mBed RFM12B module library
Fork of RF12B by
Embed:
(wiki syntax)
Show/hide line numbers
RFM12B.h
00001 /* 00002 RFM12B Library. Based on work done by JeeLabs.org ported to mBed by SK Pang. 00003 http://jeelabs.net/projects/cafe/wiki/RF12 00004 Jan 2012 skpang.co.uk 00005 00006 RFM12B Library (Moteino Comunication Protocol). Based on work done by Felix Rusu ported to mBed by Hugo Rodrigues 00007 http://lowpowerlab.com/blog/2012/12/28/rfm12b-arduino-library/ 00008 May 2013 Hugo Rodrigues 00009 00010 http://opensource.org/licenses/mit-license.php 00011 00012 Permission is hereby granted, free of charge, to any person obtaining a copy 00013 of this software and associated documentation files (the "Software"), to deal 00014 in the Software without restriction, including without limitation the rights 00015 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00016 copies of the Software, and to permit persons to whom the Software is 00017 furnished to do so, subject to the following conditions: 00018 00019 The above copyright notice and this permission notice shall be included in 00020 all copies or substantial portions of the Software. 00021 00022 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00023 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00024 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00025 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00026 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00027 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00028 THE SOFTWARE. 00029 */ 00030 00031 #ifndef _RFM12B_H 00032 #define _RFM12B_H 00033 00034 #include "mbed.h" 00035 00036 /// RF12 Maximum message size in bytes. 00037 #define RF12_MAXDATA 128 00038 /// Max transmit/receive buffer: 4 header + data + 2 crc bytes 00039 #define RF_MAX (RF12_MAXDATA + 6) 00040 00041 #define RF12_433MHZ 1 00042 #define RF12_868MHZ 2 00043 #define RF12_915MHZ 3 00044 00045 #define RF12_HDR_IDMASK 0x7F 00046 #define RF12_HDR_ACKCTLMASK 0x80 00047 #define RF12_DESTID (rf12_hdr1 & RF12_HDR_IDMASK) 00048 #define RF12_SOURCEID (rf12_hdr2 & RF12_HDR_IDMASK) 00049 00050 // shorthands to simplify sending out the proper ACK when requested 00051 #define RF12_WANTS_ACK ((rf12_hdr2 & RF12_HDR_ACKCTLMASK) && !(rf12_hdr1 & RF12_HDR_ACKCTLMASK)) 00052 00053 /// Shorthand for RF12 group byte in rf12_buf. 00054 #define rf12_grp rf12_buf[0] 00055 /// pointer to 1st header byte in rf12_buf (CTL + DESTINATIONID) 00056 #define rf12_hdr1 rf12_buf[1] 00057 /// pointer to 2nd header byte in rf12_buf (ACK + SOURCEID) 00058 #define rf12_hdr2 rf12_buf[2] 00059 00060 /// Shorthand for RF12 length byte in rf12_buf. 00061 #define rf12_len rf12_buf[3] 00062 /// Shorthand for first RF12 data byte in rf12_buf. 00063 #define rf12_data (rf12_buf + 4) 00064 00065 // RF12 command codes 00066 #define RF_RECEIVER_ON 0x82DD 00067 #define RF_XMITTER_ON 0x823D 00068 #define RF_IDLE_MODE 0x820D 00069 #define RF_SLEEP_MODE 0x8205 00070 #define RF_WAKEUP_MODE 0x8207 00071 #define RF_TXREG_WRITE 0xB800 00072 #define RF_RX_FIFO_READ 0xB000 00073 #define RF_WAKEUP_TIMER 0xE000 00074 00075 //RF12 status bits 00076 #define RF_LBD_BIT 0x0400 00077 #define RF_RSSI_BIT 0x0100 00078 00079 //#define DEBUG 00080 00081 // transceiver states, these determine what to do with each interrupt 00082 enum { 00083 TXCRC1, TXCRC2, TXTAIL, TXDONE, TXIDLE, TXRECV, TXPRE1, TXPRE2, TXPRE3, TXSYN1, TXSYN2, 00084 }; 00085 00086 class RFM12B 00087 { 00088 00089 public: 00090 /* Constructor */ 00091 RFM12B(PinName SDI, PinName SDO, PinName SCK, PinName NCS, PinName NIRQ, PinName NIRQ_LED); 00092 00093 /* Initialises the RFM12B module */ 00094 void Initialize(uint8_t nodeid, uint8_t freqBand, uint8_t groupid=0xAA, uint8_t txPower=0, uint8_t airKbps=0x08); 00095 00096 void ReceiveStart(void); 00097 bool ReceiveComplete(void); 00098 bool CanSend(); 00099 00100 void SendStart(uint8_t toNodeId, bool requestACK = false, bool sendACK = false); 00101 void SendStart(uint8_t toNodeId, const void* sendBuf, uint8_t sendLen, bool requestACK = false, 00102 bool sendACK = false); 00103 void SendACK(const void* sendBuf = "", uint8_t sendLen = 0); 00104 void Send(uint8_t toNodeId, const void* sendBuf, uint8_t sendLen, bool requestACK = false); 00105 00106 void Sleep(int n); 00107 void Sleep(); 00108 void Wakeup(); 00109 bool LowBattery(); 00110 00111 volatile uint8_t * GetData(); 00112 uint8_t GetDataLen(void); // how many bytes were received 00113 uint8_t GetSender(void); 00114 00115 bool ACKRequested(); 00116 bool ACKReceived(uint8_t fromNodeID = 0); 00117 00118 void Encryption(bool encrypt); // does en-/decryption 00119 void SetEncryptionKey(const uint8_t* key); // set encryption key 00120 00121 bool CRC_Pass(void); 00122 00123 protected: 00124 /* SPI module */ 00125 SPI spi; 00126 00127 /* Other digital pins */ 00128 DigitalOut NCS; 00129 InterruptIn NIRQ; 00130 DigitalIn NIRQ_in; 00131 DigitalOut NIRQ_LED; 00132 00133 volatile uint8_t nodeID; // address of this node 00134 volatile uint8_t networkID; // network group 00135 00136 volatile uint8_t* Data; 00137 volatile uint8_t* DataLen; 00138 00139 void InterruptHandler(); // interrupt routine for data reception 00140 00141 int writeCmd(int cmd); // write a command to the RF module 00142 uint16_t crc16_update(uint16_t crc, uint8_t data); 00143 uint16_t xfer(uint16_t cmd); 00144 uint8_t byte(uint8_t out); 00145 00146 private: 00147 volatile uint8_t rf12_buf[RF_MAX]; // recv/xmit buf, including hdr & crc bytes 00148 00149 volatile uint8_t rxfill; // number of data bytes in rf12_buf 00150 volatile int8_t rxstate; // current transceiver state 00151 volatile uint16_t rf12_crc; // running crc value 00152 uint32_t seqNum; // encrypted send sequence number 00153 uint32_t cryptKey[4]; // encryption key to use 00154 long rf12_seq; // seq number of encrypted packet (or -1) 00155 00156 bool useEncryption; 00157 }; 00158 00159 #endif /* _RFM12B_H */
Generated on Sat Jul 23 2022 15:50:27 by
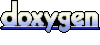