
This program utilizes the mcr20 Thread Shield on the FRDM-K64F MCU which is a two-part workspace (HVAC Server (RX)/Probe(TX)) to handle low temperature events read at the probe(s) to prevent pipes from freezing.
Dependencies: fsl_phy_mcr20a fsl_smac mbed-rtos mbed
Fork of mcr20_wireless_uart by
circular_buffer.cpp
00001 #include "circular_buffer.h" 00002 00003 CircularBuffer::CircularBuffer() 00004 { 00005 size = gCircularBufferSize_c; 00006 readIndex = 0; 00007 writeIndex = 0; 00008 count = 0; 00009 MEM_Init(); 00010 buffer = (uint8_t *) MEM_BufferAlloc(size * sizeof(uint8_t)); 00011 if ( NULL == buffer ) 00012 { 00013 /*if buffer alloc fails stop the program execution*/ 00014 while(1); 00015 } 00016 } 00017 00018 CircularBuffer::CircularBuffer(uint32_t sz) 00019 { 00020 size = sz; 00021 readIndex = 0; 00022 writeIndex = 0; 00023 count = 0; 00024 MEM_Init(); 00025 buffer = (uint8_t *) MEM_BufferAlloc(size * sizeof(uint8_t)); 00026 if ( NULL == buffer ) 00027 { 00028 /*if buffer alloc fails stop the program execution*/ 00029 while(1); 00030 } 00031 } 00032 00033 CircularBuffer::~CircularBuffer() 00034 { 00035 size = 0; 00036 readIndex = 0; 00037 writeIndex = 0; 00038 count = 0; 00039 MEM_BufferFree(buffer); 00040 } 00041 00042 bufferStatus_t CircularBuffer :: addToBuffer (uint8_t c) 00043 { 00044 buffer[writeIndex] = c; 00045 writeIndex++; 00046 if (writeIndex >= size) 00047 { 00048 writeIndex = 0; 00049 } 00050 count++; 00051 if (count >= size) 00052 { 00053 return buffer_Full_c; 00054 } 00055 return buffer_Ok_c; 00056 } 00057 00058 bufferStatus_t CircularBuffer :: getFromBuffer (uint8_t *c) 00059 { 00060 if ( 0 == count ) 00061 { 00062 return buffer_Empty_c; 00063 } 00064 (*c) = buffer[readIndex]; 00065 readIndex++; 00066 if (readIndex >= size) 00067 { 00068 readIndex = 0; 00069 } 00070 count--; 00071 return buffer_Ok_c; 00072 } 00073 00074 uint32_t CircularBuffer :: getCount() 00075 { 00076 return count; 00077 }
Generated on Tue Jul 12 2022 21:51:45 by
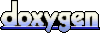