[] = "); 00087 00088 for(i=0;i<sizeof(data);++i){ 00089 printf(" 0x%x : " ,data[i]); 00090 } 00091 00092 //-----y.k----- 00093 00094 printf("sizeof(data) = %d \n", sizeof(data)); 00095 00096 00097 HCI* t = ((HCITransportUSB*)userData)->_target; 00098 if (t) 00099 t->ACLRecv(data,len); 00100 USBBulkTransfer(device,0x82,data,MAX_ACL_SIZE,AclCallback,userData); 00101 } 00102 00103 virtual void HCISend(const u8* data, int len) 00104 { 00105 //-----y.k----- 00106 int i = 0; 00107 00108 //u8 = unsigned char 00109 printf("-----y.k-----\n"); 00110 printf("******HCISend() :\n data[] = "); 00111 00112 for(i=0;i<len;++i){ 00113 printf(" 0x%x : " ,data[i]); 00114 } 00115 00116 printf("len = %d \n", len); 00117 printf("-----y.k-----\n"); 00118 00119 USBControlTransfer(_device,REQUEST_TYPE_CLASS, 0, 0, 0,(u8*)data,len); 00120 } 00121 //-----y.k----- 00122 00123 virtual void ACLSend(const u8* data, int len) 00124 { 00125 USBBulkTransfer(_device,0x02,(u8*)data,len); 00126 } 00127 }; 00128 00129 00130 #define WII_REMOTE 0x042500 00131 00132 class HIDBluetooth 00133 { 00134 int _control; // Sockets for control (out) and interrupt (in) 00135 int _interrupt; 00136 int _devClass; 00137 BD_ADDR _addr; 00138 u8 _pad[2]; // Struct align 00139 00140 public: 00141 HIDBluetooth() : _control(0),_interrupt(0),_devClass(0) {}; 00142 00143 bool InUse() 00144 { 00145 return _control != 0; 00146 } 00147 00148 static void OnHidInterrupt(int socket, SocketState state, const u8* data, int len, void* userData) 00149 { 00150 HIDBluetooth* t = (HIDBluetooth*)userData; 00151 00152 if (data) 00153 { 00154 if (t->_devClass == WII_REMOTE && data[1] == 0x30) 00155 { 00156 printf("================wii====================\n"); 00157 t->Led(); 00158 t->Hid(); // ask for accelerometer 00159 t->_devClass = 0; 00160 } 00161 00162 const u8* d = data; 00163 00164 //-----y.k----- 00165 int i = 0; 00166 //u8 = unsigned char 00167 printf("-----y.k-----\n"); 00168 printf("/////// OnHidInterrupt() :\n d[] = "); 00169 00170 for(i=0;i<sizeof(data)+1;++i){ 00171 printf(" 0x%x : " ,data[i]); 00172 } 00173 00174 printf("len = %d \n", len); 00175 printf("-----y.k-----\n"); 00176 //-----y.k----- 00177 00178 switch (d[1]) 00179 { 00180 case 0x02: 00181 { 00182 int x = (signed char)d[3]; 00183 int y = (signed char)d[4]; 00184 printf("Mouse %2X dx:%d dy:%d\n",d[2],x,y); 00185 } 00186 break; 00187 00188 case 0x37: // Accelerometer http://wiki.wiimoteproject.com/Reports 00189 { 00190 int pad = (d[2] & 0x9F) | ((d[3] & 0x9F) << 8); 00191 int x = (d[2] & 0x60) >> 5 | d[4] << 2; 00192 int y = (d[3] & 0x20) >> 4 | d[5] << 2; 00193 int z = (d[3] & 0x40) >> 5 | d[6] << 2; 00194 printf("WII %04X %d %d %d\n",pad,x,y,z); 00195 } 00196 break; 00197 00198 00199 ///--------------------------------- 00200 00201 case 0x01: // Mini Keyboard 00202 { 00203 int keycode = (d[4]); 00204 printf("Keycode = %02x \n",keycode); 00205 switch(keycode) 00206 { 00207 case 0x00: // key off 00208 { 00209 printf("Key OFF"); 00210 pin11 = 1; 00211 pin12 = 1; 00212 pin13 = 1; 00213 pin14 = 1; 00214 00215 myled1 = 1; 00216 myled2 = 1; 00217 myled3 = 1; 00218 myled4 = 1; 00219 00220 break; 00221 } 00222 case 0x51: // "down" 00223 { 00224 printf("Key down "); 00225 00226 pin11 = 1; 00227 pin12 = 0; 00228 pin13 = 0; 00229 pin14 = 1; 00230 00231 myled1 = 1; 00232 myled2 = 0; 00233 myled3 = 0; 00234 myled4 = 1; 00235 00236 break; 00237 } 00238 case 0x52: // "up" 00239 { 00240 printf("Key up "); 00241 00242 pin11 = 0; 00243 pin12 = 1; 00244 pin13 = 1; 00245 pin14 = 0; 00246 00247 myled1 = 0; 00248 myled2 = 1; 00249 myled3 = 1; 00250 myled4 = 0; 00251 00252 break; 00253 } 00254 00255 case 0x50: // "left" 00256 { 00257 printf("Key left "); 00258 00259 pin11 = 0; 00260 pin12 = 1; 00261 pin13 = 0; 00262 pin14 = 1; 00263 00264 myled1 = 0; 00265 myled2 = 1; 00266 myled3 = 0; 00267 myled4 = 1; 00268 00269 break; 00270 } 00271 00272 case 0x4f: // "right" 00273 { 00274 printf("Key right "); 00275 00276 pin11 = 1; 00277 pin12 = 0; 00278 pin13 = 1; 00279 pin14 = 0; 00280 00281 myled1 = 1; 00282 myled2 = 0; 00283 myled3 = 1; 00284 myled4 = 0; 00285 00286 break; 00287 } 00288 00289 case 0x28: // "Enter" 00290 { 00291 printf("Key Enter "); 00292 00293 pin11 = 0; 00294 pin12 = 0; 00295 pin13 = 0; 00296 pin14 = 0; 00297 00298 myled1 = 0; 00299 myled2 = 0; 00300 myled3 = 0; 00301 myled4 = 0; 00302 break; 00303 } 00304 default: 00305 printf("Key ???"); 00306 break; 00307 00308 } 00309 00310 } 00311 break; 00312 case 0x03: // Connected? 00313 { 00314 //pi.locate(0,1); 00315 //pi.printf("Connect!"); 00316 break; 00317 00318 } 00319 00320 00321 00322 ///--------------------------------- 00323 00324 default: 00325 printHex(data,len); 00326 } 00327 } 00328 } 00329 00330 static void OnHidControl(int socket, SocketState state, const u8* data, int len, void* userData) 00331 { 00332 printf("OnHidControl\n"); 00333 if (data) 00334 printHex(data,len); 00335 } 00336 00337 void Open(BD_ADDR* bdAddr, inquiry_info* info) 00338 { 00339 printf("L2CAPAddr size %d\n",sizeof(L2CAPAddr)); 00340 _addr = *bdAddr; 00341 L2CAPAddr sockAddr; 00342 sockAddr.bdaddr = _addr; 00343 sockAddr.psm = L2CAP_PSM_HID_INTR; 00344 printf("Socket_Open size %d\n",sizeof(L2CAPAddr)); 00345 _interrupt = Socket_Open(SOCKET_L2CAP,&sockAddr.hdr,OnHidInterrupt,this); 00346 sockAddr.psm = L2CAP_PSM_HID_CNTL; 00347 _control = Socket_Open(SOCKET_L2CAP,&sockAddr.hdr,OnHidControl,this); 00348 00349 printfBytes("OPEN DEVICE CLASS",info->dev_class,3); 00350 _devClass = (info->dev_class[0] << 16) | (info->dev_class[1] << 8) | info->dev_class[2]; 00351 } 00352 00353 void Close() 00354 { 00355 if (_control) 00356 Socket_Close(_control); 00357 if (_interrupt) 00358 Socket_Close(_interrupt); 00359 _control = _interrupt = 0; 00360 } 00361 00362 void Led(int id = 0x10) 00363 { 00364 u8 led[3] = {0x52, 0x11, id}; 00365 if (_control) 00366 Socket_Send(_control,led,3); 00367 } 00368 00369 void Hid(int report = 0x37) 00370 { 00371 u8 hid[4] = { 0x52, 0x12, 0x00, report }; 00372 if (_control != -1) 00373 Socket_Send(_control,hid,4); 00374 } 00375 }; 00376 00377 00378 HCI* gHCI = 0; 00379 00380 #define MAX_HID_DEVICES 8 00381 00382 int GetConsoleChar(); 00383 class ShellApp 00384 { 00385 char _line[64]; 00386 HIDBluetooth _hids[MAX_HID_DEVICES]; 00387 00388 public: 00389 void Ready() 00390 { 00391 printf("HIDBluetooth %d\n",sizeof(HIDBluetooth)); 00392 memset(_hids,0,sizeof(_hids)); 00393 Inquiry(); 00394 00395 } 00396 00397 // We have connected to a device 00398 void ConnectionComplete(HCI* hci, connection_info* info) 00399 { 00400 printf("ConnectionComplete "); 00401 BD_ADDR* a = &info->bdaddr; 00402 printf(a); 00403 BTDevice* bt = hci->Find(a); 00404 HIDBluetooth* hid = NewHIDBluetooth(); 00405 printf(" %08x %08x\n",bt,hid); 00406 if (hid) 00407 hid->Open(a,&bt->_info); 00408 } 00409 00410 HIDBluetooth* NewHIDBluetooth() 00411 { 00412 for (int i = 0; i < MAX_HID_DEVICES; i++) 00413 if (!_hids[i].InUse()) 00414 return _hids+i; 00415 return 0; 00416 } 00417 00418 void ConnectDevices() 00419 { 00420 BTDevice* devs[8]; 00421 int count = gHCI->GetDevices(devs,8); 00422 for (int i = 0; i < count; i++) 00423 { 00424 printfBytes("DEVICE CLASS",devs[i]->_info.dev_class,3); 00425 if (devs[i]->_handle == 0) 00426 { 00427 BD_ADDR* bd = &devs[i]->_info.bdaddr; 00428 printf("Connecting to "); 00429 printf(bd); 00430 printf("\n"); 00431 gHCI->CreateConnection(bd); 00432 } 00433 } 00434 } 00435 00436 const char* ReadLine() 00437 { 00438 int i; 00439 for (i = 0; i < 255; ) 00440 { 00441 ///printf("--- ReadLine() -> USBLoop()---\n"); 00442 USBLoop(); 00443 int c = GetConsoleChar(); 00444 if (c == -1) 00445 continue; 00446 if (c == '\n' || c == 13) 00447 break; 00448 _line[i++] = c; 00449 } 00450 _line[i] = 0; 00451 return _line; 00452 } 00453 /* 00454 void Setlocalname(){ 00455 printf("Setlocalname..\n"); 00456 gHCI->Setlocalname(); 00457 } 00458 */ 00459 00460 void Inquiry() 00461 { 00462 printf("Inquiry..\n"); 00463 gHCI->Inquiry(); 00464 } 00465 00466 void List() 00467 { 00468 #if 0 00469 printf("%d devices\n",_deviceCount); 00470 for (int i = 0; i < _deviceCount; i++) 00471 { 00472 printf(&_devices[i].info.bdaddr); 00473 printf("\n"); 00474 } 00475 #endif 00476 } 00477 00478 void Connect() 00479 { 00480 ConnectDevices(); 00481 } 00482 00483 void Disconnect() 00484 { 00485 gHCI->DisconnectAll(); 00486 } 00487 00488 void CloseMouse() 00489 { 00490 } 00491 00492 void Quit() 00493 { 00494 CloseMouse(); 00495 } 00496 00497 void Run() 00498 { 00499 for(;;) 00500 { 00501 printf("\n ---Run() for(;;) --- \n");//-----y.k----- 00502 const char* cmd = ReadLine(); 00503 printf("\n --- END : cmd = ReadLine() --- \n");//-----y.k----- 00504 00505 //printf("\n --- for(;;) cmd:%s --- \n\n",cmd); 00506 00507 if (strcmp(cmd,"scan") == 0 || strcmp(cmd,"inquiry") == 0){ 00508 printf("---Inquiry();---\n");//-----y.k----- 00509 Inquiry(); 00510 }else if (strcmp(cmd,"ls") == 0){ 00511 printf("---List();---\n");//-----y.k----- 00512 List(); 00513 }else if (strcmp(cmd,"connect") == 0){ 00514 printf("---Connect();---\n");//-----y.k----- 00515 Connect(); 00516 }else if (strcmp(cmd,"disconnect") == 0){ 00517 printf("---Disconnect();---\n");//-----y.k----- 00518 Disconnect(); 00519 }else if (strcmp(cmd,"q")== 0) 00520 { 00521 Quit(); 00522 break; 00523 } else { 00524 printf("eh? %s\n",cmd); 00525 } 00526 } 00527 } 00528 }; 00529 00530 // Instance 00531 ShellApp gApp; 00532 00533 static int HciCallback(HCI* hci, HCI_CALLBACK_EVENT evt, const u8* data, int len) 00534 { 00535 00536 printf("HciCallback %04X \n",evt); 00537 00538 switch (evt) 00539 { 00540 case CALLBACK_READY: 00541 //gApp.Setlocalname(); 00542 printf("CALLBACK_READY\n"); 00543 gApp.Ready(); 00544 break; 00545 00546 case CALLBACK_INQUIRY_RESULT: 00547 printf("CALLBACK_INQUIRY_RESULT "); 00548 printf((BD_ADDR*)data); 00549 printf("\n"); 00550 00551 //Bluetooth address : BD_ADDR 00552 00553 00554 break; 00555 00556 case CALLBACK_INQUIRY_DONE: 00557 printf("CALLBACK_INQUIRY_DONE\n"); 00558 gApp.ConnectDevices(); 00559 break; 00560 00561 case CALLBACK_REMOTE_NAME: 00562 { 00563 BD_ADDR* addr = (BD_ADDR*)data; 00564 const char* name = (const char*)(data + 6); 00565 printf(addr); 00566 printf(" % s\n",name); 00567 } 00568 break; 00569 00570 case CALLBACK_CONNECTION_COMPLETE: 00571 gApp.ConnectionComplete(hci,(connection_info*)data); 00572 break; 00573 }; 00574 return 0; 00575 } 00576 00577 // these should be placed in the DMA SRAM 00578 typedef struct 00579 { 00580 u8 _hciBuffer[MAX_HCL_SIZE]; 00581 u8 _aclBuffer[MAX_ACL_SIZE]; 00582 } SRAMPlacement; 00583 00584 HCITransportUSB _HCITransportUSB; 00585 HCI _HCI; 00586 00587 u8* USBGetBuffer(u32* len); 00588 int OnBluetoothInsert(int device) 00589 { 00590 printf("Bluetooth inserted of %d\n",device); 00591 u32 sramLen; 00592 u8* sram = USBGetBuffer(&sramLen); 00593 sram = (u8*)(((u32)sram + 1023) & ~1023); 00594 SRAMPlacement* s = (SRAMPlacement*)sram; 00595 _HCITransportUSB.Open(device,s->_hciBuffer,s->_aclBuffer); 00596 _HCI.Open(&_HCITransportUSB,HciCallback); 00597 RegisterSocketHandler(SOCKET_L2CAP,&_HCI); 00598 gHCI = &_HCI; 00599 gApp.Inquiry(); 00600 return 0; 00601 } 00602 00603 void TestShell() 00604 { 00605 printf("\nTestShell()\n\n"); 00606 USBInit(); 00607 00608 printf("\nUSBInit():OK --- gApp.Run()\n\n"); 00609 gApp.Run(); 00610 }
Generated on Tue Jul 19 2022 02:02:54 by
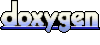